Django - Web Framework
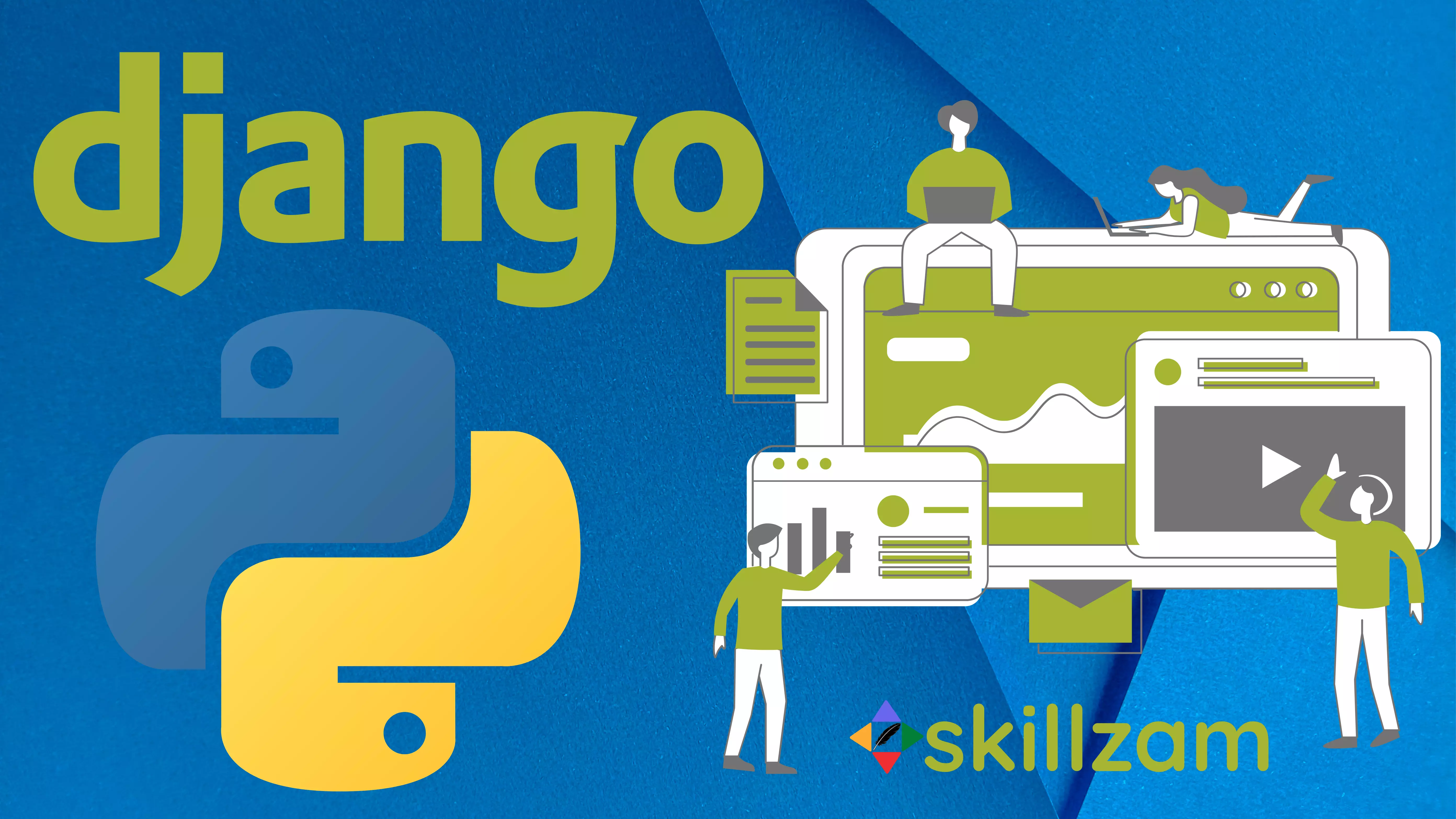
- The Model represents the data and business logic
- The View represents the user interface and interacts with the Model to retrieve or update data
- The Template represents the presentation logic and is responsible for rendering the HTML output.
History of Django:
Django was created in 2003 by Adrian Holovaty and Simon Willison while they were working at the Lawrence Journal-World newspaper in Kansas, USA.
They needed a web framework that would allow them to build web applications quickly and efficiently, and that would handle the repetitive tasks involved in web development, such as database integration and URL routing.
Django was released publicly under a BSD license in July 2005. This is an open source license granting broad permissions to modify and redistribute Django.
The framework was named after guitarist Django Reinhardt. Adrian Holovaty is a Romani jazz guitar player and a big fan of Django Reinhardt.
In 2008, the Django Software Foundation (DSF) was created to manage the development and promotion of the framework,
Today, Django is one of the most popular & widely used web frameworks in the Python ecosystem (as per Github stars), powering thousands of websites and web applications around the world.
