JavaScript Language
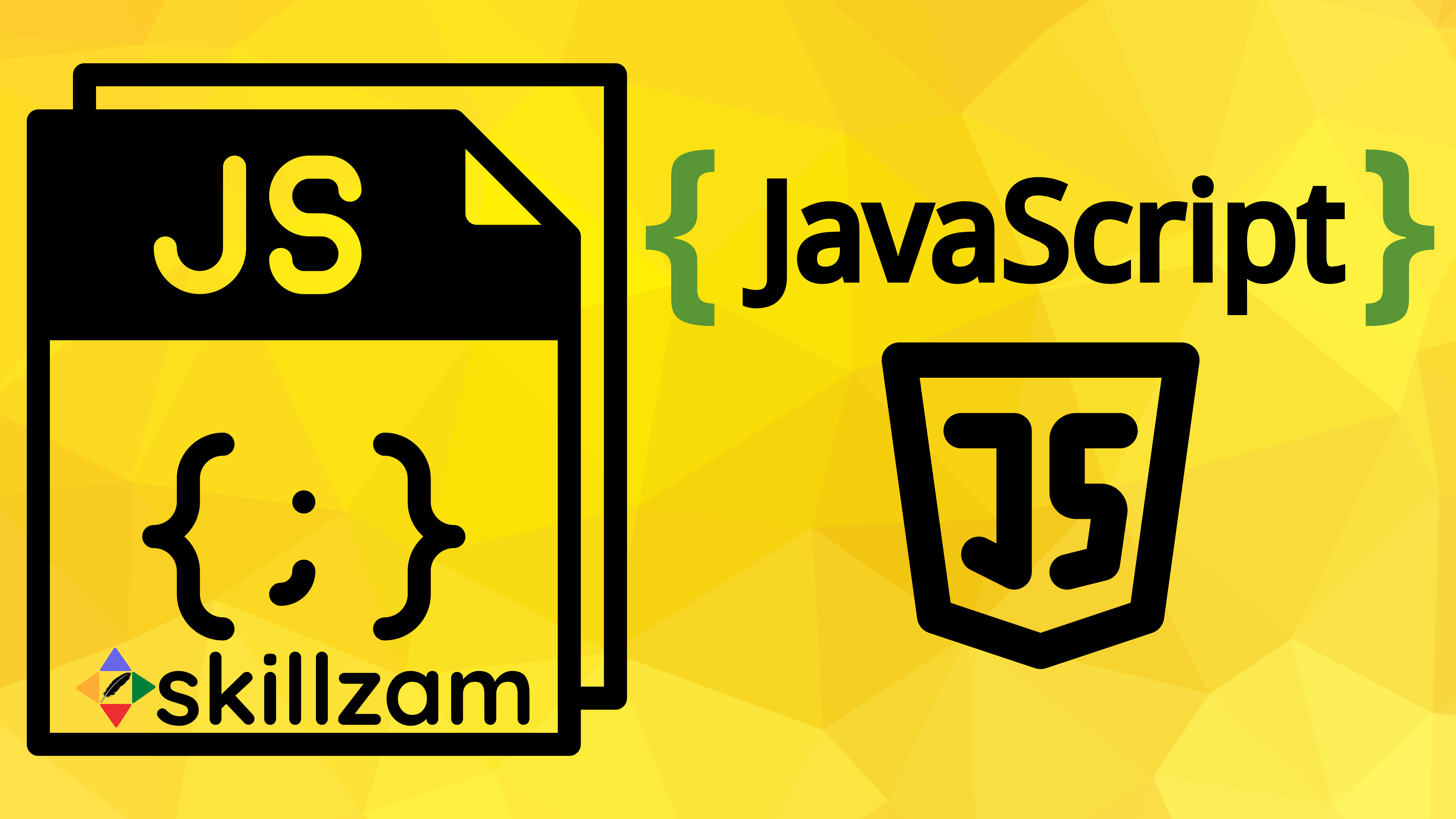
HTML
& CSS
.- (a) Client-side JavaScript extends the core language by supplying objects to control a browser & its Document Object Model (DOM). For example, client-side extensions allow an application to place elements on an HTML form and respond to user events such as mouse clicks, form input, and page navigation.
- (b) Server-side JavaScript extends the core language by supplying objects relevant to running JavaScript on a server. For example, server-side extensions allow an application to communicate with a database, provide continuity of information from one invocation to another of the application, or perform file manipulations on a server.
What is JavaScript ?
JavaScript (JS) is a lightweight
, interpreted
, just-in-time compiled
, high-level
, prototype-based
, dynamic typed
, object-oriented
programming language, with first-class functions.
TERMINOLOGIES EXPLAINED
- (a) A programming language is any set of rules that converts strings, or graphical program elements in the case of visual programming languages, to various kinds of machine code output.
- (b) Programming languages are one kind of computer language, and are used in computer programming to implement algorithms.
- (a) These are designed to have small memory footprint, are easy to implement (important when porting a language to different computer systems)
- (b) They have simple syntax and semantics, so one can learn them quickly and easily.
- (c) Some lightweight languages : for Example - JavaScript, BASIC, Lisp, Forth, and Tcl
- (a) It is a programming language which are generally interpreted, without compiling a program into machine instructions.
- (b) It is one where the instructions are not directly executed by the target machine, but instead read and executed by some other program.
- (c) Interpreted language examples - JavaScript, Perl, Python, BASIC, etc.
- (a) just-in-time (JIT) compilation (also dynamic translation or run-time compilations) is a way of executing computer code that involves compilation during execution of a program (at run time) rather than before execution.
- (b) This may consist of source code translation but is more commonly bytecode translation to machine code, which is then executed directly.
- (a) It refers to the higher level of abstraction from machine language.
- (b) Rather than dealing with registers, memory addresses, and call stacks, high-level languages deal with variables, arrays and functions, loops and other abstract computer science concepts, with a focus on usability over optimal program efficiency.
- (c) Examples: Python, JavaScript, PHP, Java, C#, Perl etc
- (a) Are those where the interpreter assigns variables a type at runtime based on the variable's value at the time.
- (b) Most dynamic languages are also dynamically typed, but not all are. Dynamic languages are frequently referred to as scripting languages.
- (c) Popular dynamic programming languages include JavaScript, Python, Ruby, PHP, Lua and Perl.
- (a) OOP (Object-Oriented Programming) is an approach in programming in which data is encapsulated within objects and the object itself is operated on, rather than its component parts.
- (b) JavaScript is heavily object-oriented. It follows a prototype-based model, but it also offers a class syntax to enable typical OOP paradigms.
- (a) Prototype-based programming is a style of object-oriented programming in which classes are not explicitly defined, but rather derived by adding properties and methods to an instance of another class or, less frequently, adding them to an empty object.
- (b) In simple words: this type of style allows the creation of an object without first defining its class.
- (a) A programming language is said to have First-class functions when functions in that language are treated like any other variable.
- (b) For example, in such a language, a function can be passed as an argument to other functions, can be returned by another function and can be assigned as a value to a variable.
Things that JavaScript can do:
- (a) Show or hide more information by click of a button
- (b) Change color of a button when mouse hovers
- (c) Slide through a carousel of images on homepage
- (d) Zooming in or zooming out on an image
- (e) Displaying a timer or count-down on a website
- (f) Playing audio & video in a web page
- (g) Displaying animations & Using a drop-down hamburger menu
- (a) Developers can use various JavaScript frameworks and libraries
- (b) JavaScript front-end frameworks include
React
,React Native
,Angular
, andVue
- (c) A few famous examples include Paypal, LinkedIn, Netflix, Uber etc. use JavaScript.
- (a) build simple web servers
- (b) develop the back-end infrastructure using
Node.js
- (a) JavaScript library,
RevealJS
is used to build a web-based slide deck - (b) Examples like slides.com
- (a) quadcopters come with a simple OS that makes it possible to install
NodeJS
. - (b) Which means, you can program a drone
History of JavaScript:
Brendan Eich created the JavaScript in 1995. At that time, web pages could only be static, lacking the capability for dynamic behavior after the page was loaded in the browser.
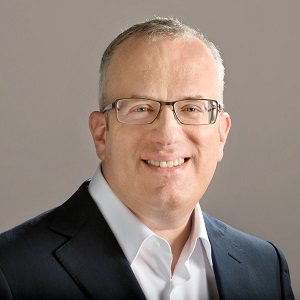
JavaScript was called LiveScript earlier at Netscape corporation. It became very popular during the dot-com boom.
ECMAScript standard
ECMA-262.
JavaScript Engines
JavaScript engine is a software component that executes JavaScript code and converts it into computer understandable language. All relevant modern engines use just-in-time compilation for improved performance. JavaScript engines are typically developed by web browser vendors, and every major browser has one.
JavaScript Analogy : English Grammar
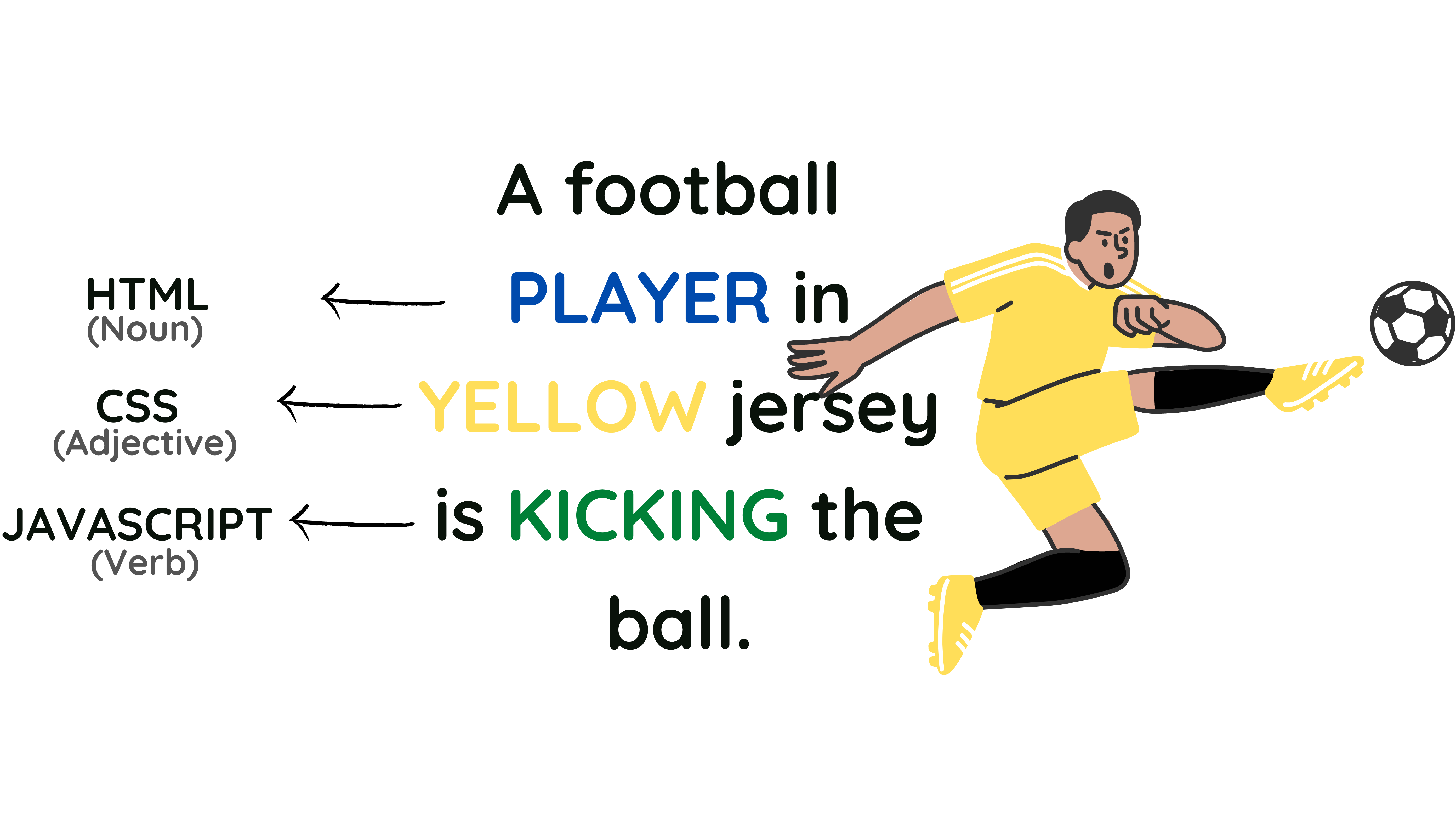
JavaScript: Install & Run
Modern Web browser
Getting started with JavaScript is easy: all you need is a modern Web browser.
Install the either one of the prefered browsers like Google Chrome or Firefox Browser
Web Console
The Web Console tool built into browers like Google Chrome / Firefox is useful for experimenting with JavaScript; you can use it in two modes: single-line input mode, and multi-line input mode.
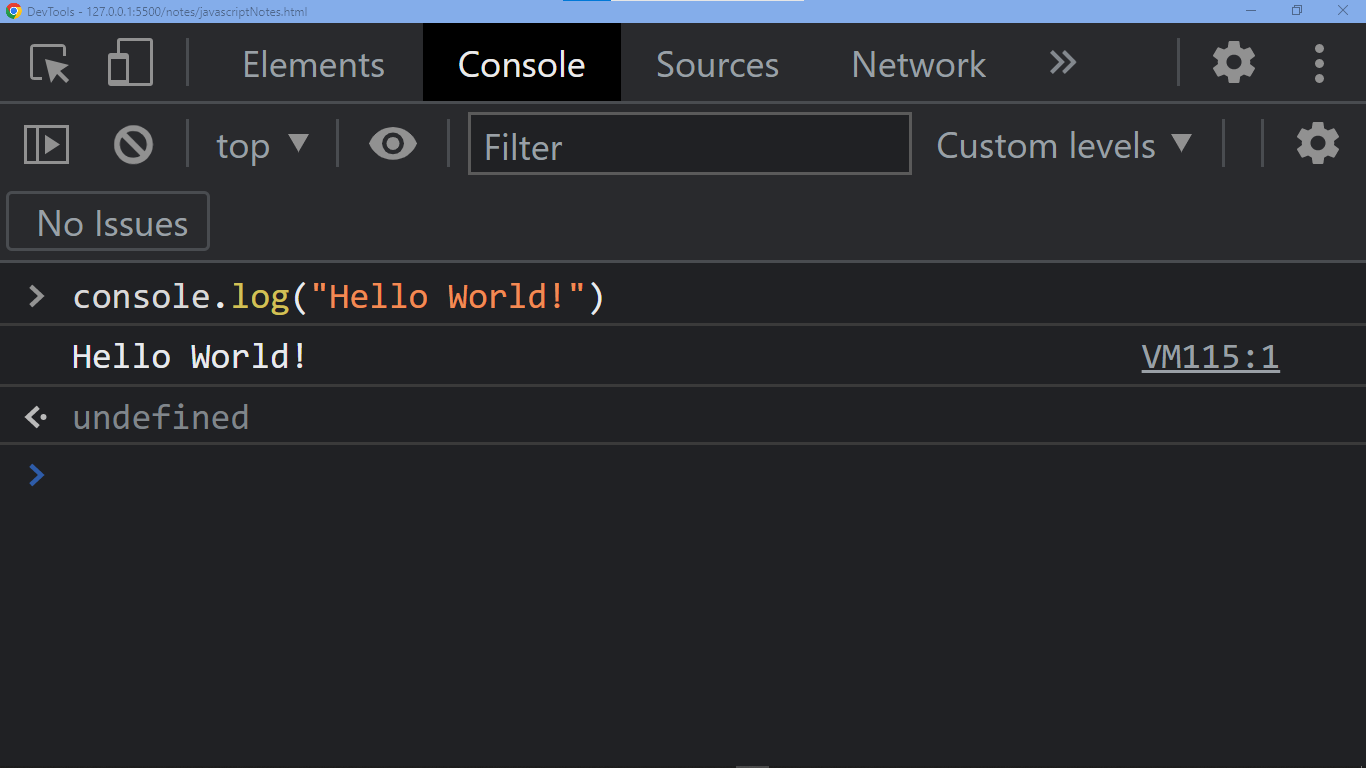
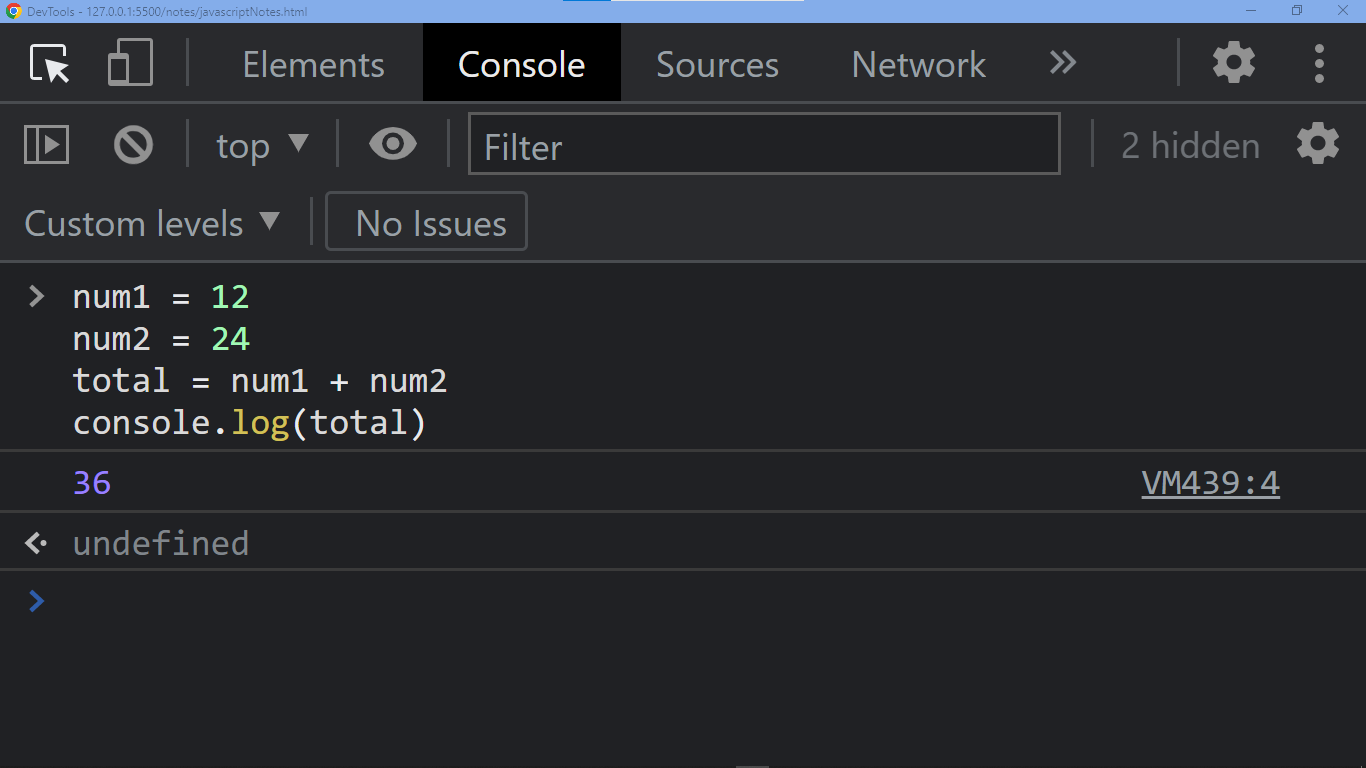
JavaScript language syntax
Syntax refers to the structure of the language, which is, what constitutes a correctly-formed program.
Observe the below code:
// Program to add two numbers
// function declaration for adding two numbers
function addNum(num1, num2) {
let total = 0
total = num1 + num2
return (total)
}
let value1 = 0; value2 = 0
value1 = parseInt(prompt('Enter the first number: ')) // Input first number
value2 = parseInt(prompt('Enter the second number: ')) // Input second number
/*
Invoking the function addNum()
and Printing the Output
*/
console.log(`The sum of ${value1} and ${value2} is ${addNum(value1, value2)}`)
OUTPUT
Enter the first number: 24 Enter the second number: 12 The sum of 24 and 12 is 36
This above script illustrates several of the important aspects of JavaScript syntax.
Let's walk through it and discuss some of the syntactical features of JavaScript.
Comments are marked by //
or /* */
// Program to add two numbers
//
and anything on the line following the sign //
is ignored by the interpreter.
value1 = parseInt(prompt('Enter the first number: ')) // Input first number
/* */
syntax.
/*
Invoking the function addNum()
and Printing the Output
*/
End-of-Line terminates a statement
total = num1 + num2
total
is assigned to sum of two numbers num1 + num2
;
Semicolon can optionally terminate a statement
Sometimes it can be useful to put multiple statements on a single line. This shows in the example, how the semicolon ;
familiar in C or Python Language, can be used optionally in JavaScript to put two statements on a single line.
In JavaScript, instructions are called statements and are separated by semicolons ;
. A semicolon is not necessary after a statement if it is written on its own line. But if more than one statement on a line is desired, then they must be separated by semicolons.
let value1 = 0; value2 = 0;
Functionally, this is entirely equivalent to writing
let value1 = 0
let value2 = 0
Block scope represented by { }
In addition, variables declared with let
or const
can belong to Block scope. This scope created with a pair of curly braces { }
(a block).
In the function declaration, the block of code looks:
In programming languages, a block of code is a set of statements that should be treated as a unit.
// function declaration for adding two numbers
function addNum(num1, num2) {
let total = 0
total = num1 + num2
return (total)
}
Whitespaces within lines does not matter
White space within lines of JavaScript code does not matter
For example, all three of these expressions are equivalent:
sumNumbers=12+34
sumNumbers = 12 + 34
sumNumbers = 12 + 34
Abusing this flexibility can lead to issues with code readibility.
Using whitespace effectively can lead to much more readable code, especially in cases where operators follow each other - compare the following two expressions for exponentiating by a negative number:
Observe that second version with spaces much is more easily readable at a single glance.
squareNumber=9**2
// Check the below with SPACES
squareNumber = 9 ** 2
Parentheses are for grouping or calling
Two major uses of parentheses. First, they can be used in the typical way to group statements or mathematical operations:
12 * (34 + 56)
# 1080
Secondly, they can also be used to indicate that a function is being invoked (called).