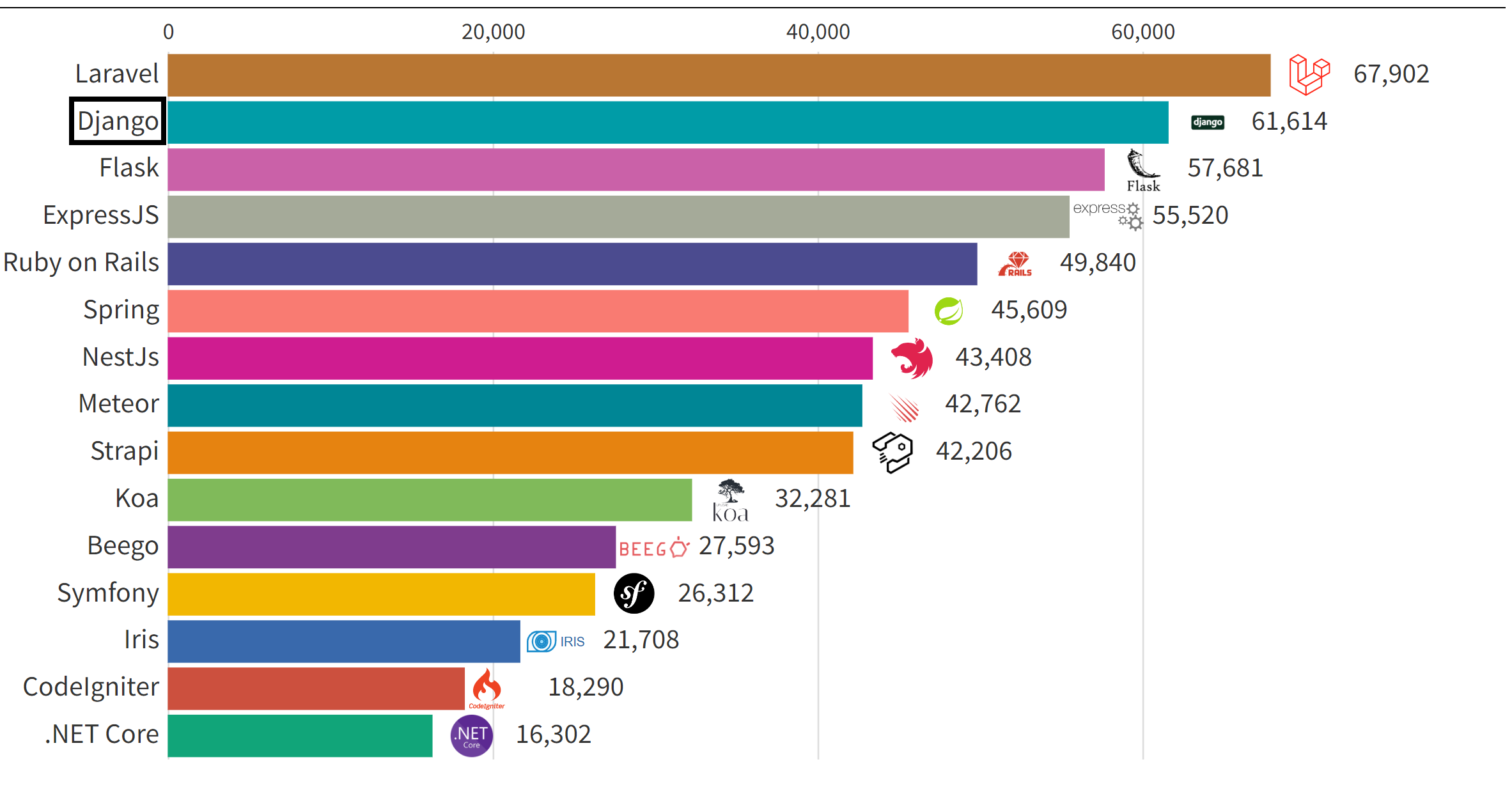
Django - Architectural pattern
Django follows the Model-View-Template (MVT) architectural pattern, which is a variant of the traditional Model-View-Controller (MVC) pattern. In MVT, the application is divided into three main components:
( In other words, the Model is defined by Python classes that define the database schema and are responsible for data storage and retrieval. The View is implemented by Python functions or classes that handle HTTP requests, perform any necessary data processing, and return HTTP responses. Finally, the Template is implemented using HTML and Django's template language and is responsible for rendering the final output that the user sees in their web browser. )
The main difference between the MVT and MVC patterns is that in MVT, the Template layer is explicitly separated from the View layer, while in MVC, the View layer is responsible for both handling HTTP requests and generating HTML output.
Django's MVT pattern provides a clear separation of concerns between the different components of the application, which makes it easier to develop, test, and maintain complex web applications.
Offical Django Documentation: Django Home
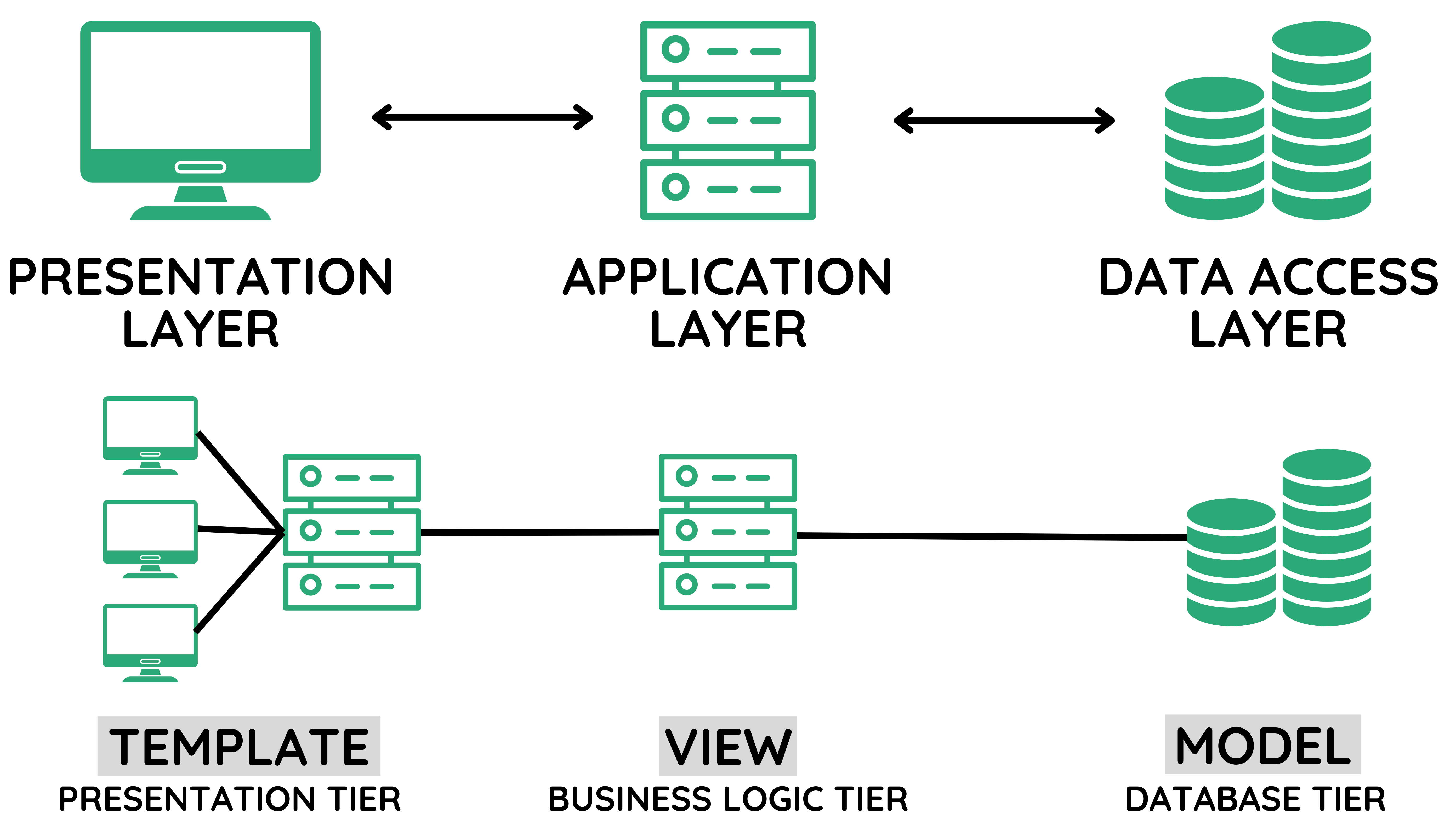
Django - Dynamic Admin interface
Django includes a built-in administrative interface that allows developers to manage the application's data and settings through a web-based interface, without having to write any code.
Django admin interface is automatically generated based on the application's models, and provides a powerful and flexible way to manage the application's data, users, permissions, and other settings. The admin interface includes features such as:
To use the Django admin interface, developers need to register their models with the admin site by creating a subclass of the admin.ModelAdmin
class and registering it using the admin.site.register()
method. This allows Django to generate the necessary views and forms to manage the data associated with the model.
Django admin interface is a powerful tool that can save developers a lot of time and effort when managing their application's data and settings, and is one of the key features that has contributed to Django's popularity and success.
How does Django framework works ?
Django is installed and web application is accessed using the URL browser requests, this is basically what happens
urls.py
file, and calls the view that matches the URL.views.py
, checks for relevant models. models.py
file. template
folder. 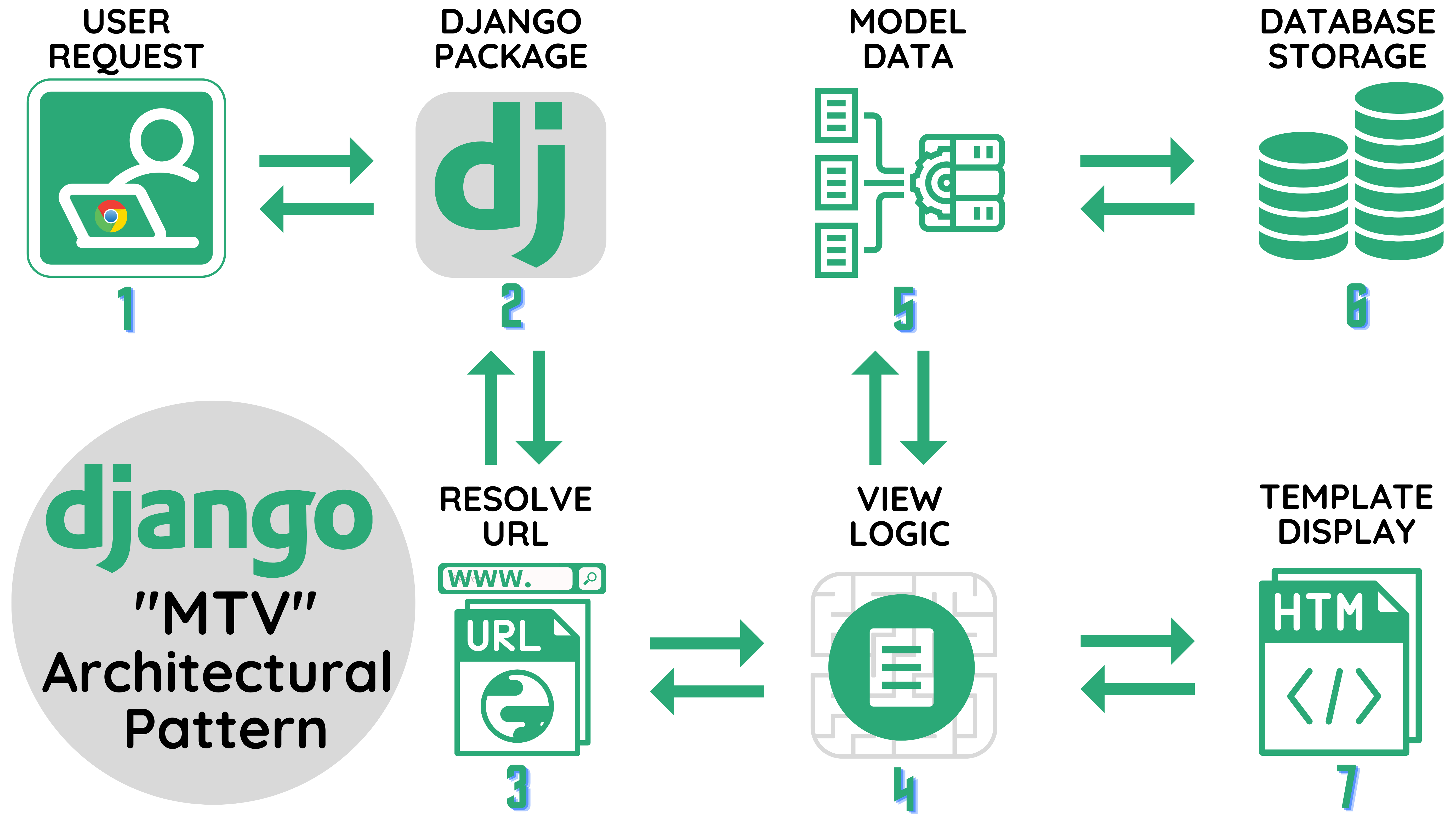
Django Install & working
Before we use Django, we need to get it installed. Being a Python web framework, Django requires Python.
Python includes a lightweight database called SQLite
so you won't need to set up a database just yet.
What Python version can I use with Django?
Django version | Python versions |
---|---|
2.2 | 3.5, 3.6, 3.7, 3.8 (added in 2.2.8), 3.9 (added in 2.2.17) |
3.1 | 3.6, 3.7, 3.8, 3.9 (added in 3.1.3) |
3.2 | 3.6, 3.7, 3.8, 3.9, 3.10 (added in 3.2.9) |
4.0 | 3.8, 3.9, 3.10 |
4.1 | 3.8, 3.9, 3.10, 3.11 (added in 4.1.3) |
1. Check whether Python is installed
You can verify that Python is installed by checking python version from command prompt or shell; you should see something like:
C:\Users\Skillzam> python --version
Python 3.11.1
If Python is NOT installed then, get the latest version of Python at https://www.python.org/downloads/ or with your operating system’s package manager.
2. Check whether package manager like PIP is installed