In the below snippet, the addNum()
is used to call the function with two arguments
. The function call is indicated by a pair of opening and closing parentheses, with the arguments to the function contained within:
addNum(value1, value2)
Some functions can be called with no arguments at all, in which case the opening and closing parentheses still must be used to indicate a function evaluation. An example of this is the toUpperCase()
method of string datatype.
The "()" after toUpperCase indicates that the function should be executed, and is required even if no arguments are necessary.
let firstString = 'Skillzam'.toUpperCase()
console.log(firstString)
// SKILLZAM
Add JavaScript to HTML
JavaScript is one of the top three core technologies of world wide web (www). The other two are HTML
& CSS
.
JavaScript can be added to HTML file in 3 ways:
Inline JavaScript
onmouseover
, onclick
, onchange
etc.<script>
tag in the HTML file.For Example: The below HTML file contains a <button>
tag, which has inline JavaScript handler -
onclick="window.alert('You clicked the button!')"
<html lang="en-US">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width">
<title>Add inline JavaScript to HTML</title>
</head>
<body>
<!-- Inline JavaScript handlers - onclick="window.alert('You clicked the button!')" -->
<button onclick="window.alert('You clicked the button!')">Click Me</button>
</body>
</html>
OUTPUTInternal JavaScript
<script>...</script>
tag of HTML, that wrap around JavaScript code inside the HTML program.<script>...</script>
tag with JavaScript code in the <body>
tag or <head>
tag, because it is completely depends on the structure of the web page being built.For Exmaple: In the below HTML code, the <script>...</script>
tag inside the <body>
section, contains the JavaScript code to write a text (string) on the webpage:
<html lang="en-US">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width">
<title>Add internal JavaScript to HTML</title>
</head>
<body>
<!-- JavaScript code begins here -->
<script>
document.write("Example to demo the Internal JavaScript")
</script>
<!-- JavaScript code ends here -->
</body>
</html>
OUTPUT 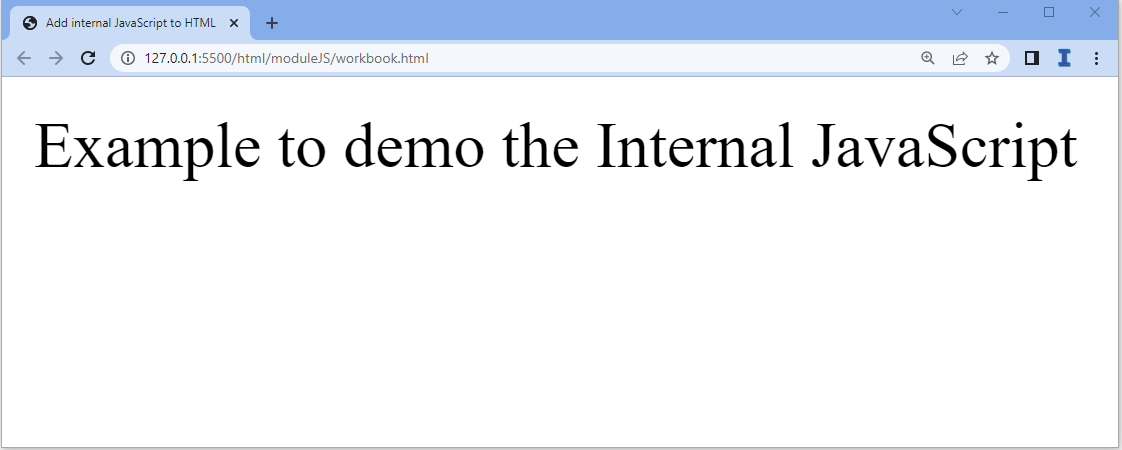
External JavaScript
.js
extension will be included in the HTML file using the src
(source) attribute of the <script>
tag.<head>
or <body>
.<script>
tag is located.<script>
tags.For Example: the below mentioned HTML
file contains External JavaScript file "app.js", which is added using <script>
tag with src
attribute.
<html lang="en-US">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width">
<title>Add external JavaScript to HTML</title>
<!-- External JavaScript file "app.js" is added
using <script> tag with "src" attribute -->
<script src="app.js"></script>
</head>
<body>
</body>
</html>
Below JavaScript code is added to external file app.js
without adding the <script>
tag
document.write("Example to demo the External JavaScript")
OUTPUT 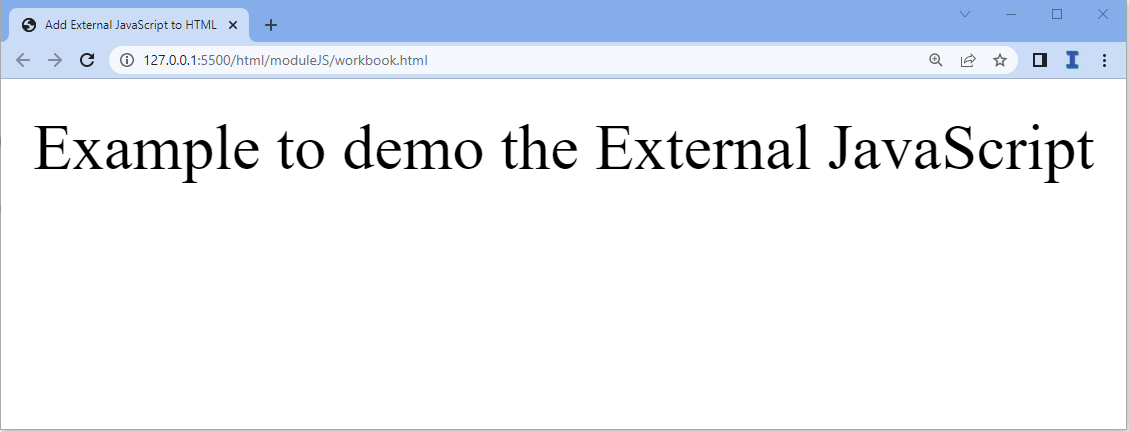
Display data in JavaScript
window.alert()
: method shows an alert box with the defined message and an OK button in an HTML document i.e. writing into an alert box.document.write()
: method aims to display some particular content in the browser window i.e. writing into the HTML output.console.log()
: method outputs a message to the web console. The message may be a single string (with optional substitution values), or it may be any one or more JavaScript objects.innerHTML
: property sets or returns the HTML content (inner HTML) of an element i.e. writing into an HTML element.NOTE: JavaScript does not have any print object or print methods. You cannot access output devices from JavaScript.
// Using alert() method - alert box with a message
alert("Hello World - By alert() method");
// Using write() method - write content to the Document
document.write("Hello World - By write() method");
// Using log() method - write message to the console
console.log("Hello World - By console.log() method");
// Using DOM selection & manipulation
document.getElementById("para").innerHTML =
"Hello World - By DOM selection & manipulation";
JavaScript Statement
( ; )
separate JavaScript statements.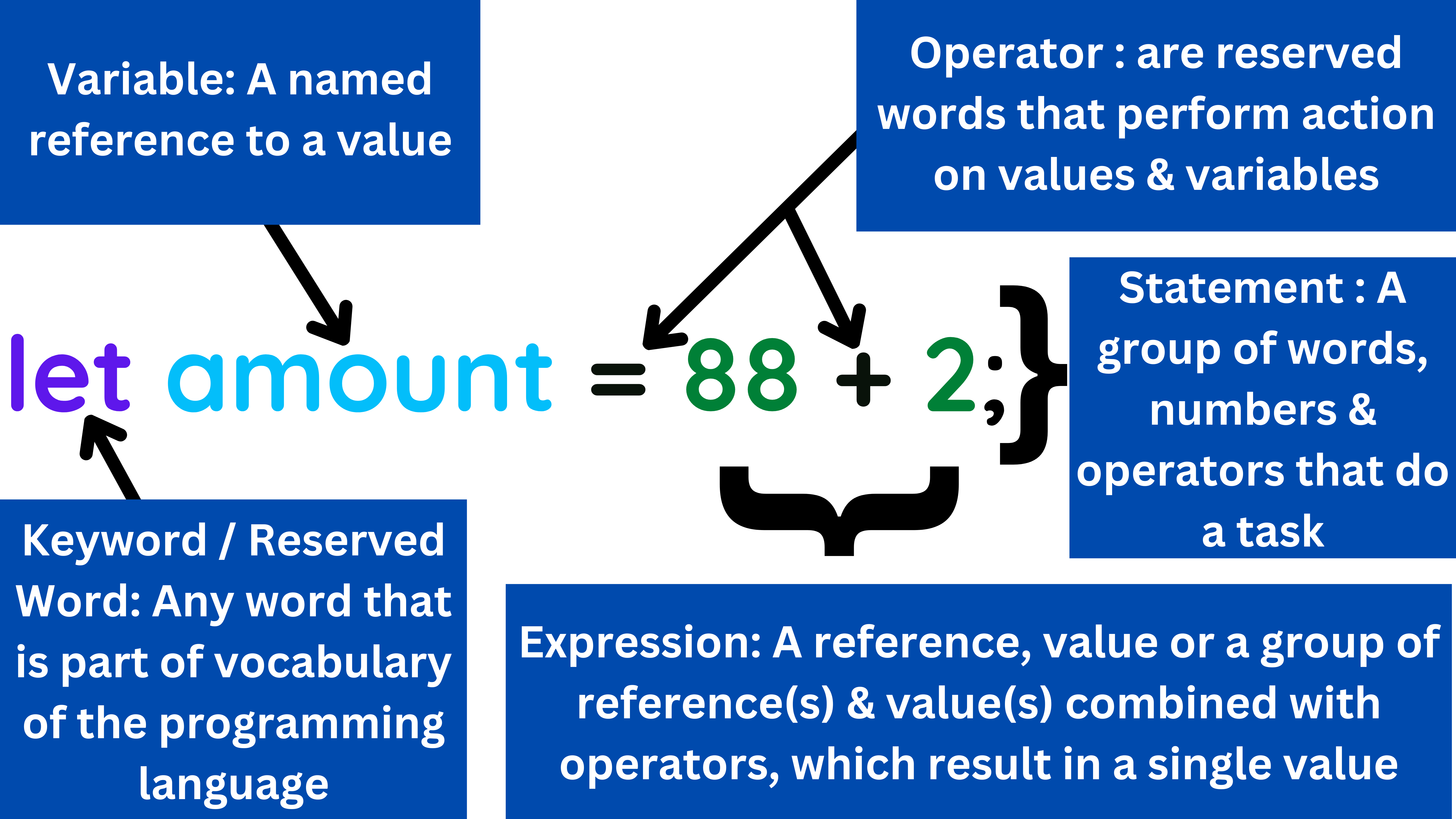
// JavaScript Statement
let amount = 88 + 2;
console.log(amount)
OUTPUT
90
Variables
We will now understand the semantics of identifier
keywords
variables
which are the main ways you store, reference, and operate on data within a JavaScript code.
Identifiers
name
given to identify a variable, function, class, module or other object.alphanumeric
characters, underscores (_)
, and dollar signs ($)
.Identifiers with special meanings: A few identifiers have a special meaning in some contexts without being reserved words of any kind. They include: arguments
, as
, async
, eval
, from
, get
, of
, set
Keywords
- (a).
function
- Declares a function - (b).
let
- Declares a block variable - (c).
try
- Implements error handling
NOTE: 16 reserved words in BOLD, have been removed from ECMAScript 5/6 standard.
abstract | double | in | super |
arguments | else | instanceof | switch |
await | enum | int | synchronized |
boolean | eval | interface | this |
break | export | let | throw |
byte | extends | long | throws |
case | false | native | transient |
catch | final | new | true |
char | finally | null | try |
class | float | package | typeof |
const | for | private | var |
continue | function | protected | void |
debugger | goto | public | volatile |
default | if | return | while |
delete | implements | short | with |
do | import | static | yield |
Variables
let
or const
.- - Using
var
- - Using
let
- - Using
const
- - Using
nothing
// Declare a JavaScript Variable
let $price = 12 // using let
const PI = 3.142 // using const
var premium = 12500.25 // using var
deductible = 500 // using nothing
console.log($price)
console.log(PI)
console.log(premium)
console.log(deductible)
OUTPUT
12 3.142 12500.25 500
Naming Variable
$
(dollar) and _
(underscores)
// Naming Variables & assignment
let price = 120 // variable names can contain letters
var Price = 24 // price & Price are different as JavaScript is case-sensitive
let interest_rate = 2.25 // '_' is a valid usage in variable name
const QTY = 3 // All-caps is valid usage in variable name
let acct101 = 'skillzam' // variable names can contain numbers
var $amount = 200.25 // variable names can contain '$' sign
let isGreater = true // variable names can contain combination of small & capital letters
JavaScript Variable Examples:
// JavaScript Variable Example
// Declare a JavaScript Variable using keywords var, let, const
var num1 = 5; // Variable name can contain numbers like num1
const num2 = 6;
let total = num1 + num2; // variable "total" will store value of 11 = ( 5 + 6 )
console.log("The value of total is: " + total)
OUTPUT
The value of total is: 11
// JavaScript Variable Example
// Variable names can start with "$" symbol like in $price1
const $price1 = 5;
const $price2 = 5;
let totalPrice = $price1 + $price2;
console.log("The total is: " + totalPrice)
OUTPUT
The total is: 10
// JavaScript Variable Example
// Variable names can start with "_" (underscore) symbol like in _name
let _name = "FIFA ";
_name += "World Cup 2022";
console.log(_name)
OUTPUT
FIFA World Cup 2022
// JavaScript Variable Example
// variable declared without a value will have the value undefined
let salary;
console.log("The salary amount is : " + salary)
OUTPUT
The salary amount is : undefined
// JavaScript Variable Example
// If you put a number in quotes, the rest of the numbers will be treated as strings, and concatenated
const intNum = 3;
const str = "31"; // Numbers within quotes will be treated as strings
let sumUp = str + intNum; // concatenation of number and string
console.log("Result (sumUp) is : " + sumUp + " and the typeof is : " + typeof(sumUp))
OUTPUT
Result (sumUp) is : 313 and the typeof is : string
Variable names with multiple words
1. Camel Case
Variable names where each word, except the first, starts with a capital letter:
let firstName = 'Jack'
2. Snake Case
Variable names where each word is separated by an underscore ( _ )
character:
let last_name = 'Sparrow'
3. Pascal Case
Variable names where each word starts with a capital letter:
var MyFavoriteTeam = 'F C Barcelona'
Keywords let
, const
, var
Ways to declare a JavaScript Variable:
- - Using
var
- - Using
let
- - Using
const
- - Using
nothing
When to use const
Always declare a variable with const
when you know that the value should not be changed. Use const
when you declare:
Block Scope
let
and const
let
or const
have Block Scope.var
cannot have block scope.
// JavaScript "let" Keyword to define variable
// Cannot redclare a block-scoped variable using (let)
let favColor = "BLUE";
let favColor = "BLACK"; // SyntaxError
console.log("Favorite color is : " + favColor)
OUTPUT
SyntaxError: Identifier 'favColor' has already been declared
Redeclaring Variables
let
or const
cannot be redeclared in the same block.let
, in another block, is allowedlet
keyword, will not redeclare the variable outside the block.var
is allowed anywhere in a program.var
keyword, will also redeclare the variable outside the block.
// JavaScript "var" Keyword to define variable
// Redeclaring a variable inside a block will also redeclare the variable outside the block
// var has "NO" block scope
var goals = 3; // Here goals variable container has the value 3
{
var goals = 2; // Here goals variable container has the value 2
}
// Here goals variable container has the value 2
console.log("Number of Goals scored : " + goals)
OUTPUT
Number of Goals scored : 2
// JavaScript "let" Keyword to define variable
// Redeclaring a variable with let, in another block, is ALLOWED
// let has block scope
let tax = 30; // ALLOWED - Here tax variable container has the value 30
{
let tax = 25; // ALLOWED - Here tax variable container has the value 25
console.log("Within Block scope : " + tax) // value 25
}
{
let tax = 33; // ALLOWED - Here tax variable container has the value 33
}
console.log("Outside the Block : " + tax) // Here tax variable container has the value 30
OUTPUT
Within Block scope : 25 Outside the Block : 30
Reassigning Variables
const
cannot be reassigned.let
or var
can be reassigned.
// JavaScript "const" Keyword to define variable
// "const" variable cannot be reassigned
try {
const PI = 3.141592653589793;
PI = 3.14; // TypeError
console.log(PI);
}
catch (err) {
console.log(err)
}
OUTPUT
TypeError: Assignment to constant variable.
JavaScript Hoisting
let
or const
are hoisted to the top of the block, but not initialized. This means block of code is aware of the variable, but it cannot be used until it has been declared.let
variable before it is declared will result in a ReferenceError
. The variable is in a "temporal dead zone" from the start of the block until it is declared.const
variable before it is declared, is a syntax errror, so the code will simply not run.var
are hoisted to the top and can be initialized at any time. This means you can use the variable before it is declared.
// JavaScript "let" Keyword to define variable
// With "let" , you CANNOT use a variable before it is declared
// "try" statement allows to define a block to be tested for errors while it is being executed
try {
countryName = "India"; // ReferenceError
let countryName;
console.log(countryName);
}
// "catch" statement allows to define a block to be executed, if an error occurs in try block
catch(err) {
console.log(err)
}
OUTPUT
ReferenceError: Cannot access 'countryName' before initialization
// JavaScript "const" Keyword to define variable
// Using "const" , before it is declared, is a syntax errror, so code will not run
cityName = "Bengaluru";
const cityName; // SyntaxError
console.log("City of residence : " + cityName)
OUTPUT
SyntaxError: Missing initializer in const declaration
JavaScript Operators
Now we'll dig into the semantics of the various operators included in JavaScript language.
Operators are reserved syntax consisting of punctuation or alphanumeric characters that carries out built-in functionality. For example, in JavaScript the addition operator +
adds numbers together and concatenates strings.
Operand is part of an instruction representing data manipulated by the operator
. For example, when you add two numbers, the numbers are the operand and "+" is the operator.
Listed below are the major Operator categories:
1. Arithmetic Operators
They are summarized in the following table:
Operator | Name | Description |
---|---|---|
a + b | Addition | Sum of a and b |
a - b | Subtraction | Difference of a and b |
a * b | Multiplication | Product of a and b |
a / b | True division | Quotient of a and b |
a % b | Modulus | Remainder after division of a by b |
a ** b | Exponentiation | a raised to the power of b |
++a | Pre Increment | returns operand 'a' after adding one |
a++ | Post Increment | returns operand 'a' before adding one |
--a | Pre Decrement | returns operand 'a' after substracting one |
a-- | Post Decrement | returns operand 'a' before substracting one |
-a | Unary Negation | The negative of a |
+a | Unary plus | a unchanged (rarely used) |
// addition, subtraction, multiplication
(12 + 34) * (12.3 - 4) // 381.8
// division, Modulus, Exponentiation
22 / 7 // 3.142857142857143
22 / 0 // Infinity
31 % 2 // 1
2 ** 3 // 8
// Increment Operator
// Pre Increment
let i = 9
j = ++i
console.log("i = " + i)
console.log("j = " + j)
// Post Increment
let x = 9
y = x++
console.log("x = " + x)
console.log("y = " + y)
OUTPUT
i = 10 j = 10 x = 10 y = 9
// Decrement Operator
// Pre Decrement
let a = 9
b = --a
console.log("a = " + a)
console.log("b = " + b)
// Post Decrement
let p = 9
q = p--
console.log("p = " + p)
console.log("q = " + q)
OUTPUT
a = 8 b = 8 p = 8 q = 9
2. Bitwise Operators
number.toString(2)
to convert number to binary.Bitwise operators are summarized in the following table:
Operator | Name | Description |
---|---|---|
a & b | Bitwise AND | Bits defined in both a and b |
a | b | Bitwise OR | Bits defined in a or b or both |
a ^ b | Bitwise XOR | Bits defined in a or b but not both |
~a | Bitwise NOT | Bitwise negation of a |
a << b | Zero fill left shift | Shift bits of a left by b units, shifting in zeros from the right. |
a >> b | Signed right shift | Shift bits of a right by b units, discarding bits shifted off. |
a >>> b | Zero fill right shift | Shift bits of a right by b units. discarding bits shifted off, and shifting in zeros from the left. |
// Number.toString(2) function returns the binary version of a specified integer
let num = 8
num.toString(2) // '1000'
// Bitwise AND
9 & 10 // 8
// 1001 (9 in Binary)
// & 1010 (10 in Binary)
//_______________________
// 1000 (8 in Binary)
// Bitwise OR
9 | 10 // 11
// 1001 (9 in Binary)
// | 1010 (10 in Binary)
//_______________________
// 1011 (11 in Binary)
// Bitwise XOR
9 ^ 10 // 3
// 1001 (9 in Binary)
// ^ 1010 (10 in Binary)
//_______________________
// 0011 (3 in Binary)
// Bitwise << 'Zero fill left shift'
4 << 1 // 8
// 0100 (4 in Binary)
// << 0001 (1 in Binary)
//_______________________
// 1000 (8 in Binary)
// Bitwise >> 'Signed right shift'
4 >> 1 // 2
// 0100 (4 in Binary)
// >> 0001 (1 in Binary)
//_______________________
// 0010 (2 in Binary)
// Bitwise >>> 'Zero fill right shift'
4 >>> 1 // 2
// 0100 (4 in Binary)
// >>> 0001 (1 in Binary)
//_______________________
// 0010 (2 in Binary)
// Bitwise NOT
// JavaScript uses 32 bits signed integers, it will not return 10. It will return -6.
// 00000000000000000000000000000101 (5)
// 11111111111111111111111111111010 (~5 = -6)
// A signed integer uses the leftmost bit as the minus sign
~5 // 10
// ~ 0101 (5 in Binary)
//_______________________
// 1010 (10 in Binary)
3. Assignment Operators
=
equal, which assigns the value of its right operand to its left operand.