To install Django, you must use a package manager like PIP, which is included in Python from version 3.4.
To check if your system has PIP installed, run this command in the command prompt:
C:\Users\Skillzam> pip --version
pip 22.3.1 from D:\install\python\Lib\site-packages\pip (python 3.11)
If PIP is NOT installed, then download and install it from this page: https://pypi.org/project/pip/
3. Create Virtual Environment
Python applications will often use packages and modules that don't come as part of the standard library. Applications will sometimes need a specific version of a library, because the application may require that a particular bug has been fixed or the application may be written using an obsolete version of the library's interface.
This means it may not be possible for one Python installation to meet the requirements of every application. If application A needs version 1.0 of a particular module but application B needs version 2.0, then the requirements are in conflict and installing either version 1.0 or 2.0 will leave one application unable to run.
The solution for this problem is to create a virtual environment, a self-contained directory tree that contains a Python installation for a particular version of Python, plus a number of additional packages.
To create a virtual environment, decide upon a directory where you want to place it, and run the venv
module as a script with the directory path.
Create a new folder "django" and navigate to that folder location.
C:\Users\Skillzam> cd Desktop
C:\Users\Skillzam\Desktop> cd code
C:\Users\Skillzam\Desktop\code> mkdir django
C:\Users\Skillzam\Desktop\code> cd django
C:\Users\Skillzam\Desktop\code\django> py -m venv myDjangoEnv
This will set up a virtual environment, and create a folder named "myDjangoEnv" with subfolders and files, like this:
myDjangoEnv
Include
Lib
pyvenv.cfg
Scripts
4. Activate the Virtual Environment
We can activate the Virtual environment, by typing the below command:
NOTE: You must activate the virtual environment every time you open the command prompt to work on your project.
C:\Users\Skillzam\Desktop\code\django> myDjangoEnv\Scripts\activate.bat
We can also deactivate the Virtual environment, by typing the below command:
C:\Users\Skillzam\Desktop\code\django> myDjangoEnv\Scripts\deactivate.bat
5. Django installation
We need to be in virtual environment, in order to install Django.
Django is installed using pip
, with the below command:
(myDjangoEnv) C:\Users\Skillzam\Desktop\code\django> py -m pip install Django
Collecting Django
Downloading Django-4.1.7-py3-none-any.whl (8.1 MB)
---------------------------------------- 8.1/8.1 MB 1.7 MB/s eta 0:00:00
Collecting asgiref<4,>=3.5.2
Downloading asgiref-3.6.0-py3-none-any.whl (23 kB)
Collecting sqlparse>=0.2.2
Downloading sqlparse-0.4.3-py3-none-any.whl (42 kB)
---------------------------------------- 42.8/42.8 kB 707.1 kB/s eta 0:00:00
Collecting tzdata
Downloading tzdata-2022.7-py2.py3-none-any.whl (340 kB)
---------------------------------------- 340.1/340.1 kB 2.6 MB/s eta 0:00:00
Installing collected packages: tzdata, sqlparse, asgiref, Django
Successfully installed Django-4.1.7 asgiref-3.6.0 sqlparse-0.4.3 tzdata-2022.7
[notice] A new release of pip available: 22.3.1 -> 23.0.1
[notice] To update, run: python.exe -m pip install --upgrade pip
6. Check Django installed version
If Django is installed, you should see the version of your installation. If it isn't, you'll get an error telling “No module named django”
.
We can check the installed Django version number like this:
(myDjangoEnv) C:\Users\Skillzam\Desktop\code\django> django-admin --version
4.1.7
To verify that Django can be seen by Python, type py
from your command prompt. Then at the Python prompt, try to import django
(myDjangoEnv) C:\Users\Skillzam\Desktop\code\django> py
Python 3.11.1 (tags/v3.11.1:a7a450f, Dec 6 2022, 19:58:39) [MSC v.1934 64 bit (AMD64)] on win32
Type "help", "copyright", "credits" or "license" for more information.
>>> import django
>>> print(django.get_version())
4.1.7
>>>
7. Create Django Project
Navigate to where in the file system we want to store the code (in the virtual environment), and run this command in the command prompt:
(myDjangoEnv) C:\Users\Skillzam\Desktop\code\django\myDjangoEnv> django-admin startproject mysite
Django creates a mysite
folder on the computer, with this content:
mysite/
manage.py
mysite/
__init__.py
asgi.py
settings.py
urls.py
wsgi.py
These files are:
mysite
/ root directory is just a container for your project. Its name doesn't matter to Django; you can rename it to anything you like.manage.py
: A command-line utility that lets you interact with this Django project in various ways.mysite
/ directory is the actual Python package for your project. Its name is the Python package name you'll need to use to import anything inside it (e.g. mysite.urls
).mysite/__init__.py
: An empty file that tells Python that this directory should be considered a Python package.mysite/asgi.py
: To apply ASGI middleware, or to embed Django in another ASGI application, you can wrap Django's application object in this file.mysite/settings.py
: Settings/configuration for this Django project.mysite/urls.py
: The URL declarations for this Django project; a “table of contents” of your Djangopowered site.mysite/wsgi.py
: An entry-point for WSGI-compatible web servers to serve your project.8. Run Django Project
To run the Django project, we need to navigate to the mysite
folder and execute the below command in the command prompt:
(myDjangoEnv) C:\Users\Skillzam\Desktop\code\django\myDjangoEnv\mysite> py manage.py runserver
Watching for file changes with StatReloader
Performing system checks...
System check identified no issues (0 silenced).
You have 18 unapplied migration(s). Your project may not work properly until you apply the migrations for app(s): admin, auth, contenttypes, sessions.
Run 'python manage.py migrate' to apply them.
March 13, 2023 - 01:50:05
Django version 4.1.7, using settings 'mysite.settings'
Starting development server at http://127.0.0.1:8000/
Quit the server with CTRL-BREAK.
Now open the browser - Google Chrome and type http://127.0.0.1:8000/
in the address bar to see the below result.