// Assignment Operator (=) equal
let assignNum = 66
console.log(assignNum)
// 66
For example, to add 6 to assignNum
we write:
assignNum + 6
// 72
assignNum
with this new value; in this case, we could combine the addition and the assignment and write assignNum = assignNum + 6
. Because this type of combined operation and assignment is so common, JavaScript includes built-in update operators for all of the arithmetic operations:
// Assignment Operator with addition (+=)
let assignNum = 66
assignNum += 6 // equivalent to assignNum = assignNum + 6
console.log(assignNum)
// 72
Operator | Description | Same as |
---|---|---|
a += b | Add and equal operator | a = a + b |
a -= b | Subtract and equal operator | a = a - b |
a *= b | Asterisk and equal operator | a = a * b |
a /= b | Divide and equal operator | a = a / b |
a **= b | Exponent and equal operator | a = a ** b |
a %= b | Modulus and equal operator | a = a % b |
a &= b | Bitwise AND Assignment Operator | a = a & b |
a |= b | Bitwise OR Assignment Operator | a = a | b |
a ^= b | Bitwise XOR Assignment Operator | a = a ^ b |
a << = b | Bitwise Zero fill LEFT shift assignment operator | a = a << b |
a >>= b | Bitwise Signed RIGHT shift assignment operator | a = a >> b |
a &&= b | Logical AND assignment operator | a = a && b |
a ||= b | Logical OR assignment operator | a = a || b |
a ??= b | Nullish coalescing assignment operator | a = a ?? b |
Each one is equivalent to the corresponding operation followed by assignment: that is,
for any operator "■"
the expression a ■= b
is equivalent to a = a ■ b
with a slight catch.
// Demo the Add and Assignment Operators
let num1 = 6,
num2 = 2;
num1 += num2 // num1 = num1 + num2
console.log( "Value of num1 = " + num1)
OUTPUT
Value of num1 = 8
// Demo the Substract and Assignment Operators
let num1 = 6,
num2 = 2;
num1 -= num2 // num1 = num1 - num2
console.log( "Value of num1 = " + num1)
OUTPUT
Value of num1 = 4
// Demo the Multiple and Assignment Operators
let num1 = 6,
num2 = 2;
num1 *= num2 // num1 = num1 * num2
console.log( "Value of num1 = " + num1)
OUTPUT
Value of num1 = 12
// Demo the Divide and Assignment Operators
let num1 = 22,
num2 = 7;
num1 /= num2 // num1 = num1 / num2
console.log( "Value of num1 = " + num1)
OUTPUT
Value of num1 = 3.142857142857143
// Demo the Exponent and Assignment Operators
let num1 = 3,
num2 = 2;
num1 **= num2 // num1 = num1 ** num2
console.log( "Value of num1 = " + num1)
OUTPUT
Value of num1 = 9
// Demo the Modulus and Assignment Operators
let num1 = 7,
num2 = 4;
num1 %= num2 // num1 = num1 % num2
console.log( "Value of num1 = ", num1)
OUTPUT
Value of num1 = 3
// Demo the Bitwise AND Assignment Operator
let num1 = 6,
num2 = 13;
num1 &= num2 // num1 = num1 & num2
console.log( "Value of num1 = " + num1)
// 0110 (6 in binary)
// & 1101 (13 in binary)
// ________
// 0100 = 4 (In decimal)
OUTPUT
Value of num1 = 4
// Demo the Bitwise OR Assignment Operator
let num1 = 6,
num2 = 13;
num1 |= num2 // num1 = num1 | num2
console.log( "Value of num1 = " + num1)
// 0110 (6 in binary)
// | 1101 (13 in binary)
// ________
// 1111 = 15 (In decimal)
OUTPUT
Value of num1 = 15
// Demo the Bitwise XOR Assignment Operator
let num1 = 9,
num2 = 10;
num1 ^= num2 // num1 = num1 ^ num2
console.log( "Value of num1 = " + num1)
// 1001 (9 in Binary)
// ^ 1010 (10 in Binary)
//_______________________
// 0011 (3 in Binary)
OUTPUT
Value of num1 = 3
// Demo the Bitwise 'Zero fill LEFT shift' Assignment Operator
let num1 = 4,
num2 = 1;
num1 <<= num2 // num1 = num1 << num2
console.log("Value of num1 = " + num1)
// 0100 (4 in Binary)
// << 0001 (1 in Binary)
//_______________________
// 1000 (8 in Binary)
OUTPUT
Value of num1 = 8
// Demo the Bitwise 'Signed RIGHT shift' assignment operator
let num1 = 4,
num2 = 1;
num1 >>= num2 // num1 = num1 >> num2
console.log("Value of num1 = " + num1)
// 0100 (4 in Binary)
// >> 0001 (1 in Binary)
//_______________________
// 0010 (2 in Binary)
OUTPUT
Value of num1 = 2
// Demo the Logical AND assignment operator
let num1 = 9,
num2 = 10;
num1 &&= num2 // num1 = num1 && num2
console.log("Value of num1 = " + num1)
OUTPUT
Value of num1 = 10
// Demo the Logical AND assignment operator
let num1 = 9,
num2 = 10;
num1 ||= num2 // num1 = num1 || num2
console.log("Value of num1 = " + num1)
OUTPUT
Value of num1 = 9
// Demo Nullish coalescing assignment operator
// Also called logical nullish assignment operator
// only assigns if a is nullish (null or undefined) (a ??= b)
let num1 = null, // Oberve that num1 is null
num2 = 10;
num1 ??= num2 // num1 = num1 ?? num2
console.log("Value of num1 = " + num1)
OUTPUT
Value of num1 = 10
// Demo Nullish coalescing assignment operator
// On object data structures
const player = {
sport : 'hockey',
country : 'India'
}
// Note that 'name' key is undefined in 'player' object
// player.name = player.name ?? 'Dhanraj Pillay'
player.name ??= 'Dhanraj Pillay'
console.log("Value of player.name = " + player.name)
OUTPUT
Value of player.name = Dhanraj Pillay
4. Comparison Operators
true
and false
.===
and !==
operators, which perform strict equality and inequality comparisons. These operators do not attempt to convert the operands to compatible types before checking equality.Operation | Description |
---|---|
a == b | a equal to b |
a === b | a equal to b & has same type |
a != b | a not equal to b |
a < b | a less than b |
a > b | a greater than b |
a <= b | a less than or equal to b |
a >= b | a greater than or equal to b |
Comparison operators can be combined with the arithmetic
and bitwise
operators to express a virtually limitless range of tests for the numbers.
For example, we can check if a number is odd by checking that the modulus with 2 returns 1:
// 13 is odd
13 % 2 == 1 // returns true
// 24 is even
24 % 2 == 0 // returns true
// Equal and Strict Equal
let num1 = 99,
num2 = '99';
isEqual = (num1 == num2) // returns true
console.log(isEqual)
isStrictEqual = (num1 === num2) // returns false
console.log(isStrictEqual)
isNotEqual = (num1 != num2) // returns false
console.log(isNotEqual)
OUTPUT
true false false
We can string-together multiple comparisons to check more complicated relationships:
// string-together multiple comparisons
// Example : check if givenNum is between 9 and 19
let givenNum = 18
9 < givenNum < 19 // true
5. Logical Operators
Operator | Usage | Description |
---|---|---|
Logical AND | a && b | Returns a if it can be converted to false ; otherwise, returns b . Thus, when used with Boolean values, && returns true if both operands are true ; otherwise, returns false . |
Logical OR | a || b | Returns a if it can be converted to true ; otherwise, returns b . Thus, when used with Boolean values, || returns true if either operand is true ; if both are false , returns false . |
Logical NOT | !a | Returns false if its single operand that can be converted to true ; otherwise, returns true . |
// logical AND (&&)
const result1 = true && true; // t && t returns true
const result2 = false && false; // f && f returns false
const result3 = false && true; // f && t returns false
const result4 = false && 6 === 9; // f && f returns false
const result5 = 'bat' && 'ball'; // t && t returns 'ball'
const result6 = 'false' && 'false' // t && t returns 'false'
// logical OR (||)
const value1 = true || true; // t || t returns true
const value2 = false || false; // f || f returns false
const value3 = false || true; // f || t returns true
const value4 = false || 6 === 9; // f || f returns false
const value5 = 'bat' || 'ball'; // t || t returns 'bat'
const value6 = 'false' || 'false' // t || t returns 'false'
// logical NOT (!)
const op1 = !true; // !t returns false
const op2 = !false; // !f returns true
const op3 = !"code"; // !t returns false
const op4 = !null; // !f returns true
const op5 = !undefined; // !f returns true
// logical AND (&&) Example
let testNum = 11;
(testNum < 15) && (testNum > 10) // true
// Logical OR (||) Example
let testNum = 11;
(testNum > 9) || (testNum % 2 == 0) // true
// Logical NOT (!)
let testNum = 11;
!(testNum < 7) // true
Boolean algebra aficionados might notice that the XOR operator is not included; this can of course be constructed in several ways from a compound statement of the other operators.
Otherwise, a clever trick you can use for XOR of Boolean values is the following:
// (testNum > 1) ^ (testNum < 10) // return 0 or 1
let testNum = 11;
(testNum > 1) != (testNum < 10) // true
These sorts of Boolean operations will become extremely useful when we begin discussing control flow statements such as conditionals
and loops
6. Conditional Operators
if/else
statement.SYNTAX : variableName = (condition) ? valueOne : valueTwo
Conditional operator assigns a value to a variable based on some condition (true or false).
- (a). Expression consists of three operands: the
condition
,valueOne
andvalueTwo
. - (b). Evaluation of the
condition
should result in eithertrue/false
or a boolean value. - (c). The
true
value lies between “?” & “:” and is executed if thecondition
returnstrue
. Similarly, thefalse
value lies after “:” and is executed if the condition returnsfalse
.
// Conditional (Ternary) Operator
// Example to demo voting rights eligibility
let age = 24, // Actual Age
eligibleAge = 18, // Eligible Age
votingRights;
votingRights = (age > eligibleAge) ? "eligible to vote!" : "NOT eligible to vote!";
console.log("Age of the citizen is " +
age +
" years. \n" +
"Hence, citizen is " +
votingRights);
OUTPUT
Age of the citizen is 24 years. Hence, citizen is eligible to vote!
typeof()
Operator
typeof
operator returns a string indicating the type of a variable or unevaluated operand.Operand
is the string, variable, keyword, or object for which the type is to be returned.typeof
operator has higher precedence than binary operators like addition (+).SYNTAX :
typeof(operand)
typeof operand
Listed below are types returned from typeof
operator :
// typeof value returned from a 'integer' operand
const intNum = 123
typeof intNum
// 'number'
// typeof value returned from a 'decimal' operand
let decimalNum = 3.142
typeof decimalNum
// 'number'
// typeof value returned from a 'string' operand
let CompanyName = "Workzam"
typeof(CompanyName)
// 'string'
// typeof value returned from a 'boolean' operand
let isGreater = true
typeof(isGreater)
// 'boolean'
// typeof value returned from a 'unassigned' operand
let randNum;
typeof(randNum)
// 'undefined'
// typeof value returned from a 'null' operand
let tickerSym = null
typeof(tickerSym)
// 'object'
// typeof value returned from a 'bigint' operand
const largeNumber = 1234567890123456789012345678901234567890n;
typeof(largeNumber)
// 'bigint'
// typeof value returned from a 'symbol' operand
const player = {
fname: "Leo",
lname: "Messi",
position: "Forward"
};
let uid = Symbol('uid');
player[uid] = 98765;
typeof(uid)
// 'symbol'
// typeof value returned from a 'function' operand
function printVal () {
console.log('Hello')
}
typeof printVal
// 'function'
// typeof value returned from a 'array' operand
const cricketer = ['Sachin', 'Dhoni', 'Virat']
typeof cricketer
// 'object'
// typeof value returned from a 'object' operand
const player = {
fname: "Leo",
lname: "Messi",
position: "Forward"
};
typeof player
// 'object'
Data Types
Using immutable datatypes has several benefits :
- (1). To improve performance (no planning for the object's future changes)
- (2). To reduce memory use (make object references instead of cloning the whole object)
- (3). Thread-safety (multiple threads can reference the same object without interfering with one other)
- (4). Lower developer mental burden (the object's state won't change and its behavior is always consistent)
Seven primitive datatypes
true
/ false
)253- 1
)Object wrapper
Each primitive type (except for the types of undefined
and null
) has a corresponding wrapper class. The key purpose of these classes is to provide properties (mostly methods) for primitive values.
There are two ways of invoking wrapper classes:
Number
, String
and Boolean
can be instantiated via new
. Doing this (explicitly) is almost never useful for programmers.Datatype | typeof() return value |
Object wrapper |
---|---|---|
Number | "number" | Number |
String | "string" | String |
Boolean | "boolean" | Boolean |
Bigint | "bigint" | BigInt |
Symbol | "symbol" | Symbol |
Undefined | "undefined" | N/A |
Null | "object" | N/A |
Primitive Methods
- (1). Primitives are still primitive. A single value, as desired
- (2). JavaScript allows access to methods(functions) and properties of
string
,number
,boolean
,bigint
andsymbol
- (3). In order for that to work, a special “object wrapper” that provides the extra functionality is created, and then is destroyed.
- (4). Primitives
null
andundefined
are exceptions. They have no corresponding “wrapper objects” and provide no methods. In a sense, they are “the most primitive”.
For Example: There exists a string method slice()
that returns a substring of a string.
// slice() returns substring
let city = "Mumbai"
city.slice(0, 3) // returns substring
OUTPUT
'Mum'
number
number
is a built-in primitive data type in JavaScript.number
represents both integer and floating point numbers. Infinity
, -Infinity
and NaN
(Not a Number)._
as the separator, which plays the role of the “syntactic sugar”, it makes the number more readable. For Example: The number 1_000_000_000 represents a million.number
is capable of safely storing integers in the range - (253- 1) (Number.MIN_SAFE_INTEGER
) to 253- 1 (Number.MAX_SAFE_INTEGER
).number
is capable of storing positive floating-point numbers between 2-1074 (Number.MIN_VALUE
) and 21024 (Number.MAX_VALUE
)- (a). Positive values greater than
Number.MAX_VALUE
are converted to+Infinity
. - (b). Positive values smaller than
Number.MIN_VALUE
are converted to+0
. - (c). Negative values smaller than
- Number.MAX_VALUE
are converted to-Infinity
. - (d). Negative values greater than
- Number.MIN_VALUE
are converted to-0
.
// JavaScript DataType : number
const x = 5;
const y = 10.75;
const z = 0;
let value1 = x + y; // number datatype
let value2 = "string"/x; // NaN - Not a number
let value3 = x/z; // Infinity
console.log("value1 = " + value1 + " and typeof(value1) is " + typeof(value1))
console.log("value2 = " + value2 + " and typeof(value2) is " + typeof(value2))
console.log("value3 = " + value3 + " and typeof(value3) is " + typeof(value3))
OUTPUT
value1 = 15.75 and typeof(value1) is number value2 = NaN and typeof(value2) is number value3 = Infinity and typeof(value3) is number
// DataType number (integer/decimals) precision
let intNum1 = 999999999999999 // accurate up to 15 digits
let intNum2 = 9999999999999999 // round-off
let intNum3 = 1 / 3 // maximum number of decimals is 17
console.log(intNum1)
console.log(intNum2)
console.log(intNum3)
OUTPUT
999999999999999 10000000000000000 0.3333333333333333
// Number in Exponential notation
let num1 = 12345e10 // exponential notation (e)
let num2 = 12345e1000 // very large number
console.log(num1)
console.log(num2)
OUTPUT
123450000000000 Infinity
// Adding number and string
let academy = "Skillzam"
const num = 99
let addUp = academy + num // '+' operand will result in string datatype
console.log("addUp = " + addUp + " and typeof(addUp) is " + typeof(addUp))
OUTPUT
addUp = Skillzam99 and typeof(addUp) is string
NaN (Not a Number)
NaN
is a reserved word indicating that a number is not a legal number.
Five different types of operations that return NaN
:
parseInt("skillzam")
, Number(undefined)
, or implicit ones like Math.abs(undefined)
.Math
operation where the result is not a real number - Example: Math.sqrt(-1)0 * Infinity
, 1Infinity
, Infinity / Infinity
, Infinity - Infinity
NaN
is contagious - Example: 9NaN
, 7 * "workzam"
Date
new Date("skillzam").getTime()
, "".charCodeAt(1)
// Types of operations that return NaN - Not a Number
console.log(parseInt("skillzam")) // NaN
console.log(Number(undefined)) // NaN
console.log(Math.abs(undefined)) // NaN
console.log(Math.sqrt(-9)) // NaN
console.log(Infinity - Infinity) // NaN
console.log(9 ** NaN) // NaN
console.log(9 * "workzam") // NaN
console.log("".charCodeAt(9)) // NaN
let dTime = new Date("skillzam").getTime()
console.log(dTime) // NaN
Infinity or -Infinity
Infinity
is a numeric value representing infinity.Infinity
(positive infinity) is greater than any other number.Infinity
is the value JavaScript will return, if you calculate a number outside the largest possible number.
// Infinity or -Infinity
console.log(Infinity); // Infinity
console.log(Infinity * 9); // Infinity
console.log(Math.pow(9, 999)); // Infinity
console.log(Math.log(0)); // -Infinity
console.log(9 / Infinity); // 0
console.log(9 / 0); // Infinity
string
string
is a built-in primitive data type in JavaScript.string
represents a collection of alphanumeric characters within a single quotes '...'
or double quotes "..."
or Backticks `...`
string
, as long as they don't match the quotes surrounding the string.For Example: 'Camel is called the "ship" of the desert'
string
occupies a position in the string. The first element is at index
0, the next at index
1, and so on.String()
calls in a non-constructor context (that is, called without using the new
keyword) are primitive strings. new
keyword returns a string wrapper object.String
objects also give different results when using eval()
. Primitives passed to eval()
are treated as source code; String
objects are treated as all other objects are, by returning the object.
// Strings created using Single quotes ('...')
let academy = 'Skillzam'
console.log(academy)
OUTPUT
Skillzam
// Strings created using Double quotes ("...")
let tagLine = "Learn without limits!"
console.log(tagLine)
OUTPUT
Learn without limits!
// Strings created using Backticks (`...`)
let moreLine = `A CURE platform:
- Cross-Skill
- Up-Skill
- Re-Skill
- Expert-Skill`
console.log(moreLine)
OUTPUT
A CURE platform: - Cross-Skill - Up-Skill - Re-Skill - Expert-Skill
// Primitive string using String() method without "new" keyword
let techHiring = String('www.' + 'workzam' + '.com') // '+' is used for concatenation
console.log("techHiring = " + techHiring)
console.log("typeof(techHiring) is " + typeof(techHiring))
OUTPUT
techHiring = www.workzam.com typeof(techHiring) is string
// string wrapper object using "new" keyword
// String with new returns a string wrapper object
let playerType = new String("Midfielder")
let player = "Ronaldinho is a " + playerType
console.log("playerType = " + playerType)
console.log("typeof(playerType) is " + typeof(playerType))
console.log("player = " + player)
console.log("typeof(player) is " + typeof(player))
OUTPUT
playerType = Midfielder typeof(playerType) is object player = Ronaldinho is a Midfielder typeof(player) is string
object
type.
String {'Midfielder'}
0: "M"
1: "i"
2: "d"
3: "f"
4: "i"
5: "e"
6: "l"
7: "d"
8: "e"
9: "r"
length: 10
[[Prototype]]: String
[[PrimitiveValue]]: "Midfielder"
String Properties
Strings have three important properties:
// String contains characters/symbols
let strVar01 = "$killzam 2023"
let strVar02 = "ಕನ್ನಡ"
console.log("typeof strVar01 = " + typeof(strVar01))
console.log("typeof strVar02 = " + typeof(strVar02))
OUTPUT
typeof strVar01 = string typeof strVar02 = string
// Length of a given string using String attribute "length"
// It returns number of characters contained in a string including SPACES
let strVar03 = ' JAVASCRIPT '
console.log("Length of strVar03 = " + strVar03.length)
OUTPUT
Length of strVar03 = 12
// Strings are immutable
let fruit = "GATES"
fruit[0] = "D" // Using indexing, to change first charater to "D"
console.log(fruit) // No change in the original string value "GATES"
OUTPUT
GATES
Escape Characters
\
followed by the character you want to insert.Code | Description |
---|---|
\' | Single Quote |
\" | Double Quote |
\` | Backticks |
\\ | Backslash |
\n | New Line |
\b | Backspace |
\r | Carriage Return |
\t | Tab |
// Double quotes inside a string, which is surrounded by double quotes
var sentence = "Camel is called the "ship" of the desert" // SyntaxError
OUTPUT
SyntaxError: Unexpected identifier 'ship'
// Escape character \" to avoid SyntaxError
var sentence = "Camel is called the \"ship\" of the desert"
console.log(sentence)
OUTPUT
Camel is called the "ship" of the desert
Template literals
`...`
characters.- (1). multi-line strings
- (2). string interpolation with embedded expressions
- (3). tagged templates which are special constructs
Multi-line Strings
A string with multi-lines can be created by template Literals using backticks.
// Multi-line strings created using Backticks (`...`)
let multiLine = `A CURE platform:
- Cross-Skill
- Up-Skill
- Re-Skill
- Expert-Skill`
console.log(multiLine)
OUTPUT
A CURE platform: - Cross-Skill - Up-Skill - Re-Skill - Expert-Skill
String Interpolation
$
and curly braces { }
i.e. ${expression}
.
// String interpolation using single variable placeholders
let name = 'Michael Phelps'
console.log(`${name} is called 'Flying Fish'!`)
OUTPUT
Michael Phelps is called 'Flying Fish'!
// String interpolation using multiple variable placeholders
let name = 'Challenger Deep'
let location = 'Mariana Trench'
let txt = `The ${name} in the ${location}, is the deepest part of the ocean.`
console.log(txt)
OUTPUT
The Challenger Deep in the Mariana Trench, is the deepest part of the ocean.
// String Interpoltion using expression placeholder
// Solve Quadratic Equation: x**2 - 8x + 15 = 0
const a = 1
const b = -8
const c = 15
const root1 = `Value of root1 = ${(-b + ((b**2 - 4*a*c)**0.5)) / (2*a)}`
const root2 = `Value of root2 = ${(-b - ((b**2 - 4*a*c)**0.5)) / (2*a)}`
console.log("The roots of Quadratic Equation are :")
console.log(root1)
console.log(root2)
OUTPUT
The roots of Quadratic Equation are : Value of root1 = 5 Value of root2 = 3
Tagged Templates
()
when calling the literal.
// Template literals are also Tagged templates
const championName = 'Garry Kasparov'
const championAge = 22
const isChampion = true;
function tagChampion(strings, champName, champAge) {
let str0 = strings[0]; // ''
let str1 = strings[1]; // ' became the youngest ever undisputed World Chess Champion at '
let str2 = strings[2]; // '.'
if(isChampion) {
return `${str0}${champName}${str1}${champAge}${str2}`;
}
}
// creating tagged template by passing two arguments "championName" & "championAge"
const output = tagChampion`${championName} became the youngest ever undisputed World Chess Champion at ${championAge}.`;
console.log(output);
OUTPUT
Garry Kasparov became the youngest ever undisputed World Chess Champion at 22.