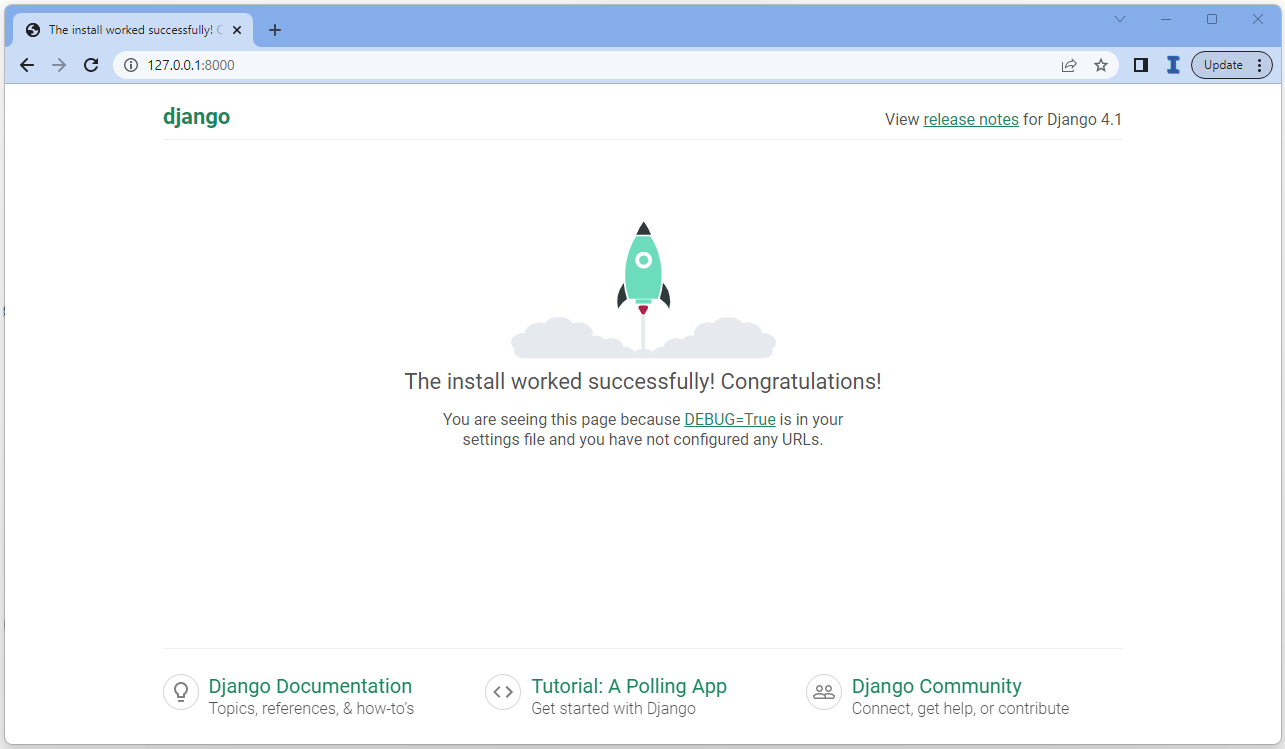
We have just started the Django development server, a lightweightWeb server written purely in Python.
Django development server is included, so that we can develop things rapidly, without having to deal with configuring a production server - such as Apache
- until you're ready for production.
NOTE: Don't use Django development server in anything resembling a production environment. It's intended only for use while developing.
The development server automatically reloads Python code for each request as needed. You don't need to restart the server for code changes to take effect. However, some actions like adding files don't trigger a restart, so you'll have to restart the server in these cases.
Remember that Django is in the business of making Web frameworks, not Web servers.
Changing the port :
By default, the runserver
command starts the development server on the internal IP at port 8000
.
If you want to change the server's port, pass it as a command-line argument. For instance, this command starts the server on port 8080:
(myDjangoEnv) C:\Users\Skillzam\Desktop\code\django\myDjangoEnv\mysite> py manage.py runserver 8080
If you want to change the server's IP, pass it along with the port. So to listen on all public IPs (useful if you want to show off your work on other computers on your network), use:
(myDjangoEnv) C:\Users\Skillzam\Desktop\code\django\myDjangoEnv\mysite> py manage.py runserver 0.0.0.0:8000
Hello World - using Django framework
1. Create an App
Once the Django project is setup, we need to create an application or an App.
PATH
)Projects vs. Apps
app
is a Web application that does something - e.g., a Weblog system, a database of public records or a simple poll app.project
is a collection of configuration and apps for a particular website. Navigate to the selected location where we want to store the app, in our case the firstApp
folder, and run the below command :
(myDjangoEnv) C:\Users\Skillzam\Desktop\code\django\myDjangoEnv\mysite> py manage.py startapp firstApp
That'll create a directory firstApp
, which is laid out like this:
firstApp/
migrations/
__init__.py
__init__.py
admin.py
apps.py
models.py
tests.py
views.py
2. Create first view
Django views are Python functions that takes http requests and returns http response, like HTML documents. Views are usually put in a file called views.py located on your app's folder.
Open the file mysite/firstApp/views.py
and put the following Python code in it:
from django.shortcuts import render
from django.http import HttpResponse
# Create your views here.
def index(request):
return HttpResponse("Hello World!")
3. Create file urls.py
To call the view, we need to map it to a URL - and for this we need a URLconf
. To create a URLconf in the mysite/firstApp/
directory, create a file called urls.py
Create a file named urls.py
in the same folder as the views.py
file, and type this code in it:
from django.urls import path
from . import views
urlpatterns = [
path('index/', views.index, name='index'),
]
4. Point the root URLconf
The next step is to point the root URLconf at the firstApp.urls
module.
There is a file called urls.py
on the mysite
folder, open that file and add the include
module in the import
statement, and also add a path()
function in the urlpatterns[]
list, with arguments that will route users that comes in via 127.0.0.1:8000/
from django.contrib import admin
from django.urls import include, path
urlpatterns = [
path('', include('firstApp.urls')),
path('admin/', admin.site.urls),
]
5. Run Django Project containing the App
To run the Django project, we need to navigate to the mysite
folder and execute the below command in the command prompt:
(myDjangoEnv) C:\Users\Skillzam\Desktop\code\django\myDjangoEnv\mysite> py manage.py runserver
Watching for file changes with StatReloader
Performing system checks...
System check identified no issues (0 silenced).
You have 18 unapplied migration(s). Your project may not work properly until you apply the migrations for app(s): admin, auth, contenttypes, sessions.
Run 'python manage.py migrate' to apply them.
March 13, 2023 - 12:35:52
Django version 4.1.7, using settings 'mysite.settings'
Starting development server at http://127.0.0.1:8000/
Quit the server with CTRL-BREAK.
Now open the browser - Google Chrome and type http://127.0.0.1:8000/index
in the address bar to see the below result.
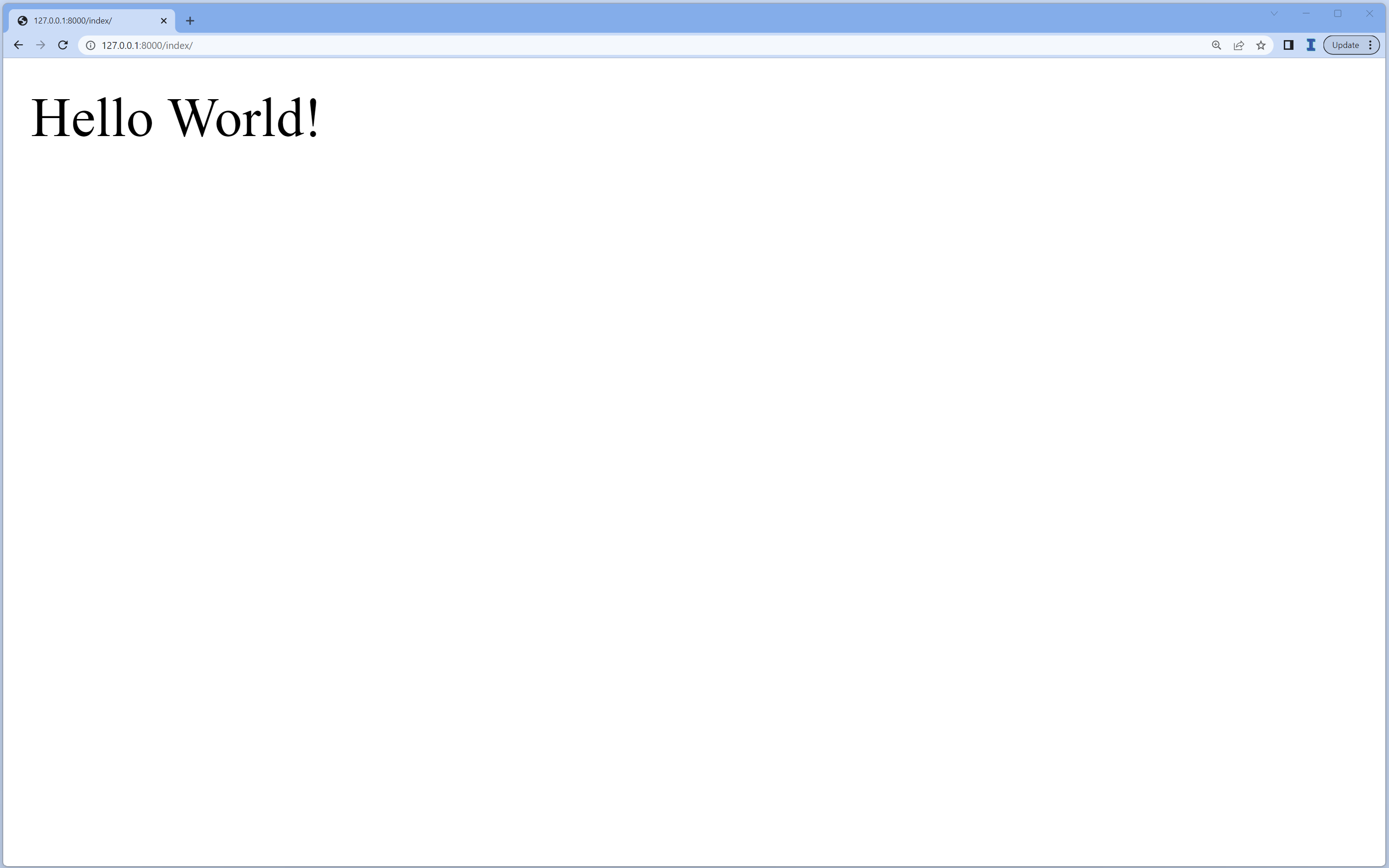
Django Interview Questions
Get the hold of actual interview questions during job hiring.
What is Django, and what are its key features?
Django is a high-level Python web framework that follows the Model-Template-View (MTV) architectural pattern. Its key features include a powerful Object-Relational Mapping (ORM) system, a built-in template engine, an automatic admin interface, and support for URL routing and middleware.
What are the advantages of using Django?
Some advantages of using Django are:
What is a virtual environment in Django?
A virtual environment is a self-contained directory that contains a specific version of Python and its dependencies. It allows you to install packages without affecting the global Python installation. In Django, virtual environments are often used to manage project-specific dependencies.
What is an ORM in Django?
An ORM (Object-Relational Mapping) is a technique that allows you to interact with a database using objects instead of SQL queries. Django provides an ORM that makes it easy to interact with databases and write database queries using Python code.
What is the difference between a Django project and a Django app?
A Django project is the entire web application, including all the configuration files, settings, and top-level URLs. A Django app is a self-contained module that provides a specific functionality within a project and can be reused across projects. An app typically contains models, views, templates, and static files for a specific functionality within the web application. A project can contain multiple apps, and an app can be used in multiple projects.
How does Django handle database migrations?
Django uses a built-in ORM system that maps Python objects to database tables. When a model is changed, Django creates a migration file that contains the necessary changes to the database schema. These migration files can be applied to the database using the "migrate" command.
What is the Django template language, and how is it used?