String concatenation, Indexing & Slicing
Three basic string operations:
String Concatenation
You can combine, or concatenate, two strings using the +
operator or or concat()
string method.
// String Concatenation using "+" Operator
let academy;
academy = 'Skill' + 'zam' // String Concatenation
console.log(academy)
OUTPUT
Skillzam
// String Concatenation using "concat()" string method
let fname = "Brendan"
let lname = 'Eich'
let fullname = fname.concat(" ", lname) // String Concatenation
console.log(fullname)
OUTPUT
Brendan Eich
String Indexing :
index
n
between two square brackets [ ]
immediately after the string.at()
method takes an integer value and returns the item at that index, allowing for positive and negative integers. Negative integers count back from the last item in the string/array.
// String Indexing
let rhyme = "Twinkle, Twinkle, Little Star"
rhyme[4] //returns the character at index position 4
OUTPUT
'k'
// Access an index beyond end of string,
// then JavaScript returns "undefined"
let rhyme = "Twinkle, Twinkle, Little Star"
rhyme[100] // returns "undefined"
OUTPUT
undefined
// Reverse Indexing using "at()" method
let river = "Kaveri"
let reverseIndex = river.at(-6) // allows for negative indexing as well
console.log(reverseIndex)
let beyondIndex = river.at(100) // returns "undefined"
console.log(beyondIndex)
OUTPUT
K undefined
String Slicing :
You can extract a portion of a string, called a substring. This substring is called Slice
slice()
method:
start
to end
(but not including).slice()
goes till the end of the string.slice()
also allows for negative values for start
and end
arguments, which means the position is counted from the string end (i.e. reverse indexing).end
argument is omitted, undefined, or cannot be converted to a number (using Number(end)
), or if end
>= str.length
, slice()
extracts to the end of the string.SYNTAX : slice(start [, end])
// slice() method returns the substring
let city = "Mumbai"
city.slice(0,3) // returns first three characters in string "Mumbai"
OUTPUT
'Mum'
It's important to note that, JavaScript won't raise an Error when you try to slice between boundaries that fall outside the starting or ending boundaries of a string.
// slice() boundaries that fall outside starting or ending
let city = "Mumbai"
city.slice(0,100) // returns entire string
OUTPUT
'Mumbai'
when you try to get a slice in which the entire range is out of bounds, Instead of raising an error, JavaScript returns the empty string ("")
.
// slice() method's entire range is out of bounds
let city = "Mumbai"
city.slice(6,10) // returns the empty string ''
OUTPUT
''
// slice() method with no second arguments
let stadium = "Maracanã"
stadium.slice(4) // start at position index 4, goes to end of string
OUTPUT
'canã'
// slice() method with negative values for start/end argument
let stadium = "Maracanã"
stadium.slice(-8,-4) // start at 8th position from right, end at 4th from right
OUTPUT
'Mara'
// slice() method with no arguments
// copying the entry string
let stadium = "Maracanã"
let newStadium = stadium.slice() // return entire string
console.log(newStadium)
OUTPUT
Maracanã
String Methods
object.method(parameters)
- (1).
slice()
:
returns part of the string from
start
toend
(but not including). If there is no second argument, thenslice()
goes till the end of the string.slice()
also allows for negative values forstart
andend
arguments, which means the position is counted from the string end (i.e. reverse indexing).
Ifend
parameter is omitted, undefined, or cannot be converted to a number (usingNumber(end)
), or ifend
>=str.length
,slice()
extracts to the end of the string.SYNTAX :
slice(start [, end])
- (2).
substring()
:
returns a new string containing characters of the calling string from (or between) the specified
index
(or indices). The difference betweenslice()
&substring()
is thatstart
andend
values less than 0 are treated as 0 insubstring()
.
Ifend
parameter is omitted,substring()
extracts characters to the end of the string.SYNTAX :
substring(start [, end])
- (3).
substr()
:
returns a portion of the string, starting at the specified index and extending for a given number of characters (
length
) afterwards. The difference betweenslice()
&substr()
is that the second parameter specifies thelength
of the extracted part.
Iflength
is omitted orundefined
, or ifstart + length >= str.length
,substr()
extracts characters to the end of the string.SYNTAX :
substr(start [, length])
- (4).
replace()
:
returns a new string with one, some, or all matches of a
pattern
replaced by areplacement
. Thepattern
can be a string or a RegExp, and thereplacement
can be a string or a function called for each match. Ifpattern
is a string, only the first occurrence will be replaced. The original string is left unchanged. By default, thereplace()
method is case sensitive.SYNTAX :
replace(pattern, replacement)
- (5).
replaceAll()
:
returns a new string with all matches of a
pattern
replaced by areplacement
. Thepattern
can be a string or a RegExp, and thereplacement
can be a string or a function to be called for each match. The original string is left unchanged. If pattern is a regex, then it must have the global/g
flag set, or aTypeError
is thrown.SYNTAX :
replaceAll(pattern, replacement)
- (6).
toUpperCase()
:
returns the calling string value converted to uppercase (the value will be converted to a string if it isn't one). This method does not affect the value of the string itself since JavaScript strings are immutable.
SYNTAX :
toUpperCase()
- (7).
toLowerCase()
:
returns the value of the string converted to lower case.
toLowerCase()
does not affect the value of the original string itself.SYNTAX :
toLowerCase()
- (8).
trim()
,trimStart()
,trimEnd()
:
removes whitespace from both sides of a string and returns a new string, without modifying the original string. To return a new string with whitespace trimmed from just one end, use
trimStart()
ortrimEnd()
.SYNTAX :
trim()
trimStart()
trimEnd()
- (9).
padStart()
,padEnd()
:
pads the current string with another string (multiple times, if needed) until the resulting string reaches the given length. In
padStart()
, padding is applied from the start of the current string. InpadEnd()
, padding is applied from the end of the current string. The default value forpadString
parameter is unicode "space" (" ") , character (U+0020).SYNTAX :
padStart(targetLength [, padString])
padEnd(targetLength [, padString])
- (10).
at()
:
returns the character at a specified index (position) in a string. This method allows for positive and negative integers as parameters. Negative integers count back from the last string character.
SYNTAX :
at(index)
- (11).
charCodeAt()
:
returns the unicode of the character at a specified
index
in a string. The return value is a integer between 0 and 65535 representing theUTF-16
code unitSYNTAX :
charCodeAt(index)
- (12).
split()
:
method takes a pattern and divides a String into an ordered list of substrings by searching for the pattern, puts these substrings into an array, and returns the array.
There are two argumentsseparator
andlimit
.separator
is a pattern describing where each split should occur.limit
is a non-negative integer specifying a limit on the number of substrings to be included in the array.SYNTAX :
split(separator [, limit])
- (13).
indexOf()
,lastIndexOf()
:
searches the entire calling string, and returns the index of the first occurrence of the specified substring. If the
searchString
not found, then the method returns-1
. The second argumentposition
is a number, the method returns the first occurrence of the specified substring at anindex
greater than or equal to the specified number.
lastIndexOf()
method returns the index of last occurrence of a specified text in a string.lastIndexOf()
return -1 if the text is not found. ThelastIndexOf()
methods searches backwards (from the end to the beginning), meaning: if the second parameter isposition
, the search starts at thatposition
index, and searches to the beginning of the string.
The difference betweensearch()
andindexOf()
issearch()
does not have secondposition
argument whereasindexOf()
cannot take regular expressions as search values.SYNTAX :
indexOf(searchString [, position])
lastIndexOf(searchString [, position])
- (14).
search()
:
executes a search for a match between a regular expression and String object i.e. this method searches a string or a regular expression in a String Object and returns the position of the match. If not match found, then the method will return
-1
.
The difference betweensearch()
andindexOf()
issearch()
does not have secondposition
argument whereasindexOf()
cannot take regular expressions as search values.SYNTAX :
search(searchString)
search(regex)
- (15).
includes()
:
performs a case-sensitive search to determine whether one string may be found within another string, returning boolean values
true
orfalse
as appropriate.SYNTAX :
includes(searchString [, position])
- (15).
startsWith()
:
determines whether a string begins with the characters of a specified string, returning boolean values of either
true
orfalse
as appropriate. If theposition
argument is not specified, then it will default to0
SYNTAX :
startsWith(searchString [, position])
- (16).
endsWith()
:
determines whether a string ends with the characters of a specified string, returning boolean values of either
true
orfalse
as appropriate. If theposition
argument is not specified, then it will default to0
SYNTAX :
endsWith(searchString [, position])
- (17).
raw()
:
static method is a tag function of template literals. This is similar to the
r
prefix in Python, or the@
prefix in C# for string literals. It's used to get the raw string form of template literals — that is, substitutions (e.g. ${amount}) are processed, but escape sequences (e.g. \n) are not.SYNTAX :
raw(strings, ...substitutions)
raw`templateString`
- (18).
repeat()
:
constructs and returns a new string which contains the specified number of copies of the string on which it was called, concatenated together. The argument
count
indicating the number of times to repeat the string.SYNTAX :
repeat(count)
- (19).
toString()
:
returns a string representing the specified string value.
SYNTAX :
toString()
- (20).
valueOf()
:
returns the primitive value of a
String
object.SYNTAX :
valueOf()
- (21).
match()
:
method retrieves the result of matching a string against a regular expression i.e it returns an array containing the results of matching a string against a string or a regular expression. If a regular expression does not include the g modifier (global search),
match()
will return only the first match in the string.SYNTAX :
match(searchString)
match(regex)
- (22).
matchAll()
:
returns an iterator of all results matching a string against a "string or regular expression" including capturing groups. If the parameter is a regular expression, the global flag
g
must be set, otherwise aTypeError
is thrown.SYNTAX :
matchAll(searchString)
matchAll(regex)
// slice() method returns the substring
let city = "Mumbai"
// returns first three characters in string "Mumbai"
city.slice(0,3)
OUTPUT
'Mum'
// substring() new string containing characters
let city = "Mumbai"
// returns last three characters in string "Mumbai"
city.substring(3,6)
OUTPUT
'bai'
// substr() returns a portion of the string
let city = "Newyork"
// returns first three chars in string "Newyork"
city.substr(0,3)
OUTPUT
'New'
// replace() replaces a pattern (string) with another
let academy = "Skillzam - Learn without limits!"
academy.replace('-','=>')
OUTPUT
'Skillzam => Learn without limits!'
// replace() replaces a pattern (RegExp) with string
// Regular expressions are written without quotes
let goat = "Ronaldo is the GOAT in football!"
// regular Expression - /i flag (case-insensitive)
const regex = /ronaldo/i;
goat = goat.replace(regex, 'Messi');
console.log(goat)
OUTPUT
Messi is the GOAT in football!
// replaceAll() replaces a string with string
let iFeel = 'I love cricket. Most popular sport is "cricket".'
iFeel = iFeel.replaceAll("cricket","football");
console.log(iFeel)
OUTPUT
I love football. Most popular sport is "football".
// replaceAll() replaces a RegExp with string
// Regular expressions are written without quotes
let whatIfeel = 'I love cricket. Cricket is a team sport. Most popular sport is "cricket".'
// regular expression - /g flag (global match)
whatIfeel = whatIfeel.replaceAll(/Cricket/g,"Football");
whatIfeel = whatIfeel.replaceAll(/cricket/g,"football");
console.log(whatIfeel)
OUTPUT
I love football. Football is a team sport. Most popular sport is "football".
// toUpperCase() - make string upper-case
const academy = "Skillzam - Learn without limits!"
academy.toUpperCase()
OUTPUT
'SKILLZAM - LEARN WITHOUT LIMITS!'
// toLowerCase() - make string lower-case
const pangram = "The quick brown FOX jumps over the lazy DOG."
const lowerPangram = pangram.toLowerCase()
console.log(lowerPangram)
OUTPUT
the quick brown fox jumps over the lazy dog.
// trim() - trimStart() - trimEnd()
// removes whitespace
let fastestBird = " Peregrine Falcon is the fastest bird in the world. "
let trimBird = fastestBird.trim() // removes whitespace from both sides of string
console.log(`${trimBird}`)
let trimEndBird = fastestBird.trimEnd() // removes whitespace from End of string only
console.log(`${trimEndBird}`)
fastestBird.trimStart() // removes whitespace from start of string only
OUTPUT
Peregrine Falcon is the fastest bird in the world. Peregrine Falcon is the fastest bird in the world. 'Peregrine Falcon is the fastest bird in the world. '
// padStart() - pads start of the current string
// Demo1 : padStart()
const numStart = '7';
console.log(numStart.padStart(3, '0')); // string output: 007
// Demo2 : padStart()
const cardNumber = '4321987612346789';
const lastFour = cardNumber.slice(-4);
const cardMask = lastFour.padStart(cardNumber.length, '*');
console.log(cardMask); // string output: ************6789
OUTPUT
007 ************6789
// padEnd() - pads end of the current string
// Demo1 : padEnd()
const numEnd = '7';
console.log(numEnd.padEnd(3, '0')); // string output: 700
// Demo2 : padEnd()
const cellNumber = '7173334444';
const firstThree = cellNumber.slice(0,3);
const cellMask = firstThree.padEnd(cellNumber.length, '*');
console.log(cellMask); // string output: 717*******
OUTPUT
700 717*******
// at() - returns the character at a specified Index
// Example of VIN - vehicle identification number
let vin = "1G8MB35B48Y105252" // 17 charaters VIN
const countryID = vin.at(0); // returns character at '0' index position
console.log("Country Code is : " + countryID)
OUTPUT
Country Code is : 1
// charCodeAt() - returns unicode of character at specified index
let holdingCompany = "Alphabet Inc."
const utf16 = holdingCompany.charCodeAt(0); // returns unicode 'A'
console.log(utf16)
OUTPUT
65
// split() - returns the array
// Example 1
let text = 'She sells seashells on the seashore.'
const wordsArray = text.split(' ') // returns array of words
console.log(wordsArray)
console.log(wordsArray[2])
OUTPUT
['She', 'sells', 'seashells', 'on', 'the', 'seashore.'] seashells
// split() - returns the array
// Example 2
let text = 'She sells seashells on the seashore.'
const charArray = text.split(''); // returns array of single characters
console.log(charArray)
console.log(charArray[1])
OUTPUT
['S', 'h', 'e', ' ', 's', 'e', 'l', 'l', 's', ' ', 's', 'e', 'a', 's', 'h', 'e', 'l', 'l', 's', ' ', 'o', 'n', ' ', 't', 'h', 'e', ' ', 's', 'e', 'a', 's', 'h', 'o', 'r', 'e', '.'] h
// split() - returns the array
// Example 3
let text = 'She sells seashells on the seashore.'
const newText = text.split(); // returns array with single "text" string
console.log(newText);
OUTPUT
['She sells seashells on the seashore.']
// indexOf() returns index of first occurrence of specified substring
// NOT specifying the second argument "position" will default to 0
let vibgyor = "violet indigo blue green yellow orange red"
const poistionIndigo = vibgyor.indexOf('indigo') // 'indigo' is at index 7
console.log("Index position where 'indigo' was found is " + poistionIndigo)
const poistionBrown = vibgyor.indexOf('brown') // 'brown' does not exists
console.log("Index position where 'brown' was found is " + poistionBrown)
OUTPUT
Index position where 'indigo' was found is 7 Index position where 'brown' was found is -1
// indexOf() returns index of occurrence of specified substring
// specifying the second argument "position"
let searchWhale = "The biggest whale in the world is Antarctic blue whale."
// negative position' argument will be assumed as 0 index position
const firstWhale = searchWhale.indexOf('whale',-15)
console.log("Index position where 'whale' was found is " + firstWhale)
// 'position' argument is specified as 17 index position
const secondWhale = searchWhale.indexOf('whale',17)
console.log("Index position where 'whale' was found is " + secondWhale)
OUTPUT
Index position where 'whale' was found is 12 Index position where 'whale' was found is 49
// lastIndexOf() returns index of last occurrence of specified substring
let searchWhale = "The biggest whale in the world is Antarctic blue whale."
const lastWhale = searchWhale.lastIndexOf('whale', searchWhale.length)
console.log("Index position of last occurance of 'whale' is " + lastWhale)
OUTPUT
Index position of last occurance of 'whale' is 49
// search() returns the position of first match of string/RegExp
// Argument passed is a "string"
const academy = "Skillzam - Learn without limits!"
let matchIndex = academy.search('Learn') // search for first match of 'Learn'
console.log(`The first match for searchString occurs at index position ${matchIndex}`)
OUTPUT
The first match for searchString occurs at index position 11
// search() returns the position of first match of string/RegExp
// Argument passed is a "Regular Expression"
const academy = "Skillzam - Learn without limits!"
// Any character which is not word or whitespace
const regex = /[^\w\s]/g
// Search for first match of regex '-'
let matchIndex = academy.search(regex)
let matchChar = academy[academy.search(regex)]
console.log(`The first match for character "${matchChar}" is at index position ${matchIndex}`)
OUTPUT
The first match for character "-" is at index position 9
// includes() returns true/false depending on the strind found
// NOT specifying the second argument "position" will default to 0
const rhyme = "Baa, baa, black sheep, have you any wool?"
const isExists = rhyme.includes('baa') // 'baa' exits and returns true
console.log(isExists)
OUTPUT
true
// includes() returns true/false depending on the strind found
// specifying the second argument "position"
const rhyme = "Baa, baa, black sheep, have you any wool?"
// Check if a string includes "Baa" - start at position 10
let isExists = rhyme.includes('Baa',10)
console.log(isExists)
OUTPUT
false
// startsWith() returns whether a string begins with the characters
const mountain = "Mount Kilimanjaro is the highest mountain in Africa"
let doesBegin = mountain.startsWith('Mount')
console.log(doesBegin)
OUTPUT
true
// startsWith() returns whether a string begins with the characters
// specifying the second argument "position"
const mountain = "Mount Kilimanjaro is the highest mountain in Africa"
// Check if a string starts with "Kili" - start at position 10
let doesBegin = mountain.startsWith('Kili', 10)
console.log(doesBegin) // specify position as '6', it will return true
OUTPUT
false
// endsWith() returns whether a string ends with the characters
const mountain = "Mount Kilimanjaro is the highest mountain in Africa"
let doesEnd = mountain.endsWith('Africa')
console.log(doesEnd)
OUTPUT
true
// endsWith() returns whether a string ends with the characters
// specifying the second argument "position"
const mountain = "Mount Kilimanjaro is the highest mountain in Africa"
// Check if first 32 characters of a string ends with "highest"
let doesEnd = mountain.endsWith('highest', 32)
console.log(doesEnd)
OUTPUT
true
// raw() returns raw string form of a given template literal
// substitutions are processed, but escape sequences are not
const filePath = `C:\Users\Skillzam\Desktop\code\script.js`
console.log(filePath) // Observer the escape characters being ommited
const scriptPath = String.raw`C:\Users\Skillzam\Desktop\code\script.js`
console.log(`JavaScript file is located at ${scriptPath}`)
OUTPUT
C:UsersSkillzamDesktopcodescript.js JavaScript file is located at C:\Users\Skillzam\Desktop\code\script.js
Notice the first argument is an object with a raw
property, whose value is an array-like object (with a length
property and integer indexes) representing the separated strings in the template literal. The rest of the arguments are the substitutions. Since the raw
value can be any array-like object, it can even be a string!
For example, 'ABCD' is treated as ['A', 'B', 'C', 'D']. The following is equivalent to `A${0}B${1}C${2}D`
// raw() returns raw string form of a given template literal
// substitutions are processed
let text = String.raw({ raw: "ABCD" }, 0, 1, 2);
console.log(text)
OUTPUT
A0B1C2D
// repeat() returns a string with a number of copies
// Example 1
let word = 'buz'
let newWord = word + 'z'.repeat(5)
console.log(newWord)
OUTPUT
buzzzzzz
// repeat() returns a string with a number of copies
// Example 2
let website = 'w'.repeat(3).concat('.workzam.com')
console.log(website)
OUTPUT
www.workzam.com
// repeat() returns a string with a number of copies
// Example 3
let academy = 'Skillzam '
academy = academy.repeat(3)
console.log(academy)
OUTPUT
Skillzam Skillzam Skillzam
// toString() returns a string representation
// new keyword will create a object from a constructor function (String())
const academyObj = new String('Skillzam');
console.log(academyObj);
const academy = academyObj.toString()
console.log(academy);
OUTPUT
String {'Skillzam'} Skillzam
// valueOf() returns the primitive value
const jsObj = new String('JavaScript is everywhere.');
console.log(jsObj);
console.log(jsObj.valueOf());
OUTPUT
String {'JavaScript is everywhere.'} JavaScript is everywhere.
// match() return array containing results of matching string
// Argument passed is a "string"
let tongueTwister = `Betty Botter bought a bit of butter,
but the bit of butter was bitter,
so Betty Botter bought a bit of better butter,
to make the bit of bitter butter better.`
const matchResult = tongueTwister.match('butter')
console.log(matchResult)
OUTPUT
['butter']
// match() return array containing results of matching Regex
// Argument passed is a "Regex" with 'g' modifier (global search )
let tongueTwister = `Betty Botter bought a bit of butter,
but the bit of butter was bitter,
so Betty Botter bought a bit of better butter,
to make the bit of bitter butter better.`
const regex = /butter/g // regex with g modifier (global search )
const matchResult = tongueTwister.match(regex)
console.log(matchResult)
OUTPUT
['butter', 'butter', 'butter', 'butter']
// match() return array containing results of matching Regex
// Argument passed is a "Regex"
// using global(g) and ignoreCase(i) flags with match()
let alphabets = "ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz"
const regex = /[A-D]/gi // regex with global & ignoreCase flags
const matchResults = alphabets.match(regex);
console.log(matchResults)
OUTPUT
['A', 'B', 'C', 'D', 'a', 'b', 'c', 'd']
// matchAll() return iterator of all results matching a "string"
// Argument passed is a "string"
let tongueTwister = `Betty Botter bought a bit of butter,
but the bit of butter was bitter,
so Betty Botter bought a bit of better butter,
to make the bit of bitter butter better.`
const iterator = tongueTwister.matchAll("butter") // string as argument
console.log(iterator)
const resultArray = Array.from(iterator)
console.log(resultArray)
OUTPUT
[object RegExp String Iterator] [['butter'], ['butter'], ['butter'], ['butter']]
// matchAll() return iterator of all results matching a "regex"
// Argument passed is a "Regex" with 'g' modifier (global search )
let tongueTwister = `Betty Botter bought a bit of butter,
but the bit of butter was bitter,
so Betty Botter bought a bit of better butter,
to make the bit of bitter butter better.`
const regex = /butter/g // regex with g modifier (global search )
const iterator = tongueTwister.matchAll(regex)
console.log(iterator)
const resultArray = Array.from(iterator)
console.log(resultArray)
OUTPUT
[object RegExp String Iterator] [['butter'], ['butter'], ['butter'], ['butter']]
boolean
boolean
is a built-in primitive data type in JavaScript.true
or false
.Boolean()
object constructor.Boolean()
constructor with new
to convert a non-boolean value to a boolean value — use Boolean
as a function or a double NOT !!
instead.false
if equal to zero or null, and true
otherwise:
// Boolean values numeric type
Boolean(2017) // true
Boolean(-123) // true
Boolean(2.728281) // true
Boolean(Infinity) // true
Boolean(-Infinity) // true
Boolean(0) // false
Boolean(NaN) // false
Boolean()
is false
for empty strings and true
otherwise:
// Boolean values for string type
Boolean("Workzam") // true
Boolean("2023") // true
Boolean('') // false
// Boolean values for other values
Boolean(true) // true
Boolean([12,24,36]) // true
Boolean({name: 'Brendan'}) // true
Boolean([]) // true
Boolean({}) // true
Boolean() // false
Boolean(false) // false
Boolean(null) // false
Boolean(undefined) // false
Truthy & Falsy values
if
or else if
or while
condition or as operand of the Boolean operations.- ➤ false
- ➤ 0 (zero)
- ➤ NaN
- ➤ '' (empty string)
- ➤ null
- ➤ undefined
nullish
value is the value which is either null
or undefined
. Nullish values are always falsy.
// Falsy values
Boolean(false) // false
Boolean(0) // false
Boolean(NaN) // false
Boolean('') // false
Boolean(null) // false
Boolean(undefined) // false
Boolean Methods
- (1).
toString()
:
returns a string of either
true
orfalse
depending upon the value of the object.SYNTAX :
toString()
- (2).
valueOf()
:
returns the primitive value of a
Boolean
object.SYNTAX :
valueOf()
// toString() returns string of either true or false
// new keyword will create a object from a constructor function (Boolean())
const boolAcademyObj = new Boolean('Skillzam');
console.log(boolAcademyObj);
const academy = boolAcademyObj.toString()
console.log(academy)
console.log(typeof(academy))
OUTPUT
Boolean {true} true string
// valueOf() returns the primitive value
const boolworkzamObj = new Boolean('Workzam')
console.log(boolworkzamObj)
const techHire = boolworkzamObj.valueOf()
console.log(techHire)
console.log(typeof(techHire))
OUTPUT
Boolean {true} true boolean
undefined
undefined
is a built-in primitive data type in JavaScript.undefined
. The type is also undefined
.undefined
.undefined
. An empty string has both a legal value and a type string
undefined
is false.
// undefined datatype
let studentCount;
typeof(studentCount)
OUTPUT
'undefined'
// undefined boolean value
let btc
Boolean(btc)
OUTPUT
false
// undefined datatype used in "if"
let premium;
if (typeof(premium) === "undefined") {
console.log("Premium value not assigned.")
}
typeof(premium)
OUTPUT
Premium value not assigned. 'undefined'
// undefined - any variable can be emptied
let price = 200
price = undefined // NOT recommend doing this
console.log(price)
OUTPUT
Premium value not assigned. 'undefined'
null
null
is a built-in primitive data type in JavaScript.null
is a special value which represents “nothing”, “empty” or “value unknown”.null
is not the same as 0, false, or an empty string. null is a data type of its own.null
value represents the intentional absence of any object value. It is treated as falsy for boolean operations.null
is not an identifier for a property of the global object, like undefined
can be. Instead, null
expresses a lack of identification, indicating that a variable points to no object.
// null Datatype
const studentCount = null
console.log(studentCount)
console.log(typeof(studentCount)) // typeof null
console.log(Boolean(studentCount)) // Boolean value of null
OUTPUT
null object false
Difference between null
and undefined
//null and undefined
typeof null // "object" (not "null" for legacy reasons)
typeof undefined // "undefined"
null === undefined // false
null == undefined // true
null === null // true
null == null // true
!null // true
isNaN(1 + null) // false
isNaN(1 + undefined) // true
bigint
bigint
is a built-in primitive data type in JavaScript.bigint
values represent numeric values which are too large to be represented by the number
primitive.bigint
is created by appending n
to the end of an integer literal, or by calling the BigInt()
function (without the new
operator) and giving it an integer value or string value.bigint
variables can also be created using the BigInt()
object constructor method. BigInt() can only be called without new
. Attempting to construct it with new throws a TypeError
. Syntax : BigInt(value)
bigint
value cannot be used with methods in the built-in Math
object like the number
datatype.bigint
value cannot be mixed with a number
value in operations.0n
is falsy, everything else is truthy.bigint
value is not strictly equal to a number
value. Example : 1n !== 1 , this is truebigint
values and number
values may be mixed in arrays and sorted.bigint
value follows the same conversion rules as number
when: - (1). it is converted to a
boolean
: via theBoolean()
function - (2). when used with logical operators
||
,&&
, and!
- (3). within a conditional test like an
if
statement
// bigint explained in examples
// Example 1
// create bigint variable
const largeNum = 9007199254740992n // (Number.MAX_SAFE_INTEGER + 1)
console.log(largeNum) // 9007199254740992n
console.log(typeof(largeNum)) // bigint
// bigint explained in examples
// Example 2
// typeof Operator
typeof(999n) === 'bigint' // true
typeof(Object(999n)) === 'object' // true
// bigint explained in examples
// Example 3
// Operators
let bigNum = BigInt(9007199254740992) // BigInt() : 9007199254740992n
const bigNumPlus = bigNum + 7n // Addition : 9007199254740999n
const bigNumMinus = bigNum - 7n // Substract : 9007199254740992n
const bigNumProd = bigNum * 2n // Multiply : 18014398509481984n
const bigNumBy = 10n / 3n // Divide : 3n (decimal places are truncated)
const bigNumMod = bigNum % 10n // Mod : 2n
const bigNumPow = 2n ** 53n // Power : 9007199254740992n
// bigint explained in examples
// Example 4
// Comparisons
9n === 9 // false
9n == 9 // true
9n > 99 // false
9n <= 9 // true
// Conditionals
!9n // false
!0n // true
// bigint explained in examples
// Example 5
// Array
mixedArray = [8, 2n, 0, -6n, 10, 0n] // BigInt & Number values may be mixed
Bigint Methods
- (1).
toString()
:
returns a string representing this
BigInt
value in the specified radix (base).SYNTAX :
toString(radix)
// toString() returns string representing BigInt value
// Without "radix" argument
// number dataype with big Value
const hugeNumber = 1234567890123456789012345678901234567890
console.log(`hugeNumber value is ${hugeNumber} and datatype is ${typeof(hugeNumber)}`)
// create a bigint object from a constructor function - Bigint()
const bigintNumObj = BigInt(hugeNumber)
console.log(`bigintNumObj value is ${bigintNumObj} and datatype is ${typeof(bigintNumObj)}`)
// apply toString() method to bigintNumObj
const largeNumber = bigintNumObj.toString()
console.log(`largeNumber value is ${largeNumber} and datatype is ${typeof(largeNumber)}`)
OUTPUT
hugeNumber value is 1.2345678901234568e+39 and datatype is number bigintNumObj value is 1234567890123456846996462118072609669120 and datatype is bigint largeNumber value is 1234567890123456846996462118072609669120 and datatype is string
// toString() returns string representing BigInt value
// with "radix" argument
const bigN = 9007199254740991n
bigN.toString(2) // '11111111111111111111111111111111111111111111111111111'
bigN.toString(10) // '9007199254740991'
bigN.toString(16) // '1fffffffffffff'
- (2).
valueOf()
:
returns the wrapped primitive value of a
BigInt
object.SYNTAX :
bigIntObj.valueOf()
// valueOf() returns the primitive value
// BigInt variable created by adding 'n' at the end
const largeNum = 1234567890123456789012345678901234567890n
// Using BigInt() method, BigInt variable created
const bigNum = BigInt(9007199254740992)
const largeNumValue = largeNum.valueOf()
const bigNumValue = bigNum.valueOf()
console.log(largeNumValue) // 1234567890123456789012345678901234567890n
console.log(typeof(largeNumValue)) // bigint
console.log(bigNumValue) // 9007199254740992n
console.log(typeof(bigNumValue)) // bigint
OUTPUT
1234567890123456789012345678901234567890n bigint 9007199254740992n bigint
symbol
symbol
is a built-in primitive data type in JavaScript.symbol
represents a unique "hidden" identifier that no other code can accidentally access.object
are used to store collections of data. The symbol
type is used to create unique identifiers for objects.symbol
type doesn't have a literal form. To create a new symbol, you use the global Symbol()
method/function.Symbol()
function creates a new unique value each time you call it. The function accepts a description
as an optional argument. The description
argument will make your symbol
more descriptive. Attempting to construct it with new throws a TypeError
.
Syntax :
Symbol(description)
// symbol DataType creation using Symbol() method
// with 'description' argument as 'pid'
const player = {
fname: "Leo",
lname: "Messi",
position: "Forward" }
let pid = Symbol('pid') // description argument as 'pid'
player[pid] = 98765;
console.log('Player pid using Symbol: ' + player[pid])
console.log('typeof(pid) is ' + typeof(pid))
console.log('Player pid using Object: ' + player.pid)
OUTPUT
Player pid using Symbol: 98765 typeof(pid) is symbol Player pid using Object: undefined
Shared Symbols in global registry
Symbol()
function will create a Symbol pid
whose value (98765
) remains unique throughout the lifetime of the program.for()
and keyFor()
methods.Symbol.for(key)
- (1).
Symbol.for(key)
method takes a stringkey
as argument and returns asymbol value
from the registry. - (2). To create a symbol that will be shared, use the
Symbol.for()
method instead of calling theSymbol()
function. - (3).
Symbol.for(key)
method accepts a single parameter that can be used for symbol's description. - (4).
Symbol.for(key)
method first searches for the symbol with thekey
in the global symbol registry. It returns the existingsymbol
if there is one. Otherwise, theSymbol.for(key)
method creates a new symbol, registers it to the global symbol registry with the specifiedkey
, and returns thesymbol
.
Symbol.keyFor(symbol)
- (1).
Symbol.keyFor()
method takes asymbol value
and returns the stringkey
corresponding to it. - (2). To get the
key
associated with a symbol, you use theSymbol.keyFor()
method. - (3). If a
symbol
that does not exist in the global symbol registry, theSymbol.keyFor()
method returnsundefined
.
// Symbol.for() method
let aadhar = Symbol.for('aadhar')
let citizenID = Symbol.for('aadhar')
console.log(aadhar) // Symbol(aadhar)
console.log(citizenID) // Symbol(aadhar)
console.log(aadhar === citizenID) // true
console.log(typeof aadhar) // symbol
console.log(typeof citizenID) // symbol
// Symbol.keyFor() method
let keyAadhar = Symbol.keyFor(aadhar)
let keyCitizenID = Symbol.keyFor(citizenID)
console.log(keyAadhar) // aadhar
console.log(keyCitizenID) // aadhar
Symbol Methods
- (1).
toString()
:
returns a string containing the description of the
Symbol
.SYNTAX :
toString()
// toString() returns string containing description of Symbol
Symbol('skill').toString() // "Symbol(skill)"
Symbol.iterator.toString() // "Symbol(Symbol.iterator)
Symbol.for('zam').toString() // "Symbol(zam)"
- (2).
valueOf()
:
method returns the primitive value of a
Symbol
object.SYNTAX :
valueOf()
// valueOf() returns the primitive value of Symbol
const symbolSSN = Symbol('ssn')
typeof (symbolSSN) // "symbol"
symbolSSN.valueOf() // Symbol(ssn)
typeof Object(symbolSSN) // "object"
typeof Object(symbolSSN).valueOf() // "symbol"
Arrays
const
keyword. It does NOT define a constant array. It defines a constant reference to an array.length
property will determine the length of an array.SYNTAX:
const arrayName = [item1, item2, item2, ...];
// Array Indexing Example
const academy = ['S','K','I','L','L','Z','A','M']
console.log("type of academy array is " + typeof(academy))
console.log("Length of academy array is " + academy.length)
console.log("The element at the '0' index is " + academy[0])
OUTPUT
type of academy array is object Length of academy array is 8 The element at the '0' index is S
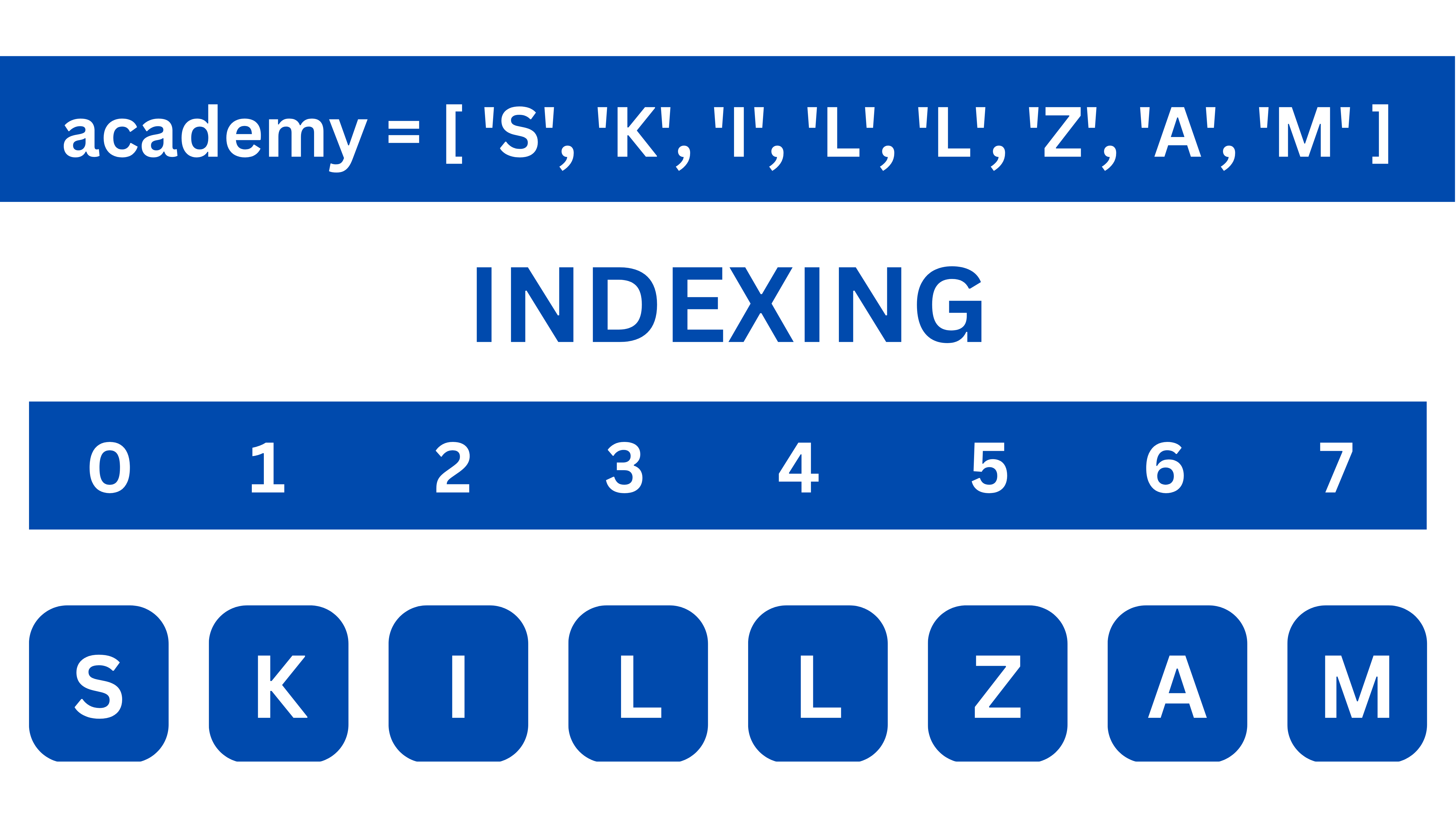
// Create array of English Vowels (string)
// using array literals
const vowels = ['a', 'e', 'i', 'o', 'u']
console.log(vowels)
OUTPUT
['a', 'e', 'i', 'o', 'u']
// Create array of Fibonacci series (number)
// using array literals
const fibo = [0, 1, 1, 2, 3, 5, 8, 13, 21, 34, 55, 89]
console.log(fibo)
OUTPUT
[0, 1, 1, 2, 3, 5, 8, 13, 21, 34, 55, 89]
// Create array of elements of different datatype
let three = "Three";
const mixData = []; // creating an empty array
// Adding elements to array
mixData[0] = 'One' // Array element is a string
mixData[1] = 2 // Array element is a integer
mixData[2] = three // Array element is a variable
mixData[3] = [4, "five", 6] // Array element is an array
mixData[4] = 7.8 // Array element is a decimal
mixData[5] = {key:9} // Array element is an object
console.log(mixData)
OUTPUT
['One', 2, 'Three', [4, 'five', 6], 7.8, {key: 9}]
Creating array
Arrays may be created in several ways:
[ ]
[a], [a, b, c]
split()
: 'SKILLZAM'.split('')
returns ['S', 'K', 'I', 'L', 'L', 'Z', 'A', 'M']
Array(item1, item2, item2,...)
or Array(arrayLength)
Array()
can be called with or without new
keyword. Both create a new Array instance.
The constructor builds a array whose items are the same and in the same order as iterable's items.
For example
Array('a','b','c')
returns ['a', 'b', 'c']
Array(1, 2, 3)
returns [1, 2, 3]
If the only argument passed to the Array()
constructor, is an integer between 0 and 232 - 1 (inclusive), this returns a new JavaScript array with its length
property.
Array(3)
returns [,,]
// Creating array using pair of square brackets
// Example: array of single digit even number
// creating Empty Array
const arrayOne = []
// Adding elements/items using index
arrayOne[0] = 6
arrayOne[1] = 28
arrayOne[2] = 496
arrayOne[3] = 8128
console.log(arrayOne)
OUTPUT
[6, 28, 496, 8128]
// Creating arrays using different ways
// use of 'new' keyword is optional
const arrayTwo = [[1],[2,3],[4,5,6]] // array from square brackets, separating items with commas
const arrayThree = new Array("WORKZAM") // array from single string argument
const arrayFour = new Array(9) // empty array with length 9
const arrayFive = "ಅ,ಆ,ಇ,ಈ".split(',') // array from string method split()
console.log(arrayTwo)
console.log(arrayThree)
console.log(arrayFour)
console.log(arrayFive)
OUTPUT
[[1],[2,3],[4,5,6]] ['WORKZAM'] [,,,,,,,,] ['ಅ', 'ಆ', 'ಇ', 'ಈ']
Working with array
// Add item to an array
const shoppingArray = ["tea", "coffee", "milk", "eggs"]
shoppingArray[4] = "honey"
console.log(shoppingArray)
OUTPUT
['tea', 'coffee', 'milk', 'eggs', 'honey']
// Modify item in an array
const shoppingArray = ["tea", "coffee", "milk", "eggs", "honey"]
shoppingArray[4] = "bread" // Change item from 'honey' to 'bread'
console.log(shoppingArray)
OUTPUT
['tea', 'coffee', 'milk', 'eggs', 'bread']
delete
.
Using
delete
creates sparse array i.e. an array which the elements are not sequential, and they don't always start at 0
.
They are essentially Array's with "holes", or gaps in the sequence of their indices.
// using "delete" remove item from array
const shoppingArray = ["tea", "coffee", "milk", "eggs", "bread"]
delete shoppingArray[0] // Remove the last item "honey" in the array
console.log(shoppingArray)
console.log(shoppingArray[0]) // deleted element at index(0) is removed
console.log(shoppingArray.length) // no change in length of array
OUTPUT
['tea', 'coffee', 'milk', 'eggs'] undefined 5
string
.
// Concatenation of arrays
const letters = ['A','B','C']
const numbers = [1,2,3]
let result = letters + numbers // Concatenation using '+' operator
console.log(result)
console.log("type of 'result' is " + typeof(result))
OUTPUT
A,B,C1,2,3 type of 'result' is string
Nested array
rows (r)
and columns (c)
. 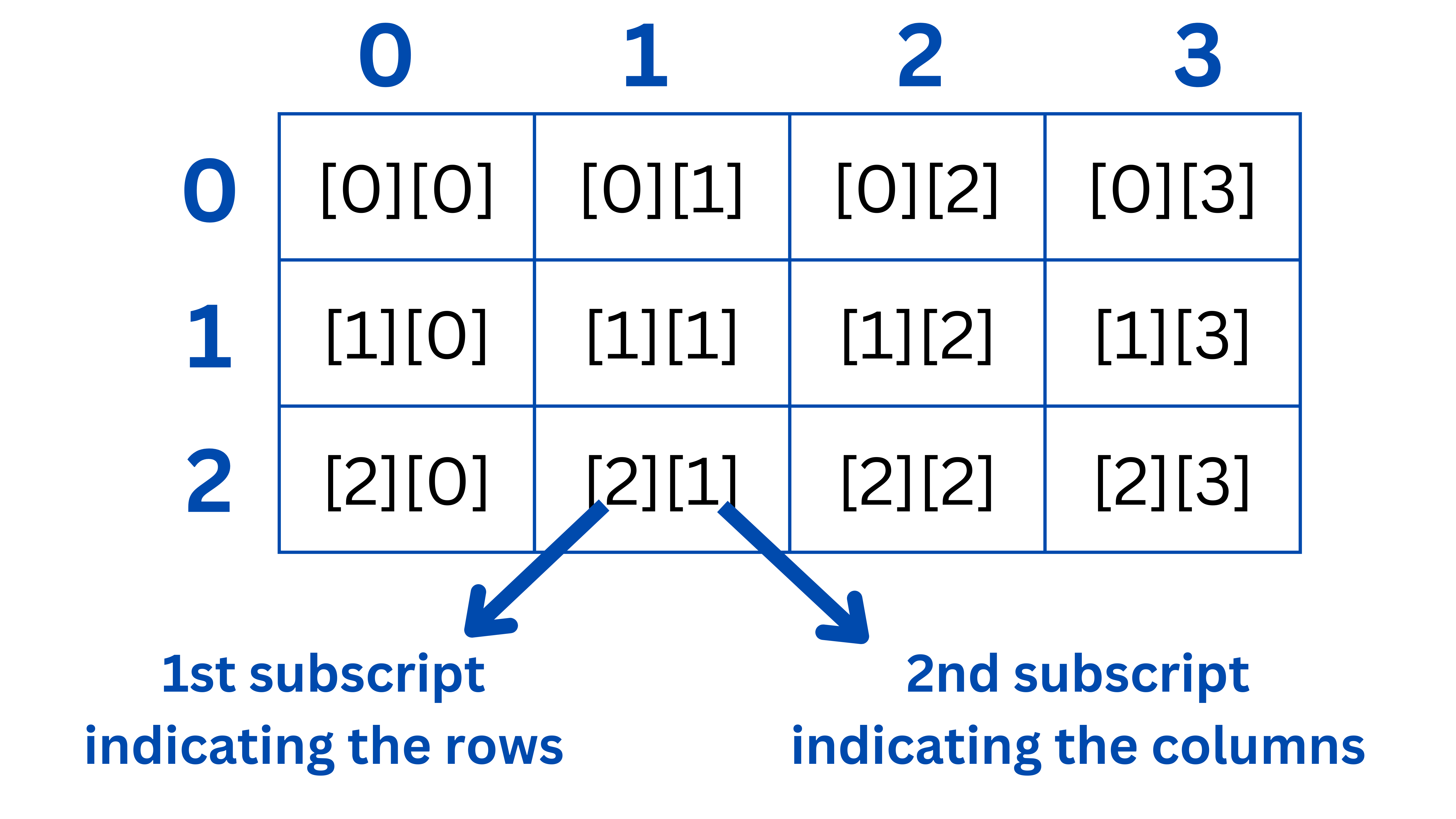
For example: An array inside an array.