The Django template language is a syntax used to create HTML templates that can be dynamically rendered with data from a Django view. It includes various tags and filters that allow for logic and iteration within templates, and it also supports template inheritance to create reusable templates.
How does Django handle user authentication and authorization?
Django provides a built-in authentication system that includes user models, views, and forms. It also supports permissions and groups to manage access control within an application. Developers can customize the authentication and authorization system to fit their specific needs.
What is the purpose of middleware in Django, and how is it used?
Middleware in Django is a way to add functionality to the request/response process before or after it reaches the view. Middleware can be used for authentication, caching, logging, and other purposes. Developers can create their own middleware classes and add them to the middleware stack in the project settings.
How does Django handle URL routing?
Django uses a built-in URL routing system that maps URLs to views. URLs are defined in the project's URL configuration file, and each URL pattern is associated with a view function or class. The URL routing system supports regular expressions and named URL parameters.
How does Django support internationalization (i18n) and localization (l10n)?
Django includes built-in support for i18n and l10n. It provides tools for translating text in templates, forms, and models. Developers can create translation files for different languages and regions, and Django will automatically detect the user's language preference and serve the appropriate translation.
How does Django handle static files?
Django includes a built-in static file handler that can serve CSS, JavaScript, and image files. Developers can define the location of their static files in the project settings, and Django will automatically collect and serve them using the "collectstatic" command.
What are some best practices for developing Django applications?
Some best practices for developing Django applications include following the DRY (Don't Repeat Yourself) principle, writing reusable code, testing thoroughly, using version control, and optimizing database queries. It's also important to follow the Django coding style and conventions to maintain consistency and readability in the codebase.
What are signals in Django, and how are they used?
Signals in Django are a way for certain parts of the application to be notified when certain events occur. They allow decoupling of components and can be used for a variety of purposes, such as triggering updates to caches or sending notifications. Signals are defined as functions or methods decorated with a signal decorator, and they are connected to sender objects using a connect function.
What is the Django REST framework, and how is it used?
The Django REST framework is a third-party package that provides tools for building RESTful APIs using Django. It includes serializers, views, and authentication and permission classes, among other features. Developers can use the Django REST framework to build APIs that interact with their Django models and provide data to clients in a variety of formats, such as JSON or XML.
What are class-based views in Django, and how are they used?
Class-based views in Django are a way to define view functions as classes, rather than as functions. They provide a structured way to handle requests and responses and can be extended and customized easily. Class-based views are defined by subclassing one of Django's built-in view classes and overriding certain methods, such as "get" or "post".
How does Django handle form validation?
Django provides a built-in forms library that allows developers to create and validate forms easily. Form validation is done automatically when the form is submitted, and any errors are displayed to the user. Validation can be customized using validators and custom form fields.
How does Django handle caching?
Django provides a caching framework that allows developers to store frequently accessed data in memory or on disk, improving performance. The caching framework includes support for different backends, such as Memcached or Redis, and provides tools for controlling cache keys and timeouts.
How does Django handle security, such as preventing cross-site scripting (XSS) attacks?
Django provides various security measures, such as automatic CSRF (Cross-Site Request Forgery) protection and built-in support for secure cookies and HTTPS. Django's template engine also includes automatic escaping of HTML characters to prevent XSS attacks.
How does Django handle testing?
Django provides a built-in testing framework that allows developers to write unit tests and integration tests for their applications. The testing framework includes support for various types of tests, such as TestCase or LiveServerTestCase, and provides tools for mocking and patching dependencies.
What is Django's approach to handling errors and exceptions?
Django provides a robust error handling system that includes middleware for catching and reporting errors, as well as a debug toolbar for developers to investigate errors in development environments. Django also includes a logging framework for tracking errors and exceptions in production environments.
How does Django handle security updates and vulnerabilities?
Django has a strong security team that responds to reported vulnerabilities promptly and releases security updates as needed. Developers are encouraged to keep their Django installations up-to-date and to follow best practices for secure development, such as avoiding hardcoded passwords and using HTTPS.
What is Django ORM, and how does it work?
Django ORM (Object-Relational Mapping) is a powerful tool that allows developers to interact with the database using Python code instead of SQL. It provides an abstraction layer over the database, allowing developers to work with database tables as Python classes and database rows as instances of those classes. Developers can define models using Python classes and attributes, and Django ORM will automatically generate the necessary SQL statements to interact with the database.
What is Django's admin interface, and how is it used?
Django's admin interface is a built-in feature that provides a graphical user interface for managing data in the database. It allows authorized users to view, create, update, and delete records in the database using a web interface. The admin interface is highly customizable and can be extended to provide additional functionality.
What are Django templates, and how are they used?
Django templates are files that define the structure and layout of web pages. They allow developers to separate the presentation logic from the application logic, making it easier to maintain and update the application. Django templates use a syntax similar to HTML and can include dynamic content using template tags and filters.
What is Django's session framework, and how is it used?
Django's session framework allows developers to store user-specific data across requests. It uses cookies or other storage mechanisms to store a unique session ID on the user's browser and uses that ID to retrieve the user's session data on subsequent requests. Developers can use the session framework to store data such as the user's login status, shopping cart contents, or preferences.
What is the Django REST framework's ModelViewSet, and how is it used?
The Django REST framework's ModelViewSet is a class that provides a set of standard CRUD (Create, Read, Update, Delete) actions for a Django model. It handles common operations such as listing, creating, updating, and deleting model instances and provides a customizable interface for handling these actions.