// Matrix like structure using arrays
const row1 = [1, 2, 3]
const row2 = [4, 5, 6]
const row3 = [7, 8, 9]
// Nesting of arrays within a array
const matrixOne = [row1, row2, row3]
console.log(matrixOne)
OUTPUT
[[1, 2, 3], [4, 5, 6], [7, 8, 9]]
// Access first list item in matrix array
matrixOne[0]
OUTPUT
[1, 2, 3]
// access first element of first array item in matrix array
matrixOne[0][0]
OUTPUT
1
Basic Array Methods
object.method(parameters)
(1) pop()
pop()
is a mutating method i.e. it changes length of the array.SYNTAX : pop()
// pop() removes last element of an array
const players = ["Sachin", "Dhoni", "Virat", "Zaheer", "Rahul"];
const popped = players.pop(); // removes last element 'Rahul'
console.log(popped)
console.log(players)
OUTPUT
Rahul ['Sachin', 'Dhoni', 'Virat', 'Zaheer']
(2) push()
push()
adds one or more elements to the end of an array.push()
is a mutating method i.e. it changes length of the array.SYNTAX : push(element1, element2,...)
// push() adds new items to the end of an array
// push() method with single 'item' as argument
const capitalCities = ["NewDelhi", "NewYork", "London"]
const arraylength = capitalCities.push("Istanbul")
console.log(arraylength)
console.log(capitalCities)
OUTPUT
4 ['NewDelhi', 'NewYork', 'London', 'Istanbul']
// push() adds new items to the end of an array
// push() method with multiple 'items' as argument
const numbers = [12, 24, 45, 67]
const arraylength = numbers.push(78, 89, 91)
console.log(arraylength)
console.log(numbers)
OUTPUT
7 [12, 24, 45, 67, 78, 89, 91]
(3) shift()
pop()
method has similar behavior to shift()
, but applied to the last element in an array.shift()
is a mutating method i.e. it changes length of the array.shift()
method is often used in condition inside while
loop.SYNTAX : shift()
// shift() removes first element from an array
const players = ["Sachin", "Dhoni", "Virat", "Zaheer", "Rahul"];
const shifted = players.shift(); // removes first element 'Sachin'
console.log(shifted)
console.log(players)
OUTPUT
Sachin ['Dhoni', 'Virat', 'Zaheer', 'Rahul']
// shift() removes first element from an array
// shift() method is used in 'while' loop
const players = ['Messi', 'Neymar', 'Ronaldo', 'Benzema']
// every iteration will remove next element from an array, until it is empty
while (typeof (player = players.shift()) !== "undefined") {
console.log(player)
}
console.log("The 'players' array contains ", players)
OUTPUT
Messi Neymar Ronaldo Benzema The 'players' array contains []
(4) unshift()
unshift()
adds one or more elements to the beginning of an array and returns the new length of an array. SYNTAX : unshift(element1, element2,...)
// unshift() adds one or more elements to beginning of Array
const healthyFood = ['Avocado', 'Kiwi', 'Moringa']
const arrayLen = healthyFood.unshift('Spinach', 'Kale', 'Collard')
console.log(arrayLen)
console.log(healthyFood)
OUTPUT
6 ['Spinach', 'Kale', 'Collard', 'Avocado', 'Kiwi', 'Moringa']
(5) includes()
element
, returning true
or false
as appropriate.fromIndex
will specify from which index position should the search start.SYNTAX : includes(element [, fromIndex])
// includes() returns true if an array contains 'element'
// Example contains one argument : 'element'
const fishes = ['Catfish', 'Bass', 'Carp', 'Tuna', 'Salmon']
let isExists = fishes.includes('Carp') // search for 'Carp'
console.log(isExists)
OUTPUT
true
// includes() returns true if an array contains 'element'
// Example contains two argument : 'element' & 'fromIndex'
const fishes = ['Catfish', 'Bass', 'Carp', 'Tuna', 'Salmon']
// search for 'Tuna' from index position 3
let isExists = fishes.includes('Tuna', 3)
console.log(isExists)
OUTPUT
true
(6) indexOf()
-1
if it is not present.indexOf()
method compares element
to items of the array using strict equality ===
.fromIndex
will specify from which index position should the search start.NaN
values in the array, the indexOf()
method will return -1
.SYNTAX : indexOf(element [, fromIndex])
// indexOf() returns first index of the found element else -1
// Example contains one argument : 'element'
const ranNum = [11, 12, 34 ,76, 11, 98]
let searchIndex = ranNum.indexOf(11)
console.log(searchIndex)
OUTPUT
0
// indexOf() returns first index of the found element else -1
// Example contains two argument : 'element' & 'fromIndex'
const ranNum = [11, 12, 34 ,76, 11, 98]
// search for 11 from index position 3
let searchIndex = ranNum.indexOf(11, 3)
console.log(searchIndex)
OUTPUT
4
(7) concat()
concat()
method does not change the existing arrays, but instead returns a new array.concat()
is a copying method. It does NOT alter any of arrays provided as arguments but instead returns a shallow copy.value
can be an arrays and/or values to concatenate into a new array.SYNTAX : concat(value1, value2,...)
// concat() merge two or more arrays and returns a new array
const symOne = ['INR', 'USD', 'EUR']
const symTwo = ['JPY', 'CNY']
const symThree = symOne.concat(symTwo)
console.log("symThree = ", symThree)
// no change to the original arrays
console.log("symOne = ", symOne)
console.log("symTwo = ", symTwo)
OUTPUT
symThree = ['INR', 'USD', 'EUR', 'JPY', 'CNY'] symOne = ['INR', 'USD', 'EUR'] symTwo = ['JPY', 'CNY']
// concat() merge two or more arrays and returns a new array
// Example to concatenate array1 with array2 and values 7 & 8
const array1 = [2, 4, 6]
const array2 = [1, 3, 5]
// concatenate array1 with array2 and values 7 & 8
const array3 = array1.concat(array2, 7, 8)
console.log("array3 = ", array3)
// no change to the original arrays
console.log("array1 = ", array1)
console.log("array2 = ", array2)
OUTPUT
array3 = [2, 4, 6, 1, 3, 5, 7, 8] array1 = [2, 4, 6] array2 = [1, 3, 5]
// concat() merge two or more arrays and returns a new array
// concat() with no arguments
const fruits = ['apples', 'oranges', 'kiwi']
const newArray = fruits.concat() // creates a shallow copy
console.log("newArray = ", newArray)
OUTPUT
newArray = ['apples', 'oranges', 'kiwi']
(8) join()
separator
string.separator
.separator
specifies a string to separate each pair of adjacent elements of the array. The separator
is converted to a string if necessary. If omitted, the array elements are separated with a comma ,
.SYNTAX : join([separator])
// join() returns a new string by concatenating elements in an array
const shopList = ["Tea", "Milk", "Sugar"]
shopList.join() // 'Tea,Milk,Sugar'
shopList.join(", ") // 'Tea, Milk, Sugar'
shopList.join(" + ") // 'Tea + Milk + Sugar'
shopList.join("") // 'TeaMilkSugar'
// array with one element
['SKILLZAM'].join() // 'SKILLZAM'
(9) reverse()
order
in the array will be turned towards the direction opposite to that previously stated.reverse()
method does not have any arguments.reverse()
is a mutating method i.e. it changes order of the array. reverse()
method returns reference to the original array, so mutating the returned array will mutate the original array as well.reverse()
to NOT mutate the original array, but return a shallow copy array, then before calling reverse()
, using the spread(...)
operator syntax or Array.from()
SYNTAX : reverse()
// reverse() method reverses an array
const colors = ['red', 'green', 'blue', 'orange', 'cyan']
colors.reverse()
console.log(colors)
OUTPUT
['cyan', 'orange', 'blue', 'green', 'red']
// reverse() method reverses an array
// Mutating returned array will mutate original array
const fibonacci = [0, 1, 1, 2, 3, 5, 8, 13, 21, 34, 55, 80]
const newFibo = fibonacci.reverse()
console.log("newFibo = ", newFibo)
// Modify one element of new array 'newFibo'
// original array 'fibonacci' is also modified
newFibo[0] = 89
console.log("newFibo = ", newFibo)
console.log("fibonacci = ", fibonacci)
OUTPUT
newFibo = [80, 55, 34, 21, 13, 8, 5, 3, 2, 1, 1, 0] newFibo = [89, 55, 34, 21, 13, 8, 5, 3, 2, 1, 1, 0] fibonacci = [89, 55, 34, 21, 13, 8, 5, 3, 2, 1, 1, 0]
// reverse() method reverses an array
// NOT mutate the original array
const fibonacci = [0, 1, 1, 2, 3, 5, 8, 13, 21, 34, 55, 80]
// spread(...) operator, will create a shallow-copied array
const newFibo = [...fibonacci].reverse()
console.log("newFibo = ", newFibo)
// Modify one element of new array 'newFibo'
// No change to original array 'fibonacci'
newFibo[0] = 89
console.log("newFibo = ", newFibo)
console.log("fibonacci = ", fibonacci)
OUTPUT
newFibo = [80, 55, 34, 21, 13, 8, 5, 3, 2, 1, 1, 0] newFibo = [89, 55, 34, 21, 13, 8, 5, 3, 2, 1, 1, 0] fibonacci = [0, 1, 1, 2, 3, 5, 8, 13, 21, 34, 55, 80]
(10) slice()
slice()
method returns a shallow copy of a portion of the original array into a new array object.slice()
method will NOT modifiy the original array.start
and end
will specify the starting and ending (end
index not included), index position of the array.start
is ommited, then it will default to 0
value.end
argument is ommitted, then array.length
is used, which means all elements until the end of array, to be extracted.SYNTAX : slice([start], [end])
// slice() returns shallow-copied portion of array
// Single optional argument 'start' is used
const dinosaurs = ['Brachiosaurus', 'Patagosaurus', 'Spinosaurus', 'Tyrannosaurus']
const carniDino = dinosaurs.slice(2)
console.log(carniDino)
OUTPUT
['Spinosaurus', 'Tyrannosaurus']
// slice() returns shallow-copied portion of array
// Both optional argument 'start' & 'end' are used
const dinosaurs = ['Brachiosaurus', 'Patagosaurus', 'Spinosaurus', 'Tyrannosaurus']
const herbiDino = dinosaurs.slice(0,2)
console.log(herbiDino)
OUTPUT
['Brachiosaurus', 'Patagosaurus']
// slice() returns shallow-copied portion of array
// NO optional arguments are used
const dinosaurs = ['Brachiosaurus', 'Patagosaurus', 'Spinosaurus', 'Tyrannosaurus']
const newDino = dinosaurs.slice() // creates a shallow-copied full array
console.log(newDino)
OUTPUT
['Brachiosaurus', 'Patagosaurus', 'Spinosaurus', 'Tyrannosaurus']
(11) splice()
splice()
method is a mutating method.start
argument specifies the starting index position of the array. If start
is omitted, then 0
is used.deleteCount
specifies the number of elements in the array to remove from start
index. If deleteCount
is omitted, then all the elements from start to the end of the array will be deleted. item
specifies the elements to add to the array, beginning from start
. If we do not specify any elements, splice()
will only remove elements from the array.SYNTAX : splice(start [, deleteCount] [, item1, item2... ])
// splice() changes the contents of an array
// Using all three arguments with 0 'deleteCount'
const animals = ['Ape', 'Cow', 'Dog', 'Fox']
animals.splice(1, 0, 'Cat') // Inserts 1 element at index 1
console.log(animals)
OUTPUT
['Ape', 'Cat', 'Cow', 'Dog', 'Fox']
// splice() changes the contents of an array
// Using all three arguments
const animals = ['Ape', 'Cow', 'Dog', 'Fox']
// Replaces 1 element with 1 element at index 3
animals.splice(3, 1, 'Elk')
console.log(animals)
OUTPUT
['Ape', 'Cow', 'Dog', 'Elk']
// splice() changes the contents of an array
// Using all three arguments
const animals = ['Ape', 'Cow', 'Dog', 'Fox']
// Replaces 1 element with 3 elements at index 3
animals.splice(3, 1, 'Elk', 'Kob', 'Yak')
console.log(animals)
OUTPUT
['Ape', 'Cow', 'Dog', 'Elk', 'Kob', 'Yak']
// splice() changes the contents of an array
// Using two arguments 'start' & 'deleteCount'
const animals = ['Ape', 'Cow', 'Dog', 'Fox']
animals.splice(3, 1) // Removes 1 element at index 3
console.log(animals)
OUTPUT
['Ape', 'Cow', 'Dog']
Objects
Object
is a complex datatypes in JavaScript.{ }
and have a comma-separated key : value
pairs (key and value separated by a colon :
).const
keyword. any data type
.For Example: Consider Football Player Leo Messi's Bio
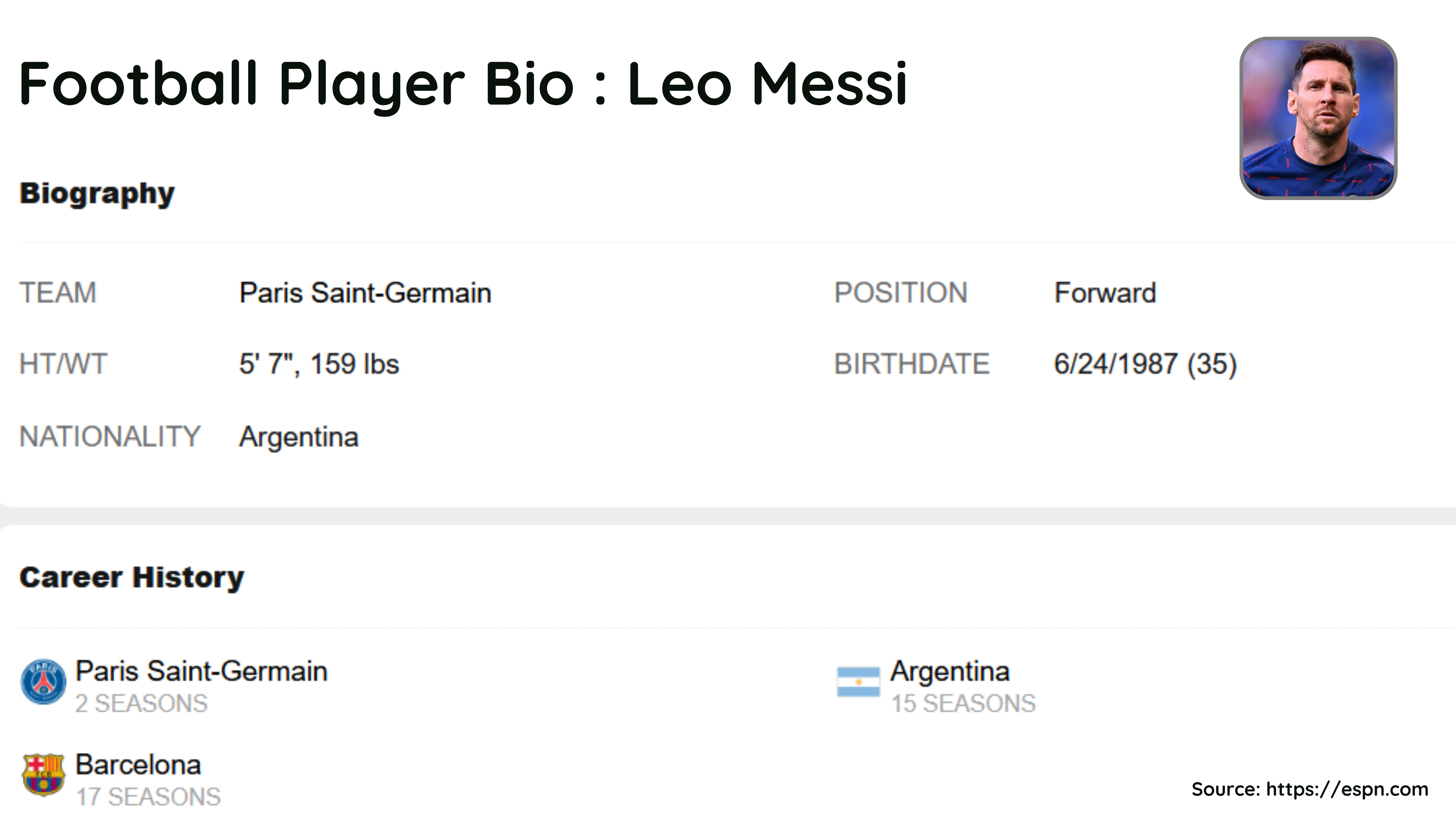
This player bio example can be represented by a object
data structure.
// Object Literal Example:
const playersBio = {
name: "Leo Messi",
team: "Paris Saint-Germain",
position: "Forward",
height: 170,
weight: 159,
birthdate: "24/6/1987",
age: 35,
nationality: "Argentina",
careerHistory: ["Barcelona","PSG","Argentina"],
isRetired: false
}
// Objects do not allow duplicate keys
const numbers = {Keyone:1, Keyone:2, Keythree:3}
console.log(numbers)
OUTPUT
{Keyone: 2, Keythree: 3}
// Object values can be of any dataType
const students = {
fName: "Jasmine",
lName: "Dsouza",
gender: "Female", // value is string DataType
age: 20, // value is number(integer) DataType
isGraduate: true, // value is boolean DataType
cgpa: 8.4, // value is number(decimal) DataType
favSub: ["Physics","Computers"] // value is array
}
console.log(students)
OUTPUT
{fName: 'Jasmine', lName: 'Dsouza', gender: 'Female', age: 20, isGraduate: true, cgpa: 8.4, favSub: ['Physics', 'Computers', 'History']}
Creating Object
key : value
pairs within braces. Example: { uid: 4098, name: 'Ravi Patil' }
new
keyword with in-built Object
constructor function. Example: const cars = new Object()
new
with user-defined constructor function
.Object.create()
to create new objects.Object.assign()
to create new objects. class
to create objects
// Creating Object: 'Object Literal'
const fruitCost = { apples:123, oranges:456} // using key:value pairs
console.log(fruitCost)
OUTPUT
{apples: 123, oranges: 456}
// Creating Object: using new keyword with
// in-built Object constructor function
const car = new Object()
// Add properties to 'car' object
car.year = 2022
car.make = 'Mahindra'
car.model = 'XUV700'
console.log(car)
OUTPUT
{year: 2022, make: 'Mahindra', model: 'XUV700'}
// Creating Object: using new with
// user-defined constructor function
// constructor function with 'this' keyword
function Cricketer (name, score) {
this.fullName = name;
this.runsScored = score;
}
// Create new Object 'playerOne'
const playerOne = new Cricketer('Virat Kohli', 183)
console.log(playerOne)
console.log(playerOne.runsScored)
OUTPUT
Cricketer {fullName: 'Virat Kohli', runsScored: 183} 183
// Creating Object: using Object.assign()
// to create new objects
// Object 'biography'
const biography = {
name: "Cristiano Ronaldo",
team: "Manchester United",
position: "Forward",
height: 187,
weight: 183,
birthdate: "5/2/1985",
age: 37
}
// Object 'playerHist'
const playerHist = {
nationality: "Portugal",
careerHistory: ["ManU","Juventus","Real Madrid"],
isRetired: false
}
// Create new Object 'PlayerBio' using Object.assign()
const PlayerBio = Object.assign({}, biography, playerHist)
console.log(PlayerBio)
console.log(PlayerBio.name)
OUTPUT
{name: 'Cristiano Ronaldo', team: 'Manchester United', position: 'Forward', height: 187, weight: 183, birthdate: "5/2/1985", age: 37, nationality: "Portugal", careerHistory: ["ManU","Juventus","Real Madrid"], isRetired: false } Cristiano Ronaldo
// Creating Object: using class to create objects
// class created with constructor function
class Employee {
constructor(name, location) {
this.fullname = name
this.city = location
}
}
// Create new object
const empOne = new Employee('Fred Silva', 'Rio de Janeiro')
console.log(empOne.fullname)
console.log(empOne.city)
console.log(empOne)
OUTPUT
Fred Silva Rio de Janeiro Employee {fullname: 'Fred Silva', city: 'Rio de Janeiro'}
Working with Object
Almost "everything" is an object in JavaScript. All values, except primitives, are objects.
new
keyword)new
keyword)new
keyword)
Access the properties of an object by referring to its key
, inside square brackets.
// Accessing the items of a Object
// Using key inside square brackets
const students = {
fName: "Jasmine",
lName: "Dsouza",
gender: "Female",
age: 20,
isGraduate: true,
cgpa: 8.4,
favSub: ["Physics","Computers","History"]
}
firstname = students['fName']
favSubject = students['favSub'][0]
console.log(firstname + ' loves ' + favSubject + '!')
OUTPUT
Jasmine loves Physics!
Access the properties of an object by referring to its key
, using dot(.)
.
// Accessing the items of a Object
// Using object name & key seperated by dot(.)
const students = {
fName: "Jasmine",
lName: "Dsouza",
gender: "Female",
age: 20,
isGraduate: true,
cgpa: 8.4,
favSub: ["Physics","Computers","History"]
}
firstname = students.fName
scoreCGPA = students.cgpa
console.log(firstname + ' scored ' + scoreCGPA + '!')
OUTPUT
Jasmine scored 8.4!
Adding new properties of an object by giving it a value.
// Adding new properties to the Object
const vehicle = {
year: 2021,
make: 'Mahindra'
}
// add new porperty - model: 'XUV700'
vehicle.model = "XUV700"
console.log(vehicle)
OUTPUT
{year: 2021, make: 'Mahindra', model: 'XUV700'}
Change/Modify the value of a specific property of an object, by referring to its key name.
// Change value of a specific property of an object
const users = {
fname: 'Guido',
lname: 'van Rossum',
email: 'guido@junkmail.com'
}
// Change 'email' property value
users['email'] = 'guido@example.com'
console.log(users)
OUTPUT
{fname: 'Guido', lname: 'van Rossum', email: 'guido@example.com'}
Computed properties of an object using square brackets []
in an object literal, when creating an object.
// Computed properties of an object
let brand = 'Samsung';
const mobile = {
[brand]: 25000, // 'Samsung' property key is taken from variable 'brand'
year: 2022
}
console.log(mobile)
OUTPUT
{Samsung: 25000, year: 2022}
Deleting a properties of an object by using delete
keyword.
// Deleting a properties from the object
const vehicle = {
year: 2021,
make: 'Mahindra',
model: 'XUV700'
}
delete vehicle.model // same as: delete vehicle['model']
console.log(vehicle)
OUTPUT
{year: 2021, make: 'Mahindra'}
in
Keyword is used to determine, if a specified key
is present in an object. For any a non-existing property, in
operator just returns undefined
.
// Check if a key exists 'in' the object
const users = {
fname: 'Guido',
lname: 'van Rossum',
email: 'guido@example.com'
}
isEmailExists = 'email' in users
console.log(isEmailExists)
OUTPUT
true
Static Methods in Object:
Object.entries()
returns an array containing all of the [key, value]
pairs of a given object's own enumerable string properties.Object.keys()
returns an array containing the key
names of all of the given object's own enumerable string properties.Object.values()
returns an array containing the values
that correspond to all of a given object's own enumerable string properties.
// Object - static methods
// Object.entries(), Object.keys(), Object.values()
const employee = {
empName: "Javid Khan",
designation: "Software Developer",
city: "Paris",
zip: 70123
}
const allItems = Object.entries(employee)
console.log(allItems)
const allKeys = Object.keys(employee)
console.log(allKeys)
const allValues = Object.values(employee)
console.log(allValues)
OUTPUT
[['empName', 'Javid Khan'], ['designation', 'Software Developer'], ['city', 'Paris'], ['zip', 70123] ] ['empName', 'designation', 'city', 'zip'] ['Javid Khan', 'Software Developer', 'Paris', 70123]
Nested Objects
JavaScript data structures support nesting. This means we can have data structures within data structures. For object
, property values in an object can be another object. You can access nested objects using the dot (.)
notation or the bracket []
notation
For example: An object containing another object.
// Nested Object : Example 1
const team = {
player1: {
name: 'Leo Messi',
position: 'Forward'
},
player2: {
name: 'Andres Iniesta',
position: 'Midfield'
},
player3: {
name: 'Xavi Hernandez',
position: 'Midfield'
}
}
team['player1']['name']
OUTPUT
'Leo Messi'
// Nested Object : Example 2
const player1 = {
name: 'Leo Messi',
position: 'Forward'
}
const player2 = {
name: 'Andres Iniesta',
position: 'Midfield'
}
const player3 = {
name: 'Xavi Hernandez',
position: 'Midfield'
}
const team = {
player1 : player1,
player2 : player2,
player3 : player3
}
team['player1']
OUTPUT
{name: 'Leo Messi', position: 'Forward'}
Object Methods
- (1).
hasOwnProperty()
:
method returns a boolean indicating whether the object has the specified property as its own property, as opposed to inheriting it.
The argumentproperty
is theString
name orSymbol
of the property to test.SYNTAX :
hasOwnProperty(property)
- (2).
toString()
:
method returns a string representing the object.
This method is meant to be overridden by derived objects for custom type conversion logic.SYNTAX :
toString()
- (3).
valueOf()
:
method of
Object
converts thethis
value to an object. This method is meant to be overridden by derived objects for custom type conversion logic.SYNTAX :
valueOf()
// hasOwnProperty() returns whether object has property
const player = {
name: 'Leo Messi',
position: 'Forward'
}
player.hasOwnProperty('position') // returns true
OUTPUT
true
// toString() returns string representing the object
function Player(name, position) {
this.name = name
this.position = position
}
const player1 = new Player('Leo Messi', 'Forward')
Player.prototype.toString = function playerToString() {
return `${this.name} plays as ${this.position}`;
}
console.log(player1.toString())
OUTPUT
Leo Messi plays as Forward
// valueOf() methods
// Example : Area of a circle
function SquareRad(num) {
this.square = num * num
}
SquareRad.prototype.valueOf = function() {
return this.square;
}
const radiusObj = new SquareRad(5)
console.log("Area of circle = " + 3.142 * radiusObj )
OUTPUT
Area of circle = 78.55
Control Flow in JavaScript
- [1]. Decision Making statements (
if
,else if
,else
,switch
) - [2]. Loop statements (
for
,for...in
,for...of
,while
,do...while
) - [3]. Jump statements (
break
,continue
)
Decision making statements
if-else
statements, allow the programmer to execute certain pieces of code depending on some Boolean condition.- [1].
if
statement - [2].
else if
statement - [3].
else
statement - [4].
switch
statement
if
, else if
and else
statements. In these conditional clauses, else if
and else
blocks are optional; additionally, you can optinally include as few or as many else if
statements as you would like.Simple if
statement
if
statement is the most simple decision-making statement.if
keyword.true
then a block of statement is executed otherwise not. true
or false
{}
) to define scope in the code. Other programming language like Python, often use "indentation" for this purpose.Example of Simple if
statement:
// Simple 'if' statement
let num1 = 24,
num2 = 12;
// 'if' condition is true, hence the block will be executed
if (num1 > num2) {
console.log(`num1(${num1}) is greater than num2(${num2})`)
}
// if condition is false, hence "if" block will NOT be executed
if (num2 > num1) {
console.log(`num2(${num2}) is greater than num1(${num1})`)
}
OUTPUT
num1(24) is greater than num2(12)
if...else
statement
if
statement alone tells us that, if a condition is true
, it will execute a block of statements and if the condition is false
it won't.false
. Here comes the else
statement.else
statement with if
statement to execute a block of code when the condition is false
.
// 'if...else' statement
let num1 = 36,
num2 = 48;
// if condition is false, hence "if" block will NOT be executed
// hence, else block will be executed
if (num1 > num2) {
console.log(`num1(${num1}) is greater than num2(${num2})`)
}
else {
console.log(`num1(${num1}) is lesser than num2(${num2})`)
}
OUTPUT
num1(36) is lesser than num2(48)
Nested if
statement
if
is an if
statement that is the target of another if
or else
.if
statements mean an if
statement inside an if
statement.if
statements within if
statements. i.e, we can place an if
statement inside another if
statement.
// Nested "if/else" statement
let ranNum = 28
// if condition is true, hence "if" block will be executed
if (ranNum == 28 || ranNum <= 30) {
// if condition is true, hence nested "if" block will be executed
if (ranNum < 30) {
console.log('ranNum is smaller than 30')
}
// if condition is false, hence nested "if" block will NOT be executed
if (ranNum < 15) {
console.log('ranNum is smaller than 15')
}
}
// never executes else block
else {
console.log('ranNum is larger than 30')
}
OUTPUT
ranNum is smaller than 30
if
...else if
...else
ladder statement
if
statements are executed from the top down. if
is true
, the statement associated with that if
is executed, and the rest of the ladder is bypassed. true
, then the final else
statement will be executed.
// if...else if...else ladder statement
let givenNum = 100
// if condition is false, hence "if" block will NOT be executed
if (givenNum == 25) {
console.log('givenNum is 25')
}
// if condition is false, hence "else if" block will NOT be executed
else if (givenNum == 50) {
console.log('givenNum is 50')
}
// if condition is false, hence "else if" block will NOT be executed
else if (givenNum == 75) {
console.log('givenNum is 75')
}
// if condition is true, hence "else if" block will be executed
else if (givenNum == 100) {
console.log('givenNum is 100')
}
// never executes else block
else {
console.log('givenNum is INVALID')
}
OUTPUT
givenNum is 100
switch
statement
switch
statement is to be used, when one of many code blocks is to be executed.switch
statement evaluates an expression, matching the expression's value against a series of case
clauses.case
clause with a matching value (using the strict equality comparison), until a break
statement is encountered. default
clause of a switch
statement will be executed, if no case
matches the expression's value.
// switch statement
// Example: Based on billing rate, decide salay of employee
const billRate = 40
switch (billRate) {
case 25:
console.log("Salary paid per month = 80000");
break;
case 40:
case 45:
console.log("Salary paid per month = 125000");
break;
case 60:
console.log("Salary paid per month = 190000");
break;
case 90:
console.log("Salary paid per month = 300000");
break;
default:
console.log("He/She is unbillable resource.");
}
OUTPUT
Salary paid per month = 125000
Shorthand if
statement
if
statement, without curly brackets.
// Shorthand "if" statement
let weightOne = 225,
weightTwo = 125;
if (weightOne > weightTwo) console.log("weightOne is heavier")
console.log("***End of Code***")
OUTPUT
weightOne is heavier ***End of Code***
Shorthand if...else
or "Ternary" operator
if
, and one for else
, you can put it all on the same line, without curly brackets.
// Shorthand "if...else" statement
// “Question mark” or "Ternary" operator
let num1 = 144,
num2 = 169;
// (condition) ? (If 'true') : (If 'false')
(num1 > num2) ? console.log("num1 is largest") : console.log("num2 is largest")
OUTPUT
num2 is largest
Loops in JavaScript
start
and end
points of the loop.- In JavaScript, we have different kind of looping statements:
- (1).
while
loops through a block of code while a specified condition is true - (2).
do while
also loops through a block of code while a specified condition is true - (3).
for
loops through a block of code a number of times - (4).
for...of
loops through the values of an iterable object - (5).
for...in
loops through the properties of an object
[1]. while
Loop
while
loop is used to execute a block of statements repeatedly until a given condition is satisfied (true
).false
, the line immediately after the loop in the program is executed.while
loop falls under the category of indefinite iteration. Indefinite iteration means that the number of times the loop is executed isn't specified explicitly in advance.while
loop is executed, expression is first evaluated in a Boolean context and if it is true
, the loop body is executed. Then the expression is checked again, if it is still true
then the body is executed again and this continues until the expression becomes false
.while
loop is a while
loop inside a while
loop.
// while loop to print numbers : 1 to 5
let i = 1
while (i < 6) {
console.log(i)
i += 1 // remember to increment i, or else loop will continue forever
}
OUTPUT
0 1 2 3 4 5
// Nested "while" loop
let j = 0,
i = 1,
str = '';
while (i <= 5) {
j = 1
while (j <= i) {
str += (j + ' ')
j += 1
}
str += "\n";
i += 1
}
console.log(str)
OUTPUT
1 1 2 1 2 3 1 2 3 4 1 2 3 4 5
[2]. do while
Loop
do while
also loops through a block of code while a specified condition is true
.while
loop, the do while
loop always executes the statement at least once before evaluating the expression.do while
loop :
Block of code
is always executed once before the condition is checked.- If
condition
istrue
, the statement executes again. At the end of every execution, the condition is checked. When the condition isfalse
, execution stops, and control passes to the statement followingdo while
.
SYNTAX:
do {
// Block of code to be executed
}
while (condition);
// do while loop Example
let counter = 1
do {
console.log(counter)
counter++
} while (counter <= 3)
OUTPUT
1 2 3
[3]. for
Loop
for
loop repeats until a specified condition evaluates to false
. The JavaScript for
loop is similar to the Java and C for
loop.for
loop is a for
loop inside a for
loop.for
loop :
expression1
= initial Expression Eg: let i = 0;expression2
= Condition Eg: i <= 10;expression3
= increment Expression Eg: i++
SYNTAX:
for (expression1; expression2; expression3) {
// Block of code to be executed
}
- When a
- (1). Initializing expression
expression1
, if any, is executed. This expression usually initializes one or more loop counters, but the syntax allows an expression of any degree of complexity. This expression can also declare variables. - (2). Condition expression
expression2
is evaluated. If the value of condition is true, the loop statements execute. Otherwise, the for loop terminates. (If the condition expression is omitted entirely, the condition is assumed to betrue
.) - (3). Block of code within curly braces
{}
executes multiple statements. - (4). Increment expression
expression3
, if any, is executed. - (5). Control returns to Step (2) i.e. condition expression
expression2
is evaluated.
for
loop executes, the following ordered steps occurs:
// for loop
// Example : Adding all single digit numbers as elements of an array
const numArray = [] // create empty array
let singleDigit,
index = 0;
for (singleDigit = 0; singleDigit <= 9; singleDigit += 1) {
numArray[index] = singleDigit
index++
}
console.log(`The "numArray" contains = [${numArray}]`)
/****************************************************/
// Tracing 'for' loop in the above example
//
// 1st: singleDigit=0; true; o/p: 0 added to the array
// 2nd: singleDigit=1; true; o/p: 1 added to the array
// 3rd: singleDigit=2; true; o/p: 2 added to the array
// ....
// .... continue adding to the array
// ....
// 9th: singleDigit=8; true; o/p: 8 added to the array
// 10th:singleDigit=9; true: o/p: 9 added to the array
// 11th:singleDigit=10; false; exit the for loop
//
// The "numArray" contains = [0,1,2,3,4,5,6,7,8,9]
/*****************************************************/
OUTPUT
The "numArray" contains = [0,1,2,3,4,5,6,7,8,9]
// for loop
// Example : Find sum of all number in an array
let evenNum = [22, 44, 66]; // array length = 3
let sumNum = 0;
for (let x = 0; x < evenNum.length; x++) {
sumNum = sumNum + evenNum[x];
}
console.log(`Sum of all the number in an array = ${sumNum}`)
/*****************************************************/
// Tracing 'for' loop in the above example
//
// 1st: x = 0; true; sumNum = 0 + 22 = 22
// 2nd: x = 1; true; sumNum = 22 + 44 = 66
// 3rd: x = 2; true; sumNum = 66 + 66 = 132
// 4th: x = 3; false; Exit the 'for' loop
//
// Sum of all the number in an array = 132
/*****************************************************/
OUTPUT
Sum of all the number in an array = 132
// Nested "for" loop example
for (let p = 1; p <= 2; p++) { //p: 1,2
console.log(`p is: ${p}`)
for (let q = 1; q < 4; q++) { //q: 1,2,3
console.log(` q is: ${q}`)
}
}
/*****************************************************/
// Tracing Nested 'for' loop in the above example
//
// Outer for loop - 1st of p: p = 1; true; o/p: p is:1
// 1st of q: q = 1; true; o/p: q is:1
// 2nd of q: q = 2; true; o/p: q is:2
// 3rd of q: q = 3; true; o/p: q is:3
// 4th of q: q = 4; false; Exit the inner q loop
// Outer for loop - 2nd of p: p = 2; true; o/p: p is:2
// 1st of q: q = 1; true; o/p: q is:1
// 2nd of q: q = 2; true; o/p: q is:2
// 3rd of q: q = 3; true; o/p: q is:3
// 4th of q: q = 4; false; Exit the inner q loop
// Outer for loop - 3rd of p: p = 3; false; Exit the outer p loop
//
/*****************************************************/
OUTPUT
p is: 1 q is: 1 q is: 2 q is: 3 p is: 2 q is: 1 q is: 2 q is: 3
// "for" loop : Iterate multi-dimension array using "for" loop
// Example : Print the country name and Flag colors
const arrFlags = [
["INDIA","Orange","White","Green"],
["GERMANY","Black","Red","Yellow"],
["RUSSIA","White","Red","Blue"],
["COLOMBIA","Yellow","Blue","Red"],
["EGYPT","Red","White","Black"]
]
for (let a = 0; a < arrFlags.length; a++) { // arrFlags.length = 5
let flagRow = arrFlags[a];
console.log(flagRow[0] + " Flag Colors");
for (let b = 1; b < flagRow.length ; b++) { // flagRow.length = 4
console.log(flagRow[b]);
}
}
/*****************************************************/
// Tracing nested 'for' loop in the above example
//
// Outer for loop - 1st of a: a = 0; true; flagRow = ["INDIA","Orange","White","Green"] ; O/P: INDIA Flag Colors
// 1st of b: b = 1; true : O/P: Orange
// 2nd of b: b = 2; true : O/P: White
// 3rd of b: b = 3; true : O/P: Green
// 4th of b: b = 4; false Exit the inner b loop
// Outer for loop - 2nd of a: a = 1; true; flagRow = ["GERMANY","Black","Red","Yellow"] ; O/P: GERMANY Flag Colors
// 1st of b: b = 1; true : O/P: Black
// 2nd of b: b = 2; true : O/P: Red
// 3rd of b: b = 3; true : O/P: Yellow
// 4th of b: b = 4; false Exit the inner b loop
// ... continue
//
/*****************************************************/
OUTPUT
INDIA Flag Colors Orange White Green GERMANY Flag Colors Black Red Yellow RUSSIA Flag Colors White Red Blue COLOMBIA Flag Colors Yellow Blue Red EGYPT Flag Colors Red White Black
[4]. for...of
Loop
for...of
statement loops through the values of an iterable objects such as arrays, strings, maps, NodeLists etc. for...of
loop :
variable
: For every iteration the value of the next property is assigned to thevariable
. Variable can be declared withconst
,let
, orvar
.iterable
: An object that has iterable properties.- Block of code within curly braces
{}
executes multiple statements.
SYNTAX:
for (variable of iterable) {
// Block of code to be executed
}
// "for .. of" loop iterating arrays
// Example : Sum of all the number in an array
let total = 0;
let arrayNum = [10, 20, 30, 40]
for (let n of arrayNum) {
total = total + n;
}
console.log(`Sum of all the number in an array = ${total}`)
/*****************************************************/
// Tracing 'for...of' loop in the above example
//
// 1st : n = 10; total = 0 + 10 = 10
// 2nd : n = 20; total = 10 + 20 = 30
// 3rd : n = 30; total = 30 + 30 = 60
// 4th : n = 40; total = 60 + 40 = 100
// No more values in the array, hence exit the for loop
//
// Sum of all the number in an array = 100
/*****************************************************/
OUTPUT
Sum of all the number in an array = 100
// "for .. of" loop iterating strings
// Example : convert string into an array of characters
const charArray = []
let charIndex = 0
for (let singleChar of "SKILLZAM") {
charArray[charIndex] = singleChar;
charIndex++;
}
console.log(`The "charArray" contains = [${charArray}]`)
/******************************************************/
// Tracing 'for...of' loop in the above example
//
// 1st: singleChar = S; o/p: S is added to the array
// 2nd: singleChar = K; o/p: K is added to the array
// 3rd: singleChar = I; o/p: I is added to the array
// 4th: singleChar = L; o/p: L is added to the array
// 5th: singleChar = L; o/p: L is added to the array
// 6th: singleChar = Z; o/p: Z is added to the array
// 7th: singleChar = A; o/p: A is added to the array
// 8th: singleChar = M; o/p: M is added to the array
// No more charaters in the string "SKILLZAM",
// hence exit the for loop
//
// The "charArray" contains = [S,K,I,L,L,Z,A,M]
/*****************************************************/
OUTPUT
The "charArray" contains = [S,K,I,L,L,Z,A,M]
// "for .. of" loop iterating Object
// Example : Iterate values in Object - turn data into an array
let gTotal = 0
const goalScores = {
Messi: 44,
Ronaldo: 43,
Diogo: 43,
Robert: 39,
Turpel: 37,
Suarez: 36,
Salah: 35,
Griezmann: 35,
Cifuente: 34,
Kane: 33
}
// Using Object constructor, create array of 'values'
let goals = Object.values(goalScores)
// goals = [44,43,43,39,37,36,35,35,34,33]
for (let goal of goals) {
gTotal += goal;
}
console.log(`The array of object values is = [${goals}]`)
console.log(`Total goals scored by top 10 players in the year 2018: ${gTotal}`)
OUTPUT
The array of object values is = [44,43,43,39,37,36,35,35,34,33] Total goals scored by top 10 players in the year 2018: 379
[5]. for...in
Loop
for...in
statement loops through the properties of an Object.for...of
loop and a for...in
loop is, while for...in
iterates over property names, for...of
iterates over property values.for...in
over an Array. It is better to use a for
loop, a for...of
loop, or Array.forEach()
when the order is important.for...in
loop :
key
: the key from thekey : value
pair of an object.object
: is a complex datatypes containing named values called properties.- Block of code within curly braces
{}
executes multiple statements.
SYNTAX:
for (key in object) {
// Block of code to be executed
}
// "for .. in" loop
// Example : Use "for .. in" loop to iterate Object literals
let str = ""
const car = {
year: 2022,
make: 'Mahindra',
model: 'XUV700'
}
for (let item in car) {
str += car[item]
str += ' '
}
console.log(str)
OUTPUT
2022 Mahindra XUV700
// "for .. in" loop
// Example : Use "for .. in" loop to iterate Object literals
const bioMessi = {
name: "Leo Messi",
team: "PSG",
position: "Forward",
height: 170,
weight: 159,
birthdate: "24/6/1987",
age: 35,
country: "Argentina",
careerHist: ["PSG","FCB","Argentina"],
isRetired: false
}
for (let bio in bioMessi) {
console.log(`${bio.toUpperCase()} is ${bioMessi[bio]}`);
}
/**************************************************************************************************/
// Tracing for loop
//
// 1st: bio = name; bioMessi[bio] = Leo Messi; o/p: NAME is Leo Messi
// 2nd: bio = team; bioMessi[bio] = PSG; o/p: TEAM is PSG
// 3rd: bio = position; bioMessi[bio] = Forward; o/p: POSITION is Forward
// 4th: bio = height; bioMessi[bio] = 170; o/p: HEIGHT is 170
// 5th: bio = weight; bioMessi[bio] = 159; o/p: WEIGHT is 159
// 6th: bio = birthdate; bioMessi[bio] = 24/6/1987; o/p: BIRTHDATE is 24/6/1987
// 7th: bio = age; bioMessi[bio] = 35; o/p: AGE is 35
// 8th: bio = country; bioMessi[bio] = Argentina; o/p: COUNTRY is Argentina
// 9th: bio = careerHist; bioMessi[bio] = PSG,FCB,Argentina; o/p: CAREERHIST is PSG,FCB,Argentina
// 10th: bio = isRetired; bioMessi[bio] = false; o/p: ISRETIRED is false
// No more name:value pair exists in the object literal, hence exit the for loop
//
/**************************************************************************************************/
OUTPUT
NAME is Leo Messi TEAM is PSG POSITION is Forward HEIGHT is 170 WEIGHT is 159 BIRTHDATE is 24/6/1987 AGE is 35 COUNTRY is Argentina CAREERHIST is PSG,Barcelona,Argentina ISRETIRED is false
Jump statements : break & continue
Jump statements are the loop control statements that change the execution from its normal sequence.
Examples:
break
statementcontinue
statementbreak
statement
break
statement breaks-out of the loop entirely. switch
statement as well.break
as needed is great for situations when a loop's condition must be checked not in the beginning or end of the loop, but in the middle or even in several places of its body.break
statement, we can stop the for
or for...of
or for...in
loop before it has looped through all the items.break
statement, we can stop the while
or do while
loop even if the condition is true
.Example of using break
statement for a less trivial task. This loop will fill a list with all Fibonacci numbers up to a certain value:
// "break" statement for printing Fibonacci numbers
let a = 0,
b = 1,
n,
maxNum = 100,
index = 0;
const listFibo = [];
while (true) {
listFibo[index] = a;
index++;
n = a + b;
a = b;
b = n;
if (a > maxNum) {
break; // usuage of break statement to exit the loop
}
}
console.log(listFibo)
OUTPUT
[0, 1, 1, 2, 3, 5, 8, 13, 21, 34, 55, 89]
continue
statement
continue
statement skips the remainder of the current loop, and goes to the next iteration.continue
statement breaks one iteration (in the loop).continue
statement we can stop the current iteration of the for
or while
loop, and continue with the next.Example of using continue
to print a string of odd numbers. In this case, the result could be accomplished just as well with an if...else
statement, but sometimes the continue
statement can be a more convenient way to express the idea you have in mind:
// "continue" statement for printing ODD numbers
const numArray = [1,2,3,4,5,6,7,8,9,10]
let str = "";
for (let num in numArray) {
// if the remainder of num / 2 is 0, skip the rest of current loop
if (num % 2 == 0) {
continue;
}
str += num + ' '
}
console.log(str)
OUTPUT
1 3 5 7 9
label
statement
label
provides a statement with an identifier that lets you refer to it elsewhere in your program. label
JavaScript statements you precede the statements with a label
name and a colon :
label
reference, the break
statement can be used to jump out of any code block.label
names can not be a reserved words. SYNTAX:
label:
statements
// label statement Example
let total = 0,
i = 1;
whileloop1: while (true) {
i = 1;
whileloop2: while (i < 3) {
total += i;
if (total > 3) {
break whileloop1; // break using 'label' statement
}
console.log("total = " + total);
i++;
}
}
OUTPUT
total = 1 total = 3
Functions in JavaScript
function
is a block of organized, reusable code that is used to perform a single, related action. Functions provide better modularity for your application and a high degree of code reusing.function
.function
will allow you to call the same block of code without having to write it multiple times. This in turn will allow you to create more complex scripts.function
, you must define it somewhere in the scope from which you wish to call it.Function definition
To create a function, we can use a function definition/declaration.
A function definition (also called function declaration, or function statement ) consists of :
function
keyword, followed by name
of the function.parameters
to the function, enclosed in parentheses ()
and separated by commas ,
{}
For example, the following code defines a simple function named createFullName :
// Function definition or declaration Example
function createFullName() {
let fname = "Brendan",
lname = "Eich",
fullname = fname + " " + lname;
console.log(fullname)
}
Function invoking
Defining a function
does not execute it. Defining it names the function
and specifies what to do when the function is called.
function
, you use its name
followed by arguments enclosing in parentheses ()
function
, JavaScript executes the code inside the function body (Block of code within curly brackets {}
.function
can call itself. It is said to be recursive function.funcName()
and window.funcName()
is the same function.
// Function definition/invoking Example
// function definition or declaration
function createFullName() {
let fname = "Brendan",
lname = "Eich",
fullname = fname + " " + lname;
console.log(fullname)
}
// function invoking / calling
createFullName()
OUTPUT
Brendan Eich
Function Arguments & Parameters
()
in the function definition.function
name, inside the parentheses ()
. You can add as many arguments/parameters as you want, just separate them with a comma ,
return
statementWhat is the difference between return
and console.log()
?
The return
keyword allows you to actually save the result of the output of a function as a variable.
The console.log()
function simply displays the output to web console, but doesn't save it for future use. console.log()
doesn't return any value, as it returns undefined
// function Parameters & Arguments
// Two Parameters (fname & lname) used in function declaration
function createFullName(fname, lname) {
let fullname = fname + " " + lname
return fullname // function returns a value
}
let firstName = "Brendan",
lastName = "Eich";
// Two Arguments used in function invoking
let funcReturn = createFullName(firstName, lastName)
console.log(funcReturn)
OUTPUT
Brendan Eich
Default parameters
// Default function Parameter values
function playerClub(club = "no one") {
console.log(`I play for ${club}.`)
}
playerClub("Barcelona")
playerClub() // default parameter is set
playerClub("Al-Nassr")
OUTPUT
I play for Barcelona. I play for no one. I play for Al-Nassr.
- [a]. giving only the mandatory argument:
ask_ok('Enter the capital city: ')
- [b]. giving one of the optional arguments:
ask_ok('Enter the capital city: ', 2)
- [c]. or even giving all arguments:
ask_ok('Enter the capital city: ', 2, 'Just asked to enter city name!')
// Default function Parameter values
function ask_ok(place, retries=3, reminder='Please try again!') {
while (true) {
let city = prompt(place);
if (city === 'Bengaluru' || city === 'Hyderabad' || city === 'Chennai') {
return true
}
if (city === 'Kanpur' || city === 'Surat') {
return false
}
retries = retries - 1;
if (retries < 0) {
console.log('invalid user response')
}
console.log(reminder)
}
}
// function invoking using only mandatory argument
ask_ok('Enter the capital city: ')
OUTPUT
Enter the capital city: Hyderabad true
Recursion Function
factorial()
is a function that we have defined to call itself ("recurse"). Suppose we want to find the factorial of 5, then it will goes as below:
0! = 1
1! = 1 x 0! = 1 x 1 = 1
2! = 2 x 1! = 2 x 1 = 2
3! = 3 x 2! = 3 x 2 = 6
4! = 4 x 3! = 4 x 6 = 24
5! = 5 x 4! = 5 x 24 = 120
// Function recursion example
function factorial(num) {
let result = 0;
if (num === 1) {
return 1;
} else {
result = num * factorial(num-1);
return result;
}
}
let randNum = 5,
funcRtn = factorial(randNum);
console.log(`The factorial of ${randNum} is ${funcRtn}`)
OUTPUT
The factorial of 5 is 120
Nested Function
function
within another function.
// Nested Function Example
function indiaWorldCup() {
const runScored = [317,350,322,301];
function announceScores() {
let matchNum = 1;
function scoreBoard() {
for (let run of runScored) {
console.log(`${matchNum} : Team India scored ${run} runs.`);
matchNum++;
}
}
scoreBoard();
}
announceScores();
}
indiaWorldCup();
OUTPUT
1 : Team India scored 317 runs. 2 : Team India scored 350 runs. 3 : Team India scored 322 runs. 4 : Team India scored 301 runs.
JavaScript Examples
JavaScript Notes contains many examples for your understanding. With our online editor, you can edit and test each example yourself.
Description of Example | Links |
---|---|
Display - Hello World | Code |
Add internal JS to HTML | Code |
Add inline JS to HTML | Code |
Variables : let, var & const | Code |
Data Types | Code |
typeof Operator | Code |
Aritmetic Operators | Code |
Assignment Operators | Code |
Comparison Operators | Code |
Logical Operators | Code |
Conditional Operator | Code |
Decision Making | Code |
Truthy & Falsy | Code |
Arrays | Code |
Array Methods - Part -1 | Code |
Objects | Code |
for Loop | Code |
while Loop | Code |
Function declaration & invoking | Code |
Function parameters & arguments | Code |
Function Block & Lexical scope | Code |
Functions : Object Methods | Code |
Function with try & Catch | Code |
Function Expression | Code |
Arrow Function Expression | Code |
Nested & Callback Functions | Code |
Default Params | Code |
Array Methods - Part -2 | Code |
spread Operator | Code |
Destructuring | Code |
JavaScript Interview Questions
Get the hold of actual interview questions during job hiring.
What is JavaScript, and how is it used in web development?
JavaScript is a programming language that is used primarily to add interactivity and dynamic behavior to websites. JavaScript code can be embedded directly into HTML web pages or included in external script files, and it can be used to manipulate HTML and CSS, handle user input, and interact with web servers through APIs. JavaScript is a crucial component of modern web development, and it is used extensively in frameworks and libraries such as React, Angular, and Vue.
What is the difference between null and undefined in JavaScript?
null and undefined are both used to represent absence of a value, but they have slightly different meanings. Undefined is a value that is assigned to a variable that has not been initialized, or to a function parameter that has not been passed a value. Null, on the other hand, is a value that is explicitly assigned to a variable or object property to represent the absence of a value. In practice, null is often used as a default value when an object property is expected to be set later, while undefined is typically used to represent a programming error or oversight.
What is hoisting in JavaScript, and how does it work?
Hoisting in JavaScript is a feature that allows variables and functions to be declared after they are used in a program. This is possible because JavaScript uses two passes to interpret code: the first pass scans the code for variable and function declarations and "hoists" them to the top of their respective scopes, and the second pass executes the code. This means that a variable or function can be used before it is declared, as long as it is declared somewhere in the same scope. However, hoisted variables and functions are not initialized until their declaration statements are reached, so they may have the value "undefined" until they are explicitly assigned a value.
What are the differences between JavaScript and other programming languages like Java and Python?
There are several differences between JavaScript and other programming languages like Java and Python:
What are arrow functions in JavaScript?
Arrow functions are a concise way to define functions in JavaScript. They were introduced in ECMAScript 6 and provide a shorter syntax for defining functions compared to traditional function declarations. Arrow functions are also automatically bound to the scope of their parent function or the global scope, depending on how they are defined.
What are the different types of events in JavaScript?
There are many types of events in JavaScript, including mouse events (such as click, mouseover, and mouseout), keyboard events (such as keypress and keydown), form events (such as submit and change), and document and window events (such as load and resize).
What is the purpose of the async/await keywords in JavaScript?
The async/await keywords were introduced in ECMAScript 7 as a way to simplify asynchronous programming in JavaScript. Async/await allows developers to write asynchronous code that looks and behaves like synchronous code, making it easier to reason about and debug. Async/await works by allowing developers to mark a function as asynchronous using the "async" keyword, and then use the "await" keyword to wait for a Promise to resolve before continuing with the execution of the code.
What is the difference between a primitive data type and an object data type in JavaScript?
A primitive data type is a value that is not an object and has no methods. Examples of primitive data types in JavaScript include numbers, strings, booleans, null, and undefined. An object data type, on the other hand, is a complex data type that can contain properties and methods. Examples of object data types in JavaScript include arrays, functions, and objects.
What is a callback function in JavaScript?
A callback function is a function that is passed as an argument to another function and is then executed when the parent function completes. Callback functions are commonly used in JavaScript for asynchronous programming tasks, such as handling events or making API calls.
What is the event loop in JavaScript, and how does it work?
The event loop in JavaScript is a mechanism that allows for asynchronous execution of code in a single-threaded environment. When an asynchronous operation is initiated, such as a network request or a timer, the operation is placed in a queue and the program continues to execute. When the operation is completed, a callback function is added to another queue. The event loop constantly checks the callback queue and executes any functions that are waiting, in the order they were added. This allows JavaScript to handle multiple asynchronous operations simultaneously, without blocking the main thread.
What is closure in JavaScript, and how is it used?
A closure in JavaScript is a function that has access to variables and functions defined in its outer scope, even after the outer function has returned. Closures are created when a function returns another function that references variables in its parent scope. This allows the inner function to access and modify the parent scope's variables, even though the parent function has already completed execution. Closures are often used to create private variables and functions in JavaScript, or to implement higher-order functions that return functions with customized behavior.
What is the difference between let, const, and var in JavaScript?
let and const are block-scoped declarations, while var is function-scoped. Variables declared with let and const cannot be redeclared in the same block, while var allows for redeclaration. Additionally, variables declared with const cannot be reassigned a new value, while let and var can be. Let and const are relatively new features of JavaScript that were introduced in ES6, while var has been part of the language since its inception.
What is the difference between == and === in JavaScript?
The double equals (==) operator in JavaScript compares two values for equality, allowing for type coercion if necessary. For example, the expression "5" == 5 would evaluate to true, because the string "5" is coerced into the number 5 for comparison. The triple equals (===) operator, on the other hand, compares two values for equality without type coercion, so the expression "5" === 5 would evaluate to false, because the types are different.
What are the different data types in JavaScript?
JavaScript has several primitive data types, including number, string, boolean, null, undefined, bigint and symbol. Additionally, JavaScript has a complex data type called object, which can store collections of key-value pairs and functions. Arrays are a special type of object that can store collections of values, and functions are a type of object that can be called like a regular function.
What is a promise in JavaScript, and how does it work?
A promise in JavaScript is an object that represents a value that may not be available yet, but will be resolved at some point in the future. Promises are used to handle asynchronous operations, such as network requests or database queries, and allow the program to continue executing while the operation is in progress. Promises have three states: pending, fulfilled, and rejected. When a promise is fulfilled, it means that the value is available and the promise's then() method is called with the value as an argument. When a promise is rejected, it means that an error occurred and the promise's catch() method is called with the error as an argument.
What is the difference between call and apply in JavaScript?
Both call() and apply() are methods in JavaScript that allow a function to be called with a specific value for the "this" keyword, and with arguments passed in as an array-like object. The main difference between call() and apply() is in how the arguments are passed in. With call(), the arguments are passed in as a comma-separated list, while with apply(), the arguments are passed in as an array. This means that apply() is useful when the number of arguments is not known ahead of time, or when the arguments are already in an array-like object.
How do you declare a variable in JavaScript?
You can declare a variable in JavaScript using the var, let, or const keyword, like this:
var greet = 'Hello, world!';
let num = 123;
const PI = 3.14;
What is the difference between a closure and a callback in JavaScript?
A closure in JavaScript is a function that has access to variables and functions defined in its outer scope, even after the outer function has returned. A callback function, on the other hand, is a function that is passed as an argument to another function and is called at a later time, usually after some asynchronous operation has completed. While both closures and callbacks are used to handle asynchronous operations in JavaScript, closures are used to maintain access to variables and functions in the outer scope, while callbacks are used to execute a function after an operation has completed.
What is the difference between let and var in JavaScript?
The main difference between let and var in JavaScript is in their scoping. Variables declared with let are block-scoped, meaning they are only accessible within the block in which they are declared. Variables declared with var, on the other hand, are function-scoped, meaning they are accessible throughout the entire function in which they are declared. Additionally, variables declared with let cannot be redeclared in the same block, while var allows for redeclaration.
What is the prototype in JavaScript?
In JavaScript, every object has a prototype property, which is a reference to another object. This prototype object contains methods and properties that are inherited by the object.
When a property or method is accessed on an object, JavaScript first looks for that property or method on the object itself. If the property or method is not found on the object, JavaScript then looks for it on the object's prototype. If the property or method is still not found, JavaScript continues the search up the prototype chain until it reaches the top level, which is typically the Object.prototype object.
In other words, the prototype is a way to implement inheritance in JavaScript, allowing objects to inherit properties and methods from other objects. This can help simplify code and make it more efficient, by allowing objects to share common functionality without having to recreate it for each object. To create a new object with a specific prototype, you can use the Object.create() method, passing in the desired prototype object as an argument.
What is event bubbling in JavaScript?
Event bubbling is a mechanism in JavaScript where events propagate from the innermost to the outermost elements in the HTML DOM. When an event is triggered on an element, it is first handled by that element's event listener. If the event listener does not stop the event from propagating, the event then bubbles up to the element's parent, and so on until it reaches the top-level element.
Event bubbling can be useful for handling events on multiple elements with a common ancestor. However, it can also cause unintended consequences if not handled properly. To stop event bubbling, you can call the event.stopPropagation() method within the event listener.
What is the difference between let and const in JavaScript?
The main difference between let and const in JavaScript is in their mutability. Variables declared with let can be reassigned a new value, while variables declared with const cannot be reassigned. Additionally, variables declared with const must be initialized with a value at the time of declaration, while variables declared with let can be initialized later. Both let and const are block-scoped, meaning they are only accessible within the block in which they are declared.
What is the difference between synchronous and asynchronous programming in JavaScript?
Synchronous programming in JavaScript refers to a style of programming where each statement is executed in turn, and the program waits for each statement to complete before moving on to the next one. Asynchronous programming, on the other hand, refers to a style of programming where operations can run in the background while the program continues to execute. Asynchronous programming is often used for I/O operations, such as reading or writing to a file, or making a network request, where the operation can take a long time to complete.
What is the difference between a for loop and a forEach loop in JavaScript?
A for loop in JavaScript is a traditional loop structure that iterates over a set of values using a counter variable. A forEach loop, on the other hand, is a method on the Array object that allows you to iterate over each element in an array and perform an action on each element. The main difference between the two is that a for loop is more flexible and can be used for iterating over any set of values, while a forEach loop is specifically designed for iterating over arrays. Additionally, a forEach loop cannot be interrupted or stopped in the middle, while a for loop can be exited using a break statement.
What is the difference between a regular function and an arrow function in JavaScript?
The main difference between a regular function and an arrow function in JavaScript is in their syntax and the way they handle the this keyword. Arrow functions have a shorter syntax than regular functions, and they do not bind their own this keyword. Instead, the this keyword in an arrow function refers to the value of this in the context in which the arrow function was defined. This can be useful for avoiding the common "this" pitfalls that can arise with regular functions.
What is the difference between a callback function and a promise in JavaScript?
A callback function is a function that is passed as an argument to another function, and is typically used for handling asynchronous operations in JavaScript. A promise, on the other hand, is an object that represents the eventual completion of an asynchronous operation, and provides a more structured and flexible way of handling asynchronous code. While both callback functions and promises can be used for handling asynchronous operations in JavaScript, promises are generally considered to be more flexible and easier to reason about, especially when dealing with complex asynchronous workflows.
What are the four principles of object-oriented programming?
The four principles of object-oriented programming are inheritance, encapsulation, abstraction, and polymorphism. Inheritance allows objects to inherit properties and methods from other objects. Encapsulation is the practice of keeping an object's internal state and behavior hidden from the outside world. Abstraction is the practice of simplifying complex systems by breaking them down into smaller, more manageable parts. Polymorphism allows objects to take on multiple forms or behaviors depending on the context in which they are used.
What is the difference between the innerHTML and textContent properties in JavaScript?
The innerHTML property in JavaScript allows for the manipulation of the HTML content inside an element, including tags and attributes. The textContent property, on the other hand, only returns the text content of an element, without any HTML tags or attributes. It is generally recommended to use textContent when dealing with text-only content, and innerHTML when dealing with HTML content that may contain tags and attributes.
What is the purpose of the "use strict" directive in JavaScript?
The "use strict" directive in JavaScript enables strict mode, which is a set of rules that must be followed in order to write secure and efficient JavaScript code. In strict mode, certain JavaScript features that are considered error-prone or dangerous are disabled, and stricter rules are enforced for variable declaration, function invocation, and other aspects of the language. Using strict mode can help to prevent common coding mistakes and improve the overall quality of JavaScript code.
What is the difference between the spread operator (...) and the rest operator (...) in JavaScript?
The spread operator (...) in JavaScript is used to expand an iterable (such as an array or a string) into individual elements. It is often used to pass the contents of an array or an object as arguments to a function or to concatenate arrays. The rest operator (...), on the other hand, is used to capture a variable number of arguments passed to a function into an array. It is often used in function declarations to allow for a variable number of arguments to be passed to the function.
What is the purpose of the "this" keyword in JavaScript?
The "this" keyword refers to the current execution context, which is typically the object that the function is a method of. It is often used to access or manipulate properties of the current object within a method, or to bind a function to a specific object. The behavior of the "this" keyword can be affected by the way in which a function is called, such as with the "call" or "apply" methods, or by using arrow functions, which bind the "this" keyword to the lexical scope of the function.
What is the purpose of the "use strict" directive in JavaScript?
The "use strict" directive in JavaScript is a feature introduced in ECMAScript 5 that enables strict mode, which is a stricter version of JavaScript that eliminates some silent errors and enforces stricter coding standards. In strict mode, certain actions that were previously ignored or silently failed will now throw errors, making it easier to write more reliable and secure code. The "use strict" directive is typically placed at the beginning of a JavaScript file or function to enable strict mode for that scope.
What is a generator function in JavaScript?
A generator function in JavaScript is a special type of function that can be paused and resumed, allowing for the generation of a sequence of values on demand. Generator functions are declared using the "function*" syntax and use the "yield" keyword to produce a value and pause execution. They can also receive input values when resumed using the "next" method.
How do you define a function in JavaScript?
You can define a function in JavaScript using the function keyword, like this:
function myFunction(parameter1, parameter2) {
// Code to perform the task
return result;
}
What is an event in JavaScript?
An event in JavaScript is an action that occurs on a web page, such as a mouse click or a key press.
What is the DOM in JavaScript?
The DOM (Document Object Model) in JavaScript is a hierarchical representation of a web page's HTML structure, which can be manipulated using JavaScript.
How do you access an element in the DOM using JavaScript?
You can access an element in the DOM using JavaScript using methods such as document.getElementById(), document.querySelector(), or document.querySelectorAll().
What is AJAX in JavaScript?
AJAX (Asynchronous JavaScript and XML) in JavaScript is a technique used to update parts of a web page without reloading the entire page.
What is a constructor function in JavaScript?
A constructor function in JavaScript is a function that is used to create and initialize an object